for
ConstructJ8 Home « for
Construct
In this lesson we take our first look at the loop statements available in Java. Loop statements allow us to iterate over some code multiple times. There are two loop statements in Java, the for
construct covered here and the while
Construct which we will cover in the next lesson.
The for
ConstructTop
The for
Construct will loop through a section of code a set number of times. The for
Construct is very versatile and has two different variations known commonly as the for loop and the
enhanced for loop.
The for loop contains three parts. In the first part we initialize a counter variable with a value, this only happens on initial entry to the for loop. The second part is a condition
which tests the variable value at the start of each loop and if the condition is no longer true
the for loop is exited. The final part of the for
Construct is an expression to be
evaluated at the end of each iteration of the loop. This normally takes the form of a counter that is decremented or incremented.
The enchanced for loop was introduced in Java and implements a for-each style loop that iterates through a collection of objects in a sequential order from start to finish.
The following table shows the different forms of the for
construct that can be used. We are using blocks of code to wrap statements which is optional when using a single statement, but good practice and will be used here.
Construct | Description |
---|---|
for loop | |
for ([initialization]; [condition]; [iteration]) { | The initialization , condition , iteration and
statement body components of a for Construct are all optional.The following will create an infinite for loop :for (;;) {} // Infinite loopThe following will create a for loop with no body:for (int i=1; i<5; i++); // No bodyThe initialization component is generally an assignment statement that
sets the initial value of a control variable used to control iteration of the loop.The condition component is a conditional expression that is always tested againt the control variable
for true before each iteration of the loop. So if this is false to begin with then any statement body component will never be executed.The iteration component
is an expression that determines the amount the control variable is changed for each loop iteration.The statement body is executed each time the condition component
returns true when tested against the control variable. |
enhanced for loop | |
for (declaration : expression) { | The declaration component declares a variable of a type compatible with the collection to be accessed which will hold a value the same as the current element within the collection.The expression component can be the result of a method call or an expression that evalutes to a collection type.The statement body is executed each time an element of the collection is iterated over. |
We will go through the different forms of the for
construct one at a time, using some code to make understanding of the above table easier.
The for
Construct and break
Top
The for
construct will loop through a section of code a set number of times dependant upon the components set for the loop and their values. Lets look at some code to illustrate how this works:
package info.java8;
/*
for Construct
*/
public class UsingFor {
public static void main (String[] args) {
for (int i=1; i<13; i++) {
System.out.println("The square of " + i + " = " + i*i);
}
for (int i=1; i<13; i++) {
// Using a break to exit loop
if (i == 6) { break; }
System.out.println("The square of " + i + " = " + i*i);
}
}
}
Running the UsingFor
class produces the following output:
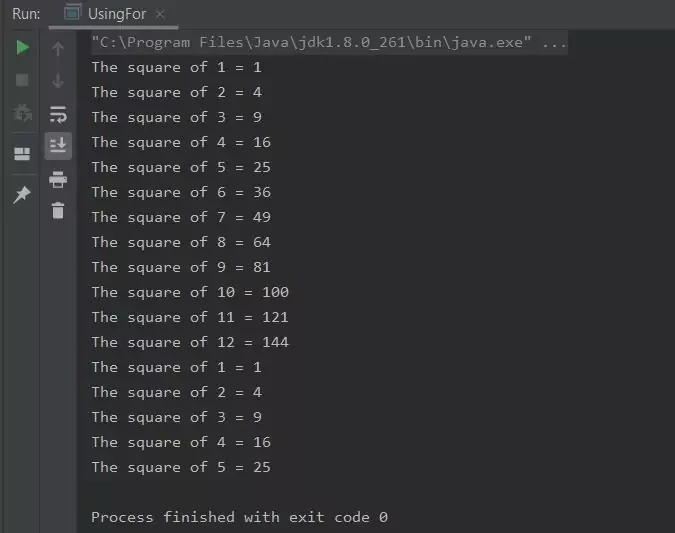
UsingFor
class.In the first loop what we are doing here is using the control variable (i) and squaring it for the output while it is less than 13. After each iteration we increment the control variable by 1. In the second loop we break
from the loop when the control variable (i) reaches 6. The break
statement forces an exit from the loop immediately. Did you notice also that we use the variable i
twice with no problem. This is
because this variable is created and exists only as long as the scope it was created in, in this case the for
construct. Take a look at the Method Scope lesson for a refresher on
local variable scope.
