Final Instance VariablesJ8 Home « Final Instance Variables
In the last lesson we looked at Instance Variables & Scope, in this lesson we finish our examination of instance variables by examining instance variable constants.
We make the value of an instance variable a constant by using the final
access modifier which means that once assigned a value it can never be changed.
Final Instance Variable Rules Top
1. You must always assign a value to an instance variable marked as final
before using it or you get a compiler error:
package info.java8;
/*
Using a final instance variable before assignment
*/
public class FinalInstanceVarUnassigned {
final int i;
FinalInstanceVarUnassigned() {
System.out.println(i);
}
}

FinalInstanceVarUnassigned
class.The above screenshot shows the result of trying to compile class FinalInstanceVarUnassigned
.
2. An instance variable that is marked as final
can never change once it has been assigned a value.
package info.java8;
/*
Trying to reassign a final instance variable
*/
public class FinalInstanceChanged {
final int i = 1;
FinalInstanceChanged() { // Constructor
i = 2;
}
}

FinalInstanceChanged
class.The above screenshot shows the result of trying to compile class FinalInstanceChanged
.
3. An instance variable that is marked as final
can only be assigned values when declared or in its constructor or we get a compiler error:
package info.java8;
/*
Assigning final instance variable outside declaration and construction
*/
public class FinalInstanceAfterConst {
final int i;
FinalInstanceAfterConst() {
assignFinal();
System.out.println(i);
}
public void assignFinal() {
i = 1;
}
}
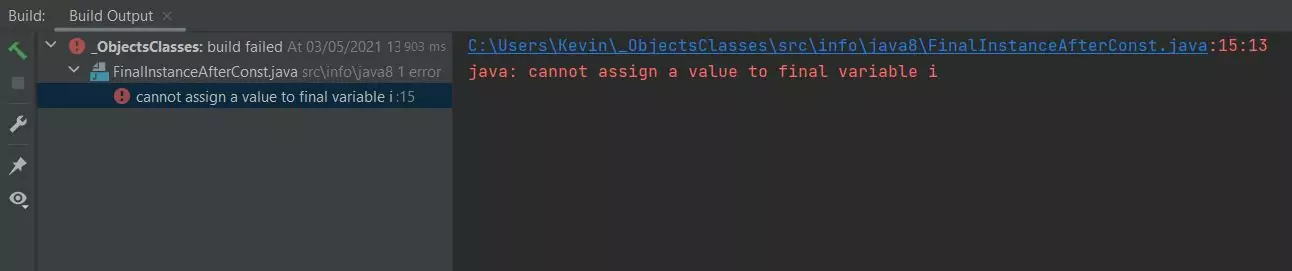
FinalInstanceAfterConst
class.The above screenshot shows the result of trying to compile class FinalInstanceAfterConst
.
Final Instance Variable Correct Use Top
The following three examples show correct initialisation of final variables at declaration or via the constructor when no inital value is assigned to a final variable:
At Declaration Top
Assign final value variable at declaration.
package info.java8;
/*
Assigning final instance variable at declaration
*/
public class FinalInstanceAtDecl {
final int i = 678;
FinalInstanceAtDecl() {
System.out.println(this.i);
}
public static void main (String[] args) {
FinalInstanceAtDecl fiad = new FinalInstanceAtDecl();
}
}
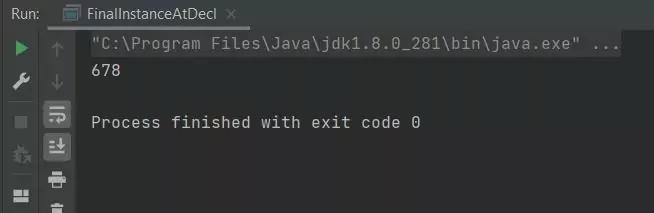
FinalInstanceAtDecl
class.The above screenshot shows the result of running the FinalInstanceAtDecl
class.
Via Constructor Parameter Top
Assign final value via constructor parameter.
package info.java8;
/*
Assigning final instance variable via constructor parameter
*/
public class FinalInstanceByConstParam {
final int i;
FinalInstanceByConstParam(int i) {
this.i = i;
System.out.println(this.i);
}
public static void main (String[] args) {
FinalInstanceByConstParam fibcp = new FinalInstanceByConstParam(2706);
}
}
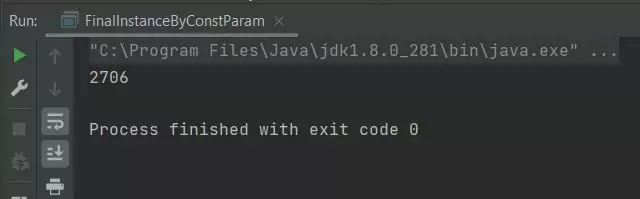
FinalInstanceByConstParam
class.The above screenshot shows the result of running the FinalInstanceByConstParam
class. There is a lengthy discussion on using the this
keyword in the Overloaded Constructors and this
lesson, for now you just need to know that it passes an implicit reference to the invoking object.
Within Constructor Top
Assign final value within constructor.
package info.java8;
/*
Assigning final instance variable within constructor
*/
public class FinalInstanceWithinConst {
final int i;
FinalInstanceWithinConst() {
i = 8657;
System.out.println(i);
}
public static void main (String[] args) {
FinalInstanceWithinConst fiwc = new FinalInstanceWithinConst();
}
}
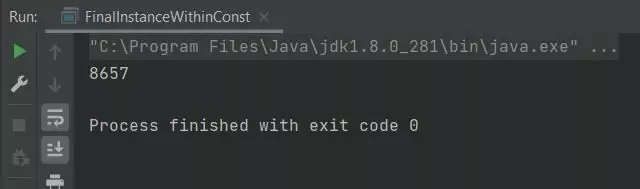
FinalInstanceWithinConst
class.The above screenshot shows the result of running the FinalInstanceWithinConst
class.
Related Quiz
Objects & Classes Quiz 9 - Final Instance Variables
Lesson 9 Complete
This lesson was about final instance variables and how to use them correctly.
What's Next?
In the next lesson we learn about constructors, which we can use to instantiate objects of our classes.