if
ConstructJ8 Home « if
Construct
In this lesson we take our first look at the conditional statements available in Java. Conditional statements allow us to evaluate an expression and execute some code dependant upon the outcome. There are two conditional statements we can use with Java, the if
Construct covered here and the switch
Construct which we will cover in the next lesson. The choice of which to use really depends on the expression being evaluated. There is also the ternary ?:
special operator which acts like an if
Construct and which we will cover after looking in detail at the if
Construct.
The if
Construct Top
Create a conditional expression using one of the relational operators available in Java to test one operand against another or test the result of a logical operation. We can execute one set of statements if the boolean result of the expression evaluates to true
and another if the expression evaluates to false
.
The relational and logical operators are discussed in more detail in the Operators lesson.
The following table shows the different forms of the if
construct that can be used. We are using blocks of code to wrap statements which is optional, but good practice and will be used here.
Construct | Description |
---|---|
Simple if | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true . |
if....else | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true ,otherwise execute statements in statement N . |
Nested if | |
if (condition) { | Execute statements in
statement1 if condition expression evaluates to true ,Execute statements in statement2 if condition2 expression evaluates to
true ,otherwise execute statements in statement3 if condition2 expression evaluates to false , otherwise execute statements in statement N if condition expression evaluates to false . |
Multiple if....else if | |
if (condition) { | Execute statements in statement1 if condition expression evaluates to true ,
Execute statements in statement2 if condition2 expression evaluates to true , Execute statements in statement3 if condition3
expression evaluates to true etc..., otherwise execute statements in statement N . |
We will go through the different forms of the if
construct one at a time, using some code to make understanding of the above table easier.
Simple if
Construct Top
In its simplest form, the if
Construct will execute the statement or block of code following the conditional expression when the expression evaluates to true
, otherwise execution will continue
after the statement or block of code. Lets look at some code to illustrate how this works:
package info.java8;
/*
Simple If Construct
*/
public class SimpleIf {
public static void main (String[] args) {
if (args[0].length() > 0)
System.out.println("Command input was: " + args[0]);
if (args[0].length() > 0) {
System.out.println("Command input was: " + args[0]);
}
}
}
Running the SimpleIf
class after adding 24 to Program arguments pane of the run configuration, produces the following output:
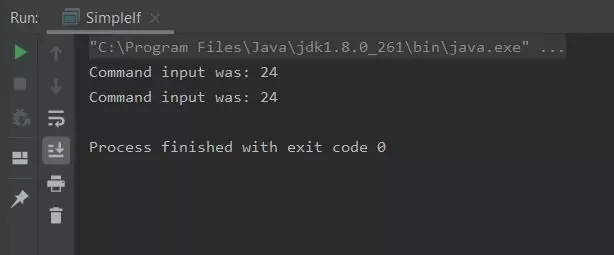
SimpleIf
class.If you have entered something when running the interpreter you should see 2 lines of output as in the screenshot above. We will talk about what args.length
means in the Arrays lesson
but for now it's enough to know that we are checking the args[]
string array for input. The first if
construct above doesn't use a block and therefore is limited to a single statement. The second
if
construct above uses a block and therefore can have multiple statements. We are just showing the first if
construct for information purposes. We recommend always using a block
when using any conditional as in the second example as it makes code easier to read, easier to maintain and is not limited to single statements.
if....else
Construct Top
In this form, the if
Construct will execute the statement or block of code following the conditional expression when the expression evaluates to true
, otherwise will execute the statement
or block of code following the else
when the expression evaluates to false
. Lets look at some code to see this in action:
package info.java8;
/*
if...else Construct
*/
public class IfElse {
public static void main (String[] args) {
if (args.length > 0)
System.out.println("Command input was: " + args[0]);
else System.out.println("No command input entered");
if (args.length > 0) {
System.out.println("Command input was: " + args[0]);
} else {
System.out.println("No command input entered");
}
}
}
Running the IfElse
class after adding 48 to Program arguments pane of the run configuration, produces the following output:
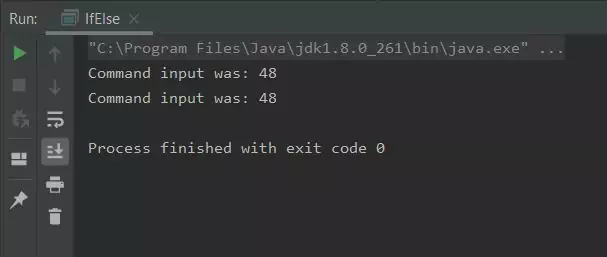
IfElse
class.If you have run the program twice with and without a parameter you should see 4 lines of output similar to the screenshot above. The first if....else
construct above doesn't use a block and therefore is
limited to a single statement for the if
and else
. The second if....else
construct above uses a block and therefore can have multiple statements for the if
and else
.
We are just showing the first if...else
construct for information purposes. We recommend always using a block
when using any conditional as in the second example as it makes code easier to read,
easier to maintain and is not limited to single statements. We will not be using the first simpler form in any more examples but you can now see how to code the construct this way.
Nested if
Construct Top
A nested if
Construct is the target of another if
or else
statement. With nested if
s the else
statement always refers to the nearest if
Construct
that is within the same block of code as the else
and is not already associated with an else
. Lets look at some code:
package info.java8;
/*
Nested if Construct
*/
public class NestedIf {
public static void main (String[] args) {
if (args.length > 0) {
int num = Integer.parseInt(args[0]);
if (num > 50) {
if (num > 75) {
System.out.println("Input over 75: " + args[0]);
} else {
System.out.println("Input 51 to 75: " + args[0]);
}
} else {
System.out.println("Input under 51: " + args[0]);
}
} else {
System.out.println("No command input was entered");
}
}
}
Running the NestedIf
class after adding 51 to Program arguments pane of the run configuration, produces the following output:
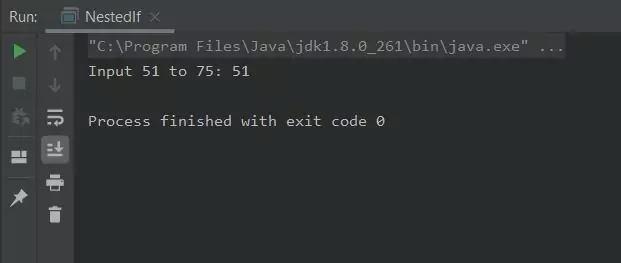
NestedIf
class.The message displayed depends which branch of the nested if
construct we branch to. As you can see we can go in as many levels as we like but even with all that lovely indentation coding multiple nested if
constructs can get complex and confusing although the example above is a bit contrived :).
Multiple if....else
Construct Top
The nested if
Construct can get confusing and out of hand and generally in programming if something looks too complicated there is an easier way to do it.
Lets rewrite part of the nested if
construct above using the multiple if....else if
construct:
package info.java8;
/*
Multiple if....else Construct
*/
public class MultipleIfElse {
public static void main (String[] args) {
if (args.length > 0) {
int num = Integer.parseInt(args[0]);
if (num > 75) {
System.out.println("Input over 75: " + args[0]);
} else if (num > 50) {
System.out.println("Input 51 to 75: " + args[0]);
} else {
System.out.println("Input under 51: " + args[0]);
}
} else {
System.out.println("No input was entered");
}
}
}
Running the MultipleIfElse
class after adding 50 to Program arguments pane of the run configuration, produces the following output:
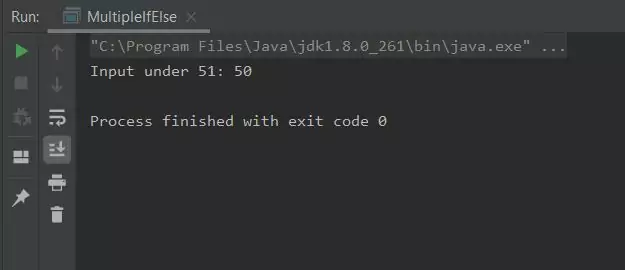
MultipleIfElse
class.The message displayed depends which branch of the multiple if....else if
construct we branch to. This example is easier to follow than the multiple nested if
of the previous example and also illustrates how we can mix the various if
constructs.
The Ternary ?:
Operator Top
The tenary (takes three operands) ?:
operator can be used to replace an if....else
construct of the following form:
Construct | Description |
---|---|
if....else | |
if (condition) { | Assign result of expression1 to
var if condition evaluates to true ,otherwise assign result of expression2 to var. |
? : Operator | |
expression1 ? expression2 : expression3 |
If expression1 returns true evaluate expression2 ,
otherwise evaluate expression3 , |
Lets examine some code to see how the ?:
operator works:
package info.java8;
/*
The Ternary Operator
*/
public class TernaryOperator {
public static void main (String[] args) {
int int1 = 1234, int2 = 5678, maxInt;
if (int1 > int2) {
maxInt = int1;
}
else {
maxInt = int2;
}
System.out.println("if...else maxInt: " + maxInt);
maxInt = (int1 > int2) ? int1 : int2;
System.out.println("ternary ?: maxInt: " + maxInt);
}
}
Running the TernaryOperator
class produces the following output:
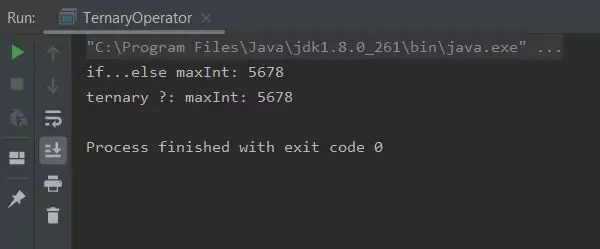
TernaryOperator
class.You should see 2 lines of output with the same values showing how we can replace this form of the if....else
construct with the ternary ?:
operator.
Related Quiz
Fundamentals Quiz 11 - if
Construct Quiz
Lesson 12 Complete
In this lesson we investigated the if
and ternary conditional statements available for use in Java.
What's Next?
In the next lesson we look at the switch
conditional statement.