Primitives - boolean
& char
data typesJ8 Home « Primitives - boolean
& char
data types
In this lesson we take a much closer look at the boolean
and char
primitive variable data types available in Java.
The following table gives information on the boolean
and char
primitive variable data types.
Type | Meaning | Bit Width | Range |
---|---|---|---|
boolean | |||
boolean | true/false values | JVM specific | true or false |
char | |||
char | Character | 16 | 0 to 65535 - ( |
The boolean
Type Top
The boolean
type represents true and false values which are defined in Java using the true
and false
reserved words. The
boolean type can be initialized using these values, control the flow of an if
Construct and is returned from a relational operator. Lets write some
code to see this in action. You can create the following class in your IDE and cut and paste the code into it.
package info.java8;
/*
Initialising and using booleans primitives
*/
public class BooleanType {
public static void main (String[] args) {
boolean a; // Not initialized
boolean b = true; // Initialized
a = false; // Initialized after creation
System.out.println("a is " + a);
System.out.println("b is " + b);
// a is false so will not execute
if (a) { System.out.println("a is executed"); }
// b is true so will execute
if (b) { System.out.println("b is executed"); }
// Relational operator output is a boolean
System.out.println("1 > 2? is " + (1 > 2));
System.out.println("2 > 1? is " + (2 > 1));
}
}
Running the BooleanType
class produces the following output:
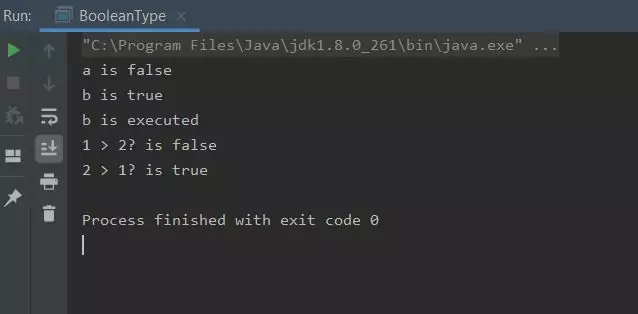
BooleanType
class.You should see 5 lines of output as shown in the screenshot. The first two output lines display the values we set for our variables. The interesting thing to note here is the use of the +
symbol, which is used in Java to concatenate strings aside from its mathematical uses. The third output line shows the results of the second if
Construct we coded. We will go into this statement in the if
Construct lesson for now it's enough to know that statements contained within the curly bracers will execute when the expression being tested is true. The last two outputs test relational operators and output a boolean dependant upon the result.
The char
Type Top
Most computer languages use the standard 8-bit ASCII character set which has a range of 0
to 127
to represent characters. in Java the
char
primitive type is an unsigned type in the range 0
to 65,536
and uses Unicode. Unicode defines a
character set that can represent any character found in any human language. The ASCII character set is a subset of Unicode and as such
ASCII characters are still valid in Java. You can create the following class in your IDE and cut and paste the code into it.
package info.java8;
/*
Initialising and using char primitives
*/
public class CharType {
public static void main (String[] args) {
// Initialized using value in single quotes
char charA = 'P';
System.out.println("charA is " + charA);
// We can subtract from the char type
charA -= 12;
System.out.println("charA is " + charA);
// We can assign an integer to the char type
charA = 45;
System.out.println("charA is " + charA);
}
}
Running the CharType
class produces the following output:
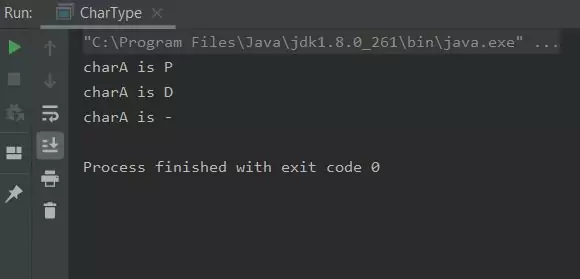
CharType
class.You should see 3 lines of output as shown in the screenshot. The first output line displays the value 'P' that we assigned to our variable. The second output
line shows the result of subtracting 12 from our initial value. The third output line shows the result of assigning integer 45 to our variable. Because the
char
primitive type is an unsigned 16-bit type we can perform mathematics on this type as if it was an integer, remember though the range is
0
to 65,536
.
Related Quiz
Fundamentals Quiz 3 - Primitives - boolean
& char
data types Quiz
Lesson 4 Complete
In this lesson we looked at the boolean
and char
primitive variable data types available in Java.
What's Next?
In the next lesson we look at the numeric primitive variable data types available in Java.