Static Methods QuizJ8 Home « Static Methods Quiz
Java Objects & Classes Quiz 13
The quiz below tests your knowledge of the material learnt in Objects & Classes - Lesson 13 - Static Methods.
Question 1 : What will be output from the following code snippet?
public class StatCalls {
public static void main (String[] args) {
int aSquare = squareNumber(5);
System.out.println(aSquare);
}
int squareNumber(int number) {
int square = number * number;
return square;
}
}
- The code snippet will not compile as a non-static method cannot be referenced from a static content.
Quiz Progress Bar
Quiz 1
Arrays