Using A Nested for
Construct and break
Top
We can also nest the for
construct within another for
construct (or any other construct for that matter). Lets look at examples of this:
package info.java8;
/*
Nested for Construct
*/
public class NestedFor {
public static void main (String[] args) {
for (int i=1; i<11; i++) {
System.out.println("\nThe " + i + " times table: ");
for (int j=1; j<11; j++) {
System.out.print(j + "*" + i + "=" + j*i + ": ");
}
}
for (int i=1; i<11; i++) {
System.out.println("\nThe " + i + " times table: ");
for (int j=1; j<11; j++) {
// Using a break to exit inner loop
if (j == 4) { break; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
}
}
}
}
Running the NestedFor
class produces the following output:
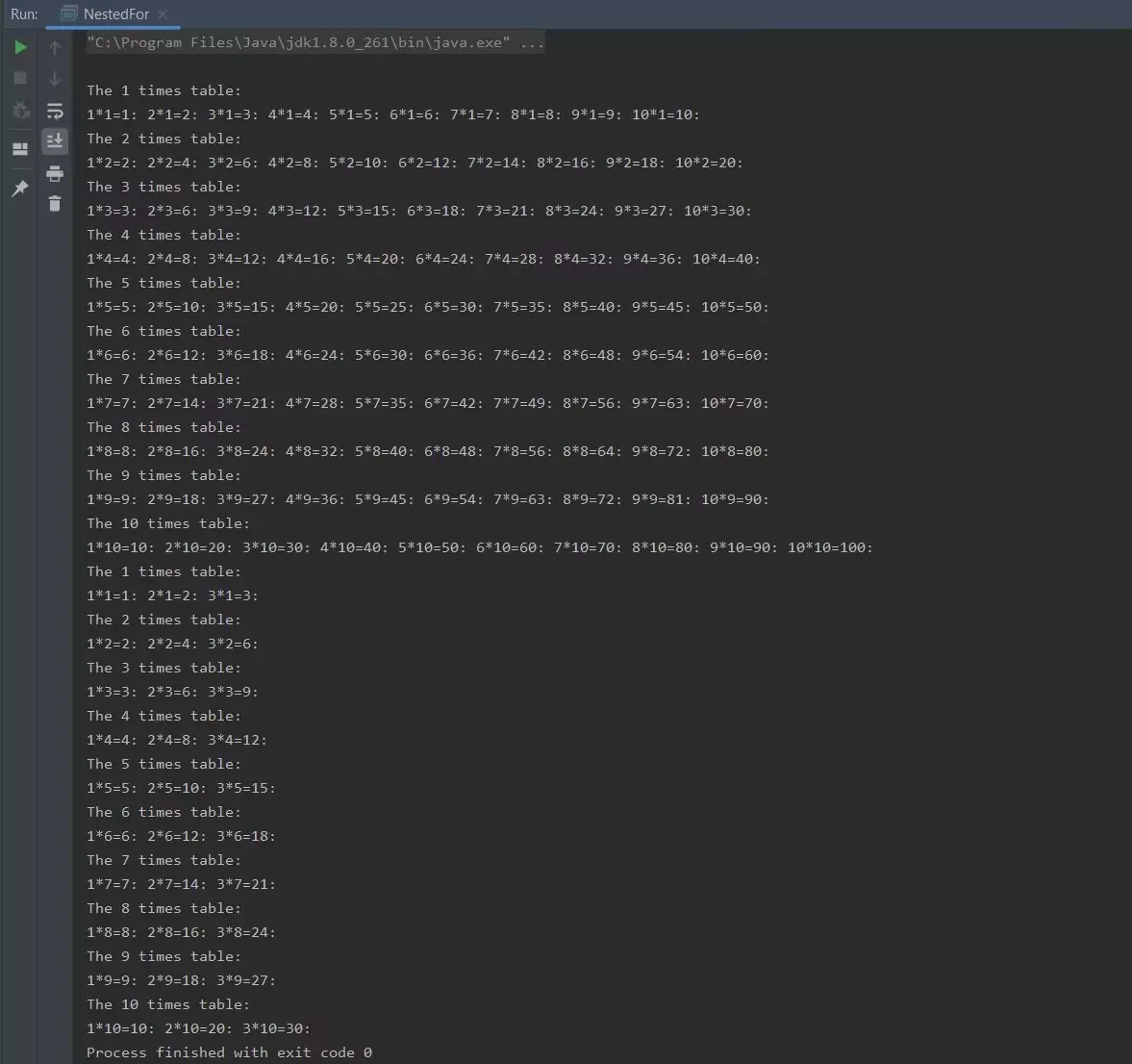
NestedFor
class.What we are doing here is running a loop within a loop to print off times tables up to 10. So the inner for
loop runs 10 times for each outer for
loop iteration. In the second example we break from the
inner loop during the fourth iteration. This is just to show that using the break
statement forces an exit from the loop you are in not any outer loops.
Using the continue
StatementTop
We can also use the continue
statement within any loop construct. For a for
construct, the continue
statement will stop this iteration of the loop and continue at the start of the next iteration. Lets look at an example of this:
package info.java8;
/*
Using continue in a for Construct
*/
public class UsingForContinue {
public static void main (String[] args) {
for (int i=1; i<13; i++) {
// Using a continue to force an iteration
if ((i%2) == 0) { continue; }
System.out.println("The square of " + i + " = " + i*i);
}
}
}
Running the UsingForContinue
class produces the following output:
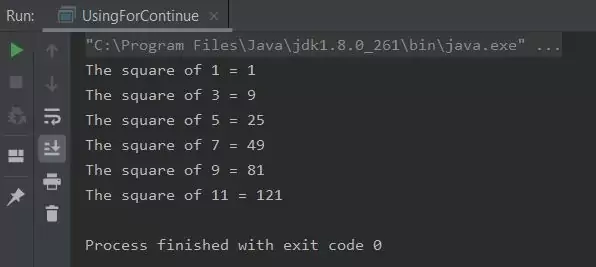
UsingForContinue
class.In this variation we are checking to see if the control variable (i) leaves no remainder. If this is the case we are forcing a continue
to the next iteration. For this example it means we are only printing out odd
squares within the specified range.
Using the break
to a labelled StatementTop
We saw how the break
statement worked with the for
construct in earlier examples. There is a variation where we can break
to a label
statement. Breaking to a labelled
statement causes program execution to resume after the labelled block. Lets look at examples of this:
package info.java8;
/*
Using break to a label in a for Construct
*/
public class UsingLabelBreak {
public static void main (String[] args) {
label1:
for (int i=1; i<11; i++) {
System.out.println("\nThe " + i + " times table: ");
for (int j=1; j<11; j++) {
// Using a break to label to exit loop
if (j == 4) { break label1; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
}
}
System.out.println("\n\n****");
for (int i=1; i<11; i++) {
System.out.println("\nThe " + i + " times table: ");
label2:
for (int j=1; j<11; j++) {
// Using a break to label to exit loop
if (j == 4) { break label2; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
}
}
}
}
Running the UsingLabelBreak
class produces the following output:
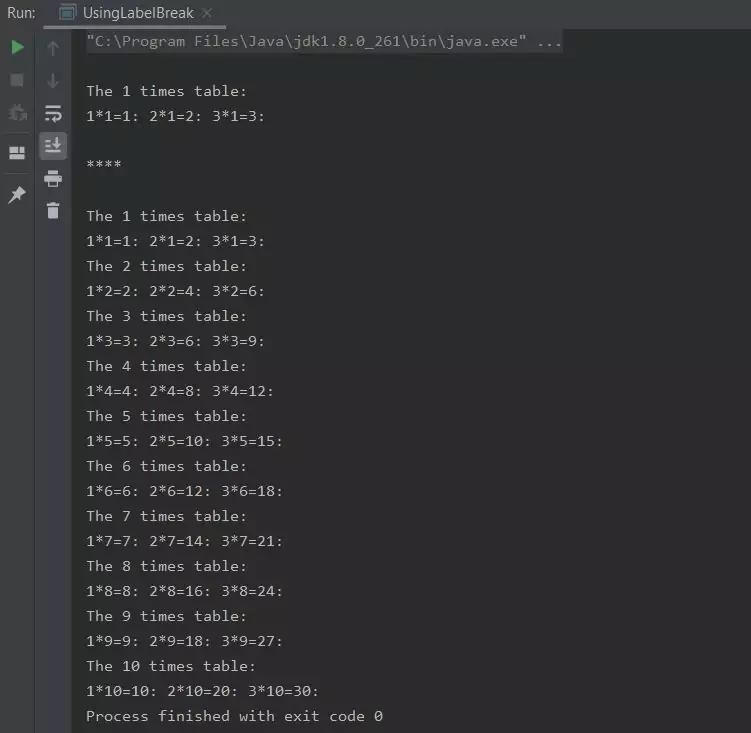
UsingLabelBreak
class.In the first loop above we break to the label1:
label, which results in processing continuing after the outer loop. So in reality we have broken out of both loops. In the second example we break to the
label2:
label, which breaks us out of the inner loop only. So processing will continue with the next iteration of the outer loop. So when using the label
statement with the break
statement positioning matters.
The enhanced for
ConstructTop
The enhanced for
construct will loop through each element within a collection. Lets look at some code to illustrate how this works:
package info.java8;
/*
enhanced for Construct
*/
public class EnhancedFor {
public static void main (String[] args) {
int [] anArray = {22,33,44,55};
for (int i : anArray) {
System.out.println("Array element = " + i);
}
}
}
Running the EnhancedFor
class produces the following output:
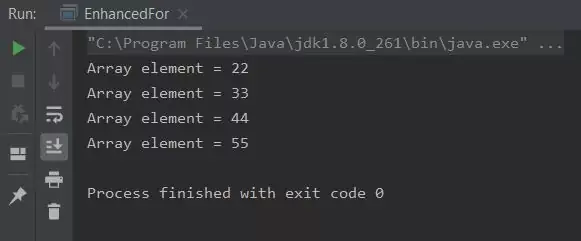
EnhancedFor
class.What we are doing here is creating an array collection of numbers; we will expand on arrays in the Arrays lesson. We then use the enhanced for
construct to iterate over the array collection.
Related Quiz
Fundamentals Quiz 13 - for
Construct Quiz
Lesson 14 Complete
In this lesson we investigated for
Construct available for use in Java.
What's Next?
In the next lesson we take our second look at loop statements when we look at the while
construct.