Static MethodsJ8 Home « Static Methods
In the last lesson we looked at static variables and their scope. In this lesson we continue our investigation of statics by looking at static methods.
Static methods run without any instance of a class being involved, as static they belong to the class as a whole, and are accessed using the name of the class in the same way as static variables. Lets think about it,
the main()
method we have used since the start of these lessons to test our classes, runs before any instances of a class exist.
In fact you cannot access the instance variables of a class from a static method within the class or the compiler gets upset. This makes sense as how would a static method know which instance variable to use, there is no reference.
Its the same thing when you try to call a non-static method from a static method, the compiler doesn't like it. The static method has no way of knowing how to use any instance variables associated with the non-static method and has no way to reference which instance they belong to. Even if the method has no instance variables the compiler still won't allow it because in the future you may amend the method to include an instance variable.
Lets look at these rules with some code:
package info.java8;
/*
Test class for static calls
*/
public class StatCalls {
public static void main (String[] args) {
int aSquare = squareNumber(5); // Calling non-static method
System.out.println(aSquare);
}
/*
A method that squares and returns the passed integer
*/
int squareNumber(int number) {
int square = number * number;
return square; // Here we use the return keyword to pass back a value
}
}

StatCalls
class.The above screenshot shows the output of running our StatCalls
class. The compiler doesn't like the fact that we are calling a non-static method from the main()
method. Lets look at using instance
variables from a static method.
package info.java8;
/*
Test class for static calls
*/
public class StatCalls2 {
int number;
public static void main (String[] args) {
number = 5; // Calling instance variable
int aSquare = StatCalls2.squareNumber(5); // Calling static method
System.out.println(aSquare);
}
/*
A method that squares and returns the passed integer
*/
static int squareNumber(int number) {
int square = number * number;
return square; // Here we use the return keyword to pass back a value
}
}

StatCalls2
class.The above screenshot shows the output of running our StatCalls2
class. The compiler doesn't like the fact that we are accessing an instance variable from the main()
static method.
Of course there is no problem using instantiation from within your static method to access non-static content as we have seen in many previous lessons when we instantiate objects from the main()
static method, or using a local reference from within your static method to access non-static content as shown below, otherwise you would never be able to get out of the main()
method.
package info.java8;
/*
Test class for accessing non-static from static
*/
public class AccessNonStatic {
int nonStatic = 28;
public static void main (String[] args) {
AccessNonStatic ans = new AccessNonStatic();
System.out.println(ans.nonStatic); // Calling non-static
}
}
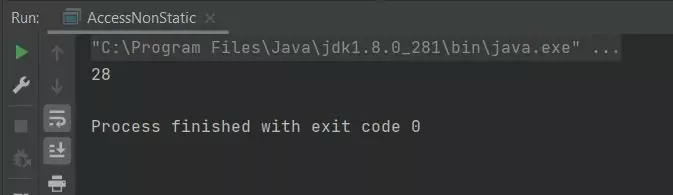
AccessNonStatic
class.The above screenshot shows the output of running our AccessNonStatic
class. As you can see by using the local reference to the class we are able to access the instance variable.
Related Quiz
Objects & Classes Quiz 13 - Static Overview
Lesson 13 Complete
In this lesson we looked at static methods and the rules on how to use them within our Java classes.
What's Next?
In the next lesson we look at Java constants and static initializer blocks.