while
ConstructJ8 Home « while
Construct
In this lesson we take our second look at the loop statements available in Java. Loop statements allow us to iterate over some code multiple times. There are two loop statements in Java, the for
Construct which we discussed in the last lesson and the while
construct which we discuss here.
The while
ConstructTop
The while
Construct can be used to loop through a section of code while a condition remains true
. The while
Construct has two different variations known commonly as the while loop and the
do-while loop.
The following table shows the different forms of the while
construct that can be used. We are using blocks of code to wrap statements which is optional when using a single statement, but good practice and will be used here.
Construct | Description |
---|---|
while loop | |
while (condition) { | The condition can be any expression that results in a boolean and the loop will continue while the expression
remains true , processing the statement body on each pass.When the condition returns false the loop ends and control is passed to the next line following the
loop.Therefore if the condition starts as false the loop will never be entered. |
do while loop | |
do { | Unlike the normal while loop the statement body is processed before the condition
is tested. The condition can be any expression that results in a boolean and the loop will continue while the expression remains true .When the condition returns
false the loop ends and control is passed to the next line following the loop.Therefore even if the condition starts as false the loop will always execute at least once. |
We will go through the different forms of the while
construct one at a time, using some code to make understanding of the above table easier.
The while
Construct and break
Top
The while
construct will loop through a section of code while a condition remains true
. Lets look at some code to illustrate how this works:
package info.java8;
/*
while Construct
*/
public class UsingWhile {
public static void main (String[] args) {
int i = 1;
while (i < 13) {
System.out.println("The square of " + i + " = " + i*i);
i++;
}
i = 1;
while (i < 13) {
// Using a break to exit loop
if (i == 6) { break; }
System.out.println("The square of " + i + " = " + i*i);
i++;
}
}
}
Running the UsingWhile
class produces the following output:
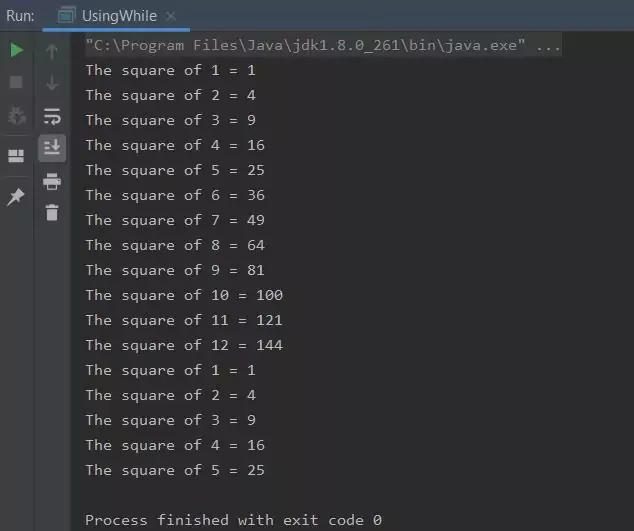
UsingWhile
class.In the first loop what we are doing here is using the control variable (i) and squaring it for the output while it is less than 13. We increment the control variable by 1 just before exiting the while
loop
each time. In the second loop we break
from the loop when the control variable (i) reaches 6. The break
statement forces an exit from the loop immediately.
Using a Nested while
Construct and break
Top
We can also nest the while
construct within another while
construct (or any other construct for that matter). Lets look at examples of this:
package info.java8;
/*
Nested while Construct
*/
public class NestedWhile {
public static void main (String[] args) {
int i = 1;
while (i < 11) {
System.out.println("\nThe " + i + " times table: ");
int j = 1;
while (j < 11) {
System.out.print(j + "*" + i + "=" + j*i + ": ");
j++;
}
i++;
}
i = 1;
while (i < 11) {
System.out.println("\nThe " + i + " times table: ");
int j = 1;
while (j < 11) {
// Using a break to exit inner loop
if (j == 4) { break; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
j++;
}
i++;
}
}
}
Running the NestedWhile
class produces the following output:
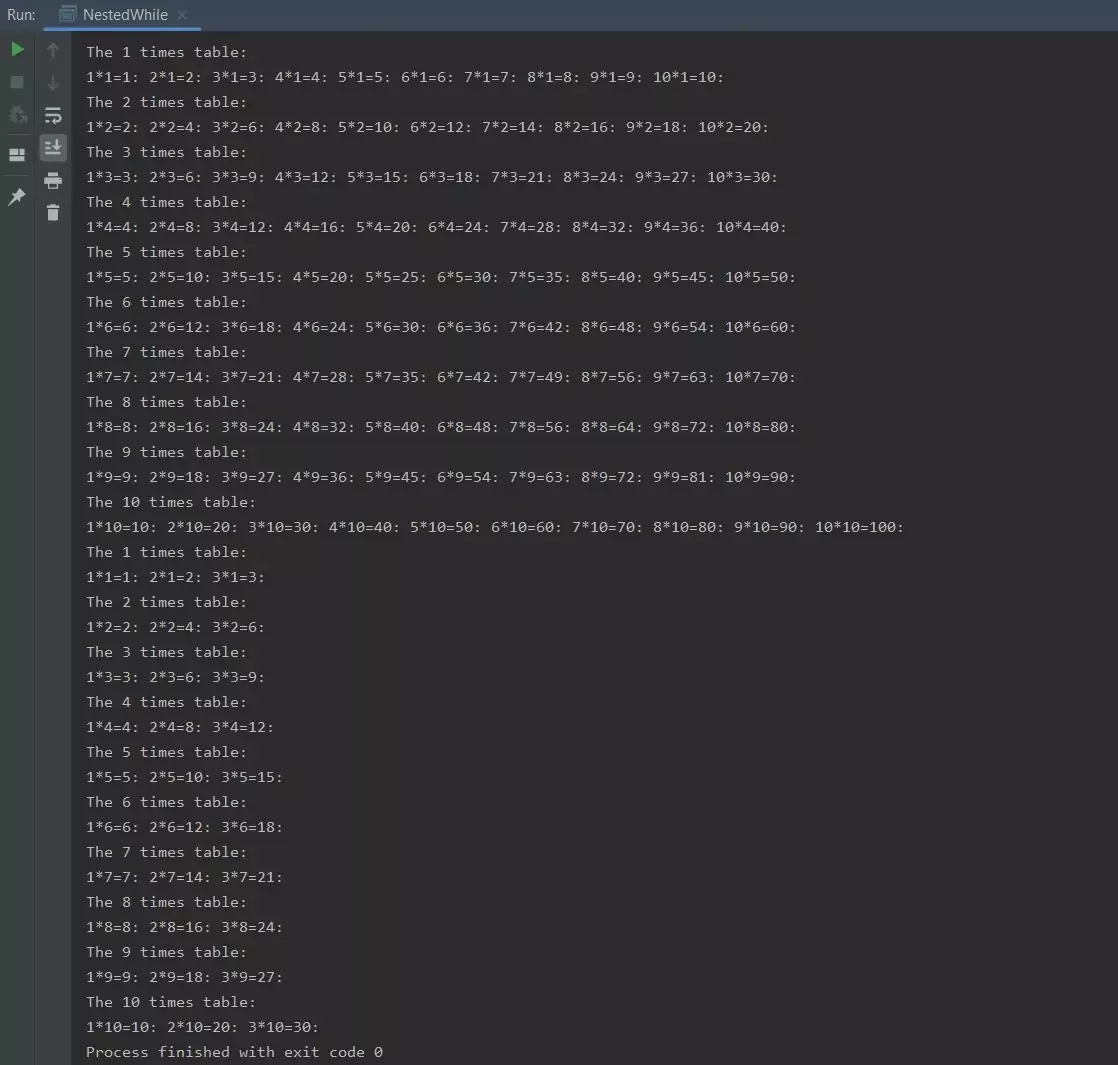
NestedWhile
class.What we are doing here is running a loop within a loop to print off times tables up to 10. So the inner while
loop runs 10 times for each outer while
loop iteration. In the second example we break from the
inner loop during the fourth iteration. This is just to show that using the break
statement forces an exit from the loop you are in not any outer loops.
Using the continue
StatementTop
For a while
construct, the continue
statement will pass control back to the start of the loop immediately. Lets look at an example of this:
package info.java8;
/*
Using continue in a while Construct
*/
public class UsingWhileContinue {
public static void main (String[] args) {
int i = 1;
while (i < 13) {
// Using a continue to force an iteration
if ((i%2) == 0) { i++; continue; }
System.out.println("The square of " + i + " = " + i*i);
i++;
}
}
}
Running the UsingWhileContinue
class produces the following output:
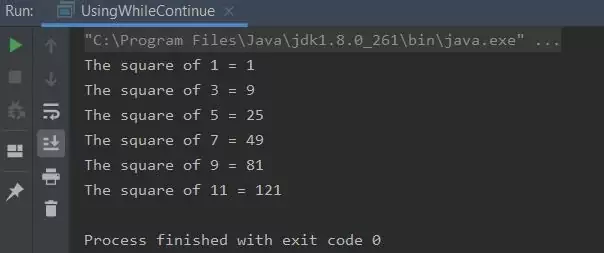
UsingWhileContinue
class.In this variation we are checking to see if the control variable (i) leaves no remainder. If this is the case we are forcing a continue
to the next iteration. For this example it means we are only printing out odd
squares within the specified range. If you notice when we hit this condition we have to increment our control variable before passing control back to the top of the loop. Unlike the for
construct, the while
has no auto increment and hence if we do not increment, we end up in an infinite loop situation.
Using the break
to a labelled StatementTop
We saw how the break
statement worked with the while
construct in earlier examples. There is a variation where we can break
to a label
statement. Breaking to a labelled
statement causes program execution to resume after the labelled block. Lets look at examples of this:
package info.java8;
/*
Using break to a label in a while Construct
*/
public class UsingWhileLabelBreak {
public static void main (String[] args) {
int i = 1, j = 1;
label1:
while (i<11) {
System.out.println("\nThe " + i + " times table: ");
j = 1;
while (j<11) {
// Using a break to label, to exit outer loop
if (j == 4) { i++; break label1; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
j++;
}
i++;
}
System.out.println("\n\n****");
i = 1;
while (i<11) {
System.out.println("\nThe " + i + " times table: ");
j = 1;
label2:
while (j<11) {
// Using a break to label, to exit inner loop
if (j == 4) { j++; break label2; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
j++;
}
i++;
}
}
}
Running the UsingWhileLabelBreak
class produces the following output:
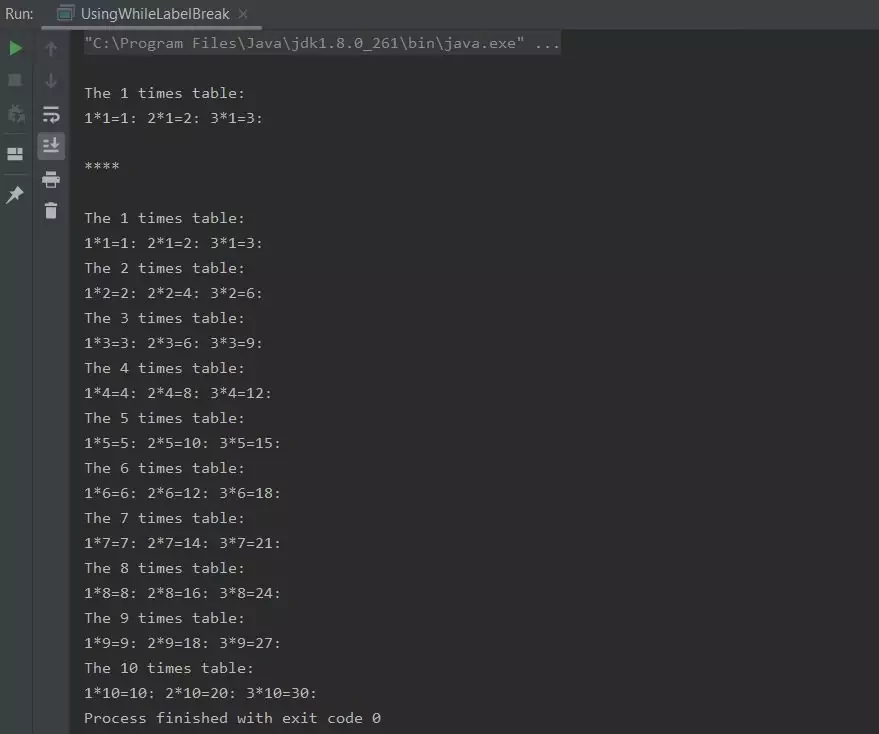
UsingWhileLabelBreak
class.In the first loop above we break to the label1:
label, which results in processing continuing after the outer loop. So in reality we have broken out of both loops. In the second example we break to the
label2:
label, which breaks us out of the inner loop only. So processing will continue with the next iteration of the outer loop. So when using the label
statement with the break
statement positioning matters.
The do while
ConstructTop
The do while
construct will loop through a section of code while a condition remains true
. Because the statement body is executed before the condition is tested the do while
construct
will always execute at least once. Lets look at some code to illustrate how this works:
package info.java8;
/*
do while Construct
*/
public class UsingDoWhile {
public static void main (String[] args) {
int i = 13;
while (i < 13) {
System.out.println("WHILE. The square of " + i + " = " + i*i);
i++;
}
do {
System.out.println("DO WHILE. The square of " + i + " = " + i*i);
i++;
} while (i < 13);
}
}
Running the UsingDoWhile
class produces the following output:
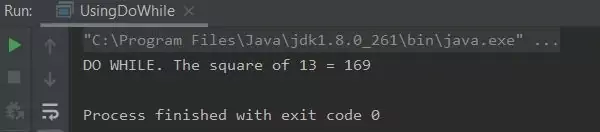
UsingDoWhile
class.In the while
loop the control expression returns false
and so the loop never runs. In the do while
loop the control expression still returns false
but the loop is always
guaranteed to execute at least once, which is what happens in this case.
Apart from this the do while
behaves exactly the same as a normal while
loop and can use the break
, continue
and label
statements in the same way.
Related Quiz
Fundamentals Quiz 14 - while
Construct Quiz
Lesson 15 Complete
In this lesson we investigated the while
Construct available for use in Java.
What's Next?
We begin a new section about objects and classes and start the section with a look at arrays.