ArraysJ8 Home « Arrays
In this lesson we start our investigation of objects and classes by looking at arrays and how to use them. in Java the predefined data type Array
object is used for array manipulation. Investigation of this
object will give us an insight into using classes before we start to create our own.
Array Definition Top
An array in Java is an object that contains a collection of values which can be a collection of primitive data types or a collection of reference variable types.
- An array of primitives data types is a collection of values that constitute the primitive values themselves.
- An array of reference variable types is actually a collection of pointer values which point to the memory address where each object is stored on the
Heap
.
Whichever variable type the array holds, primitive or reference, the array itself is still an object. in Java you can define a one dimensional array which is an object that refers to a collection of values repeated one or more times, or multi dimensional arrays which are a collection of array objects (arrays of arrays). Multi dimensional arrays may contain the same number of elements in each row or column and are known as regular arrays or an uneven number of elements in each row or column which are known as irregular arrays.
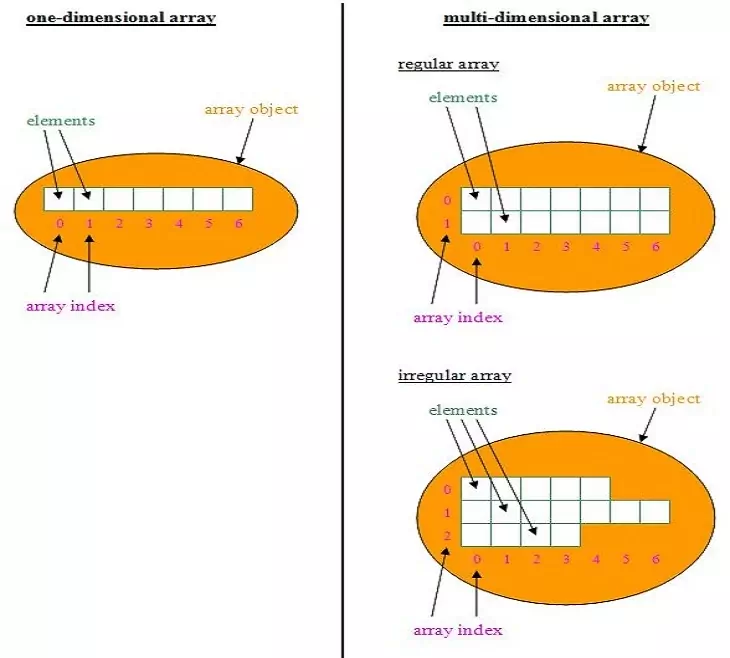
Array Creation Top
Array creation is a three step process as outlined below and can be achieved using separate statements or combined into a single statement.
- Array declaration
- Array allocation
- Array element initialization
The following table shows the different forms of the array
construct that can be used.
Construct | Description |
---|---|
One dimensional array examples | |
int intArray1[]; | Array declaration. |
intArray1 = new int[4]; | Array allocation. Array elements initialized to their default value. The new keyword is mandatory for multiple statement array creation. |
for (int i=0; i<intArray1.length; i++) { |
Array element initialization using a loop. Array element initialization using a single element. |
int intArray1[] = {0, 1, 5, 8};
| Single statement array declaration The size of the array is calculated by the number of values that are assigned to the array and should not be specfied for single statement array creation. |
Two dimensional array examples | |
int intArray1[][]; | Array declaration. |
intArray1 = new int[2][4]; | Array allocation. Array elements initialized to their default value. The new keyword is mandatory for multiple statement array creation. |
for (int i=0; i<intArray1.length; i++) { | Array element initialization using a loop. Array element initialization using a single element. |
int intArray1[][] = { {6, 2}, {5, 22, 67} }; | Single
statement array declaration The size of the array is calculated by the number of values that are assigned to the array and should not be specfied for single statement array creation. |
Multi dimensional array | |
type arrayName[][]...[] = new type[size1][size2]...[sizeN] | Same rules as one and two dimensional arrays. |
Array Notes Top
As you can see from the table above there is a lot of ways to create arrays in Java. There are several points about arrays shown in the table above that we will highlight again here before we go into some code examples:
- Array indexes are zero-based.
- When declaring an array the square brackets can appear after the type, after the array name or in the case of multi dimensional arrays a combination of both.
- After allocation of an array, each element of the array is initialized with the default for the array type:
- object -
null
- boolean -
false
- char -
/u0000
- integer types (
byte
,short
,int
andlong
) -0
- floating-point types (
float
,double
) -0.0
- object -
- For multiple statement array creation the
new
keyword is mandatory. - For single statement array creation the size of the array is calculated by the number of values that are assigned to the array and should not be specfied.
Java Documentation Top
As mentioned in the first section Java comes with very rich documentation. The following link will take you to the Oracle online version of documentation for the Java™ Platform, Standard Edition 8 API Specification. Take a look at the documentation for the Array
class which you can find by scrolling down the lower left pane and clicking on Array. Take a look through the class so you get a feel for the documentation style and the Array
class itself. You will go back to this documentation time and time again so if you haven't done so already I suggest adding this link to your browser's favourites toolbar for fast access.
Related Quiz
Objects & Classes Quiz 1 - Arrays
Lesson 1 Complete
In this lesson we looked at the various types of array for use in Java.
What's Next?
In the next lesson we look at some examples of arrays and some of the exceptions that can occur when using them.