Operators & Decision ConstructsJ8 Home « Operators & Decision Constructs
Lets take a look at the points outlined at Oracle Java SE 8 Programmer I for this part of the certification.
- Using Operators & Decision Constructs
- Use Java operators; use parentheses to override operator precedence.
- Test equality between Strings and other objects using == and equals ().
- Create if and if/else and ternary constructs.
- Use a switch statement.
The table below breaks the above list down into topics with a link to the topic in question.
topic | Link |
---|---|
Use Java operators; use parentheses to override operator precedence. | Java Operators |
Test equality between Strings and other objects using == and equals (). | String Equality |
Create if and if/else and ternary constructs. | The if ConstructThe Ternary ?: Operator |
Use a switch statement. | The switch Construct |
Java Operators Top
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In this section we cover the arithmetic operators with examples of precedence with and without the use of parentheses. A complete guide to operators are discussed in the Operators lesson and bitwise operators are discussed in the Bitwise Operators lesson.
Arithmetic Operators
We are familiar with most of the arithmetic operators in the table below from everyday life and they generally work in the same way in Java. The arithmetic operators work with all the Java numeric primitive
types and can also be applied to the char
primitive type.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
+ | Addition | int b = 5; b = b + 5; | 10 | |
- | Subtraction | int b = 5; b = b - 5; | 0 | |
/ | Division | int b = 7, b = b / 22; | 3 | When used with an integer type, any remainder will be truncated. |
* | Multiplication | int b = 5; b = b * 5; | 25 | |
% | Modulus | int b = 5; b = b % 2; | 1 | Holds the remainder value of a division. |
++ | Increment | int b = 5; b++; | 6 | See below. |
-- | Decrement | int b = 5; b--; | 4 | See below. |
Increment And Decrement Operators
Both these operators can be prepended (prefix) or appended (postfix) to the operand they are used with. When applied to an operand as part of a singular expression it makes no difference whether the increment and decrement operators are applied as a prefix or postfix. With larger expressions however, when an increment or decrement operator precedes its operand, Java will perform the increment or decrement operation prior to obtaining the operand's value for use by the rest of the expression. When an increment or decrement operator follows its operand, Java will perform the increment or decrement operation after obtaining the operand's value for use by the rest of the expression. Lets look at some code to illustrate how this works:
package info.java8;
/*
Increment And Decrement Operators
*/
public class IncDec {
public static void main (String[] args) {
byte a = 5;
byte b = 5;
// Singular Expressions
a++;
++b;
System.out.println("a = " + a);
System.out.println("b = " + b);
// Larger Expressions
a = 1;
b = a++;
System.out.println("b using a postfix = " + b);
a = 1;
b = ++a;
System.out.println("b using a prefix = " + b);
}
}
Running the IncDec
class produces the following output:
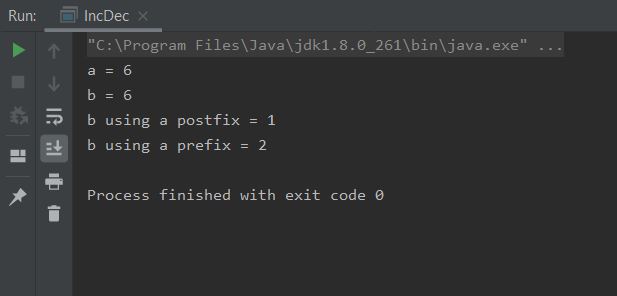
As you can see with the last two lines of output, the postfix increment is applied after the expression is evaluated, whereas the prefix increment is applied before.
Operator Precedence
We will finish our discussion on operators with a table showing the order of precedence that is invoked when Java interprets operator symbols. When using multiple operators in an expression it is always best practice to use parentheses to explicitly state the order of precedence for clarity and readability. The table lists order of precedence from highest to lowest.
Precedence | Operators | Operation | Associativity |
---|---|---|---|
1 | () | method call | left |
[ ] | array index | ||
. | method access | ||
2 | + | unary plus | right |
- | unary minus | ||
++ | prefix/postfix increment | ||
-- | prefix/postfix decrement | ||
! | boolean logical NOT | ||
~ | bitwise NOT | ||
(type) | type cast | ||
new | object creation | ||
3 | / | division | left |
* | mutiplication | ||
% | modulus | ||
4 | + | addition or string concatenation | left |
- | subtraction | ||
5 | << | bitwise left shift | left |
>> | bitwise right shift | ||
>>> | bitwise signed right shift | ||
6 | / | less than | left |
<= | less than or equal to | ||
> | greater than | ||
>= | greater than or equal to | ||
instanceof | reference test | ||
7 | == | equal to | left |
!= | not equal to | ||
8 | & | boolean logical AND or bitwise AND | left |
9 | ^ | boolean logical XOR or bitwise XOR | left |
10 | | | boolean logical OR or bitwise OR | left |
11 | && | short-circuit boolean logical AND | left |
12 | || | short-circuit boolean logical OR | left |
13 | ? : | conditional | right |
14 | = | assignment | right |
+= | shorthand addition | ||
-= | shorthand subtraction | ||
/= | shorthand division | ||
*= | shorthand mutiplication | ||
%= | shorthand modulus | ||
&= | shorthand boolean logical AND or bitwise AND | ||
|= | shorthand boolean logical OR or bitwise OR | ||
^= | shorthand boolean logical XOR or bitwise XOR | ||
<<= | shorthand bitwise left shift | ||
>>= | shorthand bitwise right shift | ||
>>>= | shorthand bitwise signed right shift |
String Equality Top
The equals()
method will return true
if the invoking string has the the same character sequence as the argument string. This method is a valid override of the Object
superclass equals()
method. The following code and screenshot shows examples of using the String
class equals()
method:
package info.java8;
/*
The equals() method
*/
public class TestEquals {
public static void main(String[] args) {
String str1 = new String("aaa");
String str2 = "aaa";
String str3 = "AAA";
System.out.println(str1 == str2);
System.out.println(str1 == str3);
System.out.println(str2 == str3);
System.out.println(str1.equals(str2));
System.out.println(str1.equals(str3));
System.out.println(str2.equals(str3));
}
}
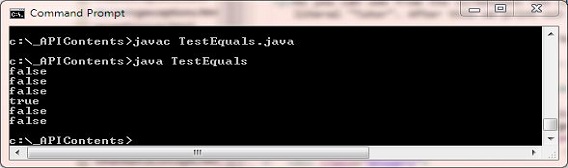
The screenshot above shows the results of running the TestEquals
class. When we check for object equality using the ==
relational operator all tests return false
as no references refer
to the same object. When we use the equals()
method then the objects referenced by str1
and str2
return true
as these objects do indeed have the same character sequence.
Incidentally there is also an equalsIgnoreCase()
method in the String
class which would return true for the last 3 tests. I'll leave it for you to investigate this method :)
The if
ConstructTop
Create a conditional expression using one of the relational operators available in Java to test one operand against another or test the result of a logical operation. We can execute one set of statements if the boolean result of the expression evaluates to true
and another if the expression evaluates to false
.
The relational and logical operators are discussed in more detail in the Operators lesson.
The following table shows the different forms of the if
construct that can be used. We are using blocks of code to wrap statements which is optional, but makes code more readable and is good practice and will be used here.
Construct | Description |
---|---|
Simple if | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true . |
if....else | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true ,otherwise execute statements in statement N . |
Nested if | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true Execute statements in statement2 if condition2 expression evaluates to
true ,otherwise execute statements in statement3 if condition2 expression evaluates to false , otherwise execute statements in statement N if condition expression evaluates to false . |
Multiple if....else if | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true
Execute statements in statement2 if condition2 expression evaluates to true , Execute statements in statement3 if condition3
expression evaluates to true etc..., otherwise execute statements in statement N . |
if
Rules and Examples
There are a few rules to remember when coding the if
statement:
- The expression we check against, in the parentheses, must evaluate to a
boolean
type:true
orfalse
. - One optional
else
statement can be coded as part of theif
construct and must come after anyelse if
statements. - Multiple
else if
statements can be coded after the initialif
and before the optionalelse
statement (if coded). - As soon as we hit a
true
condition any otherelse if
statements will not be tested.
Following are some examples showing if
statement usage:
/*
Some code showing if statement usage
*/
boolean b = false;
int i = 2;
// Simple if
if (b == false) {
System.out.println("This is executed");
}
if (i = 2) { // Will not compile, does not evaluate to boolean
System.out.println(" ");
}
// if else
if (b = false) { // Assignment not evaluation
System.out.println("This is NOT executed");
} else {
System.out.println("This is executed");
}
// Multiple if else
if (i == 0) {
System.out.println("This is NOT executed");
} else if (i == 1) {
System.out.println("This is NOT executed");
} else if (i == 2) {
System.out.println("This is executed");
} else {
System.out.println("This is NOT executed");
}
if (i == 0) {
System.out.println("This is NOT executed");
} else (i == 1) { // Will not compile, else must come last
System.out.println(" ");
} else if {
System.out.println(" ");
}
The Ternary ?:
Operator Top
The tenary (takes three operands) ?:
operator can be used to replace an if....else
construct of the following form:
Construct | Description |
---|---|
if....else | |
if (condition) { | Assign result of expression1 to
var if condition evaluates to true ,otherwise assign result of expression2 to var. |
? : Operator | |
expression1 ? expression2 : expression3 |
If expression1 returns true evaluate expression2 ,
otherwise evaluate expression3 , |
Lets examine some code to see how the ?:
operator works:
package info.java8;
/*
The Ternary Operator
*/
public class TernaryOperator {
public static void main (String[] args) {
int int1 = 1234, int2 = 5678, maxInt;
if (int1 > int2) {
maxInt = int1;
}
else {
maxInt = int2;
}
System.out.println("if...else maxInt: " + maxInt);
maxInt = (int1 > int2) ? int1 : int2;
System.out.println("ternary ?: maxInt: " + maxInt);
}
}
Running the TernaryOperator
class produces the following output:
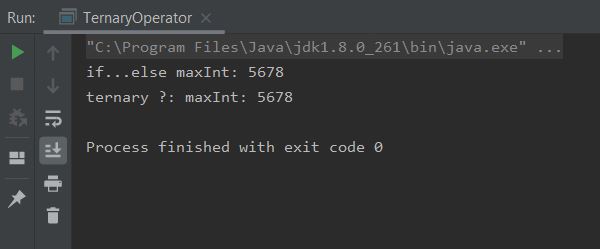
You should see 2 lines of output with the same values showing how we can replace this form of the if....else
construct with the ternary ?:
operator.
The if
ConstructTop
The Ternary ?:
Operator Top
The switch
ConstructTop
The switch
ConstructTop
The switch
Construct can be used instead of the multiple if....else if
construct when we are doing a multiway test on the same value. When this scenario arises the switch
Construct
is often a more efficent and elegant way to write our code
The switch
Construct is where we put the expression we are going to evaluate. Each case
constant is evaluated against the switch
expression and the statements within
the case
are processed on a match. We have the option to break
from the switch
after this if required. We can also code an optional default
statement,
which can act as a kind of catch all and is normally placed, at the end, after the case
statements.
Construct | Description |
---|---|
switch | |
switch (expression) { |
expression can be of type char , byte , short or int .constant1 must be a literal of a type compatible with the
switch expression .Execute statements1 on match.Optional break from switch Construct.
constant2 must be a literal of a type compatible with the switch expression .Execute statements2 on match.Optional break from switch Construct.constantN must be a literal of a type compatible with the switch expression .Execute statementsN on match.Optional break from switch Construct. Optional default statement (catch all).Execute defaultStatements .Optional break from switch Construct. |
nested switch | |
switch (expression1) { |
This is perfectly legal. |
switch
Rules and Examples
There are a few rules to remember when coding the switch
statement:
- The
switch
expression we check against, in the parentheses, must evaluate to achar
type, or thebyte
,short
orint
signed numeric integer
types or to anenum
. - When not using an
enum
in ourswitch
Construct only types that can be implicitly cast to anint
are allowed. - The
switch
Construct can only check for equality (==) and so the otherrelational operators
won't work withswitch
. - The
case
constant must evaluate to the same type as theswitch
expression. - The
case
constant is a compile time constant and because it has to be resolved at compile time, any constant or final variables must have been assigned a value. - The value of the
case
constants must be different. - When a
case
constant evaluates totrue
the associated code block and any code blocks following are executed, unless abreak
statement is encountered. - The
default
statement can be placed anywhere within theswitch
Construct and when executed, the associated code block and any code blocks following are executed, unless abreak
statement is encountered.
Following are some examples showing switch
statement usage:
/*
Some code showing switch statement usage
*/
final int i1 = 2;
final int i2;
byte b = 25;
String s = "java";
// switch
switch (i1) {
case 1:
System.out.println("This is not executed");
case 2:
System.out.println("This is executed");
case 3:
System.out.println("This is executed");
}
switch (i2) { // Not a compile time constant so won't compile
case 1:
System.out.println(" ");
case 2:
System.out.println(" ");
case 3:
System.out.println(" ");
}
switch (i1) {
case 1:
System.out.println(" ");
case 2:
System.out.println(" ");
case 2: // Same case value so won't compile
System.out.println(" ");
}
switch (b) {
case 25:
System.out.println(" ");
case 128: // Loss of precision so won't compile
System.out.println(" ");
}
switch (s) { // Incompatible switch type of String so won't compile
case "java2":
System.out.println(" ");
case "java":
System.out.println(" ");
case "java":
System.out.println(" ");
}
// switch break
int i3 = 2;
switch (i3) {
case 1:
System.out.println("This is not executed");
case 2:
System.out.println("This is executed");
break;
case 3:
System.out.println("This is not executed");
}
// switch default
int i4 = 2;
switch (i4) {
case 0:
System.out.println("This is not executed");
case 1:
System.out.println("This is not executed");
default:
System.out.println("This is executed");
}
switch (i4) {
case 0:
System.out.println("This is not executed");
default:
System.out.println("This is executed");
case 1:
System.out.println("This is executed");
}
Related Java Tutorials
Fundamentals - Conditional Statements
Fundamentals - Loop Statements
Fundamentals - Primitive Variables
Fundamentals - Operators
Flow Control - Using Assertions
Flow Control - Exception Overview
Flow Control - Exception Handling
Flow Control - Declaring Exceptions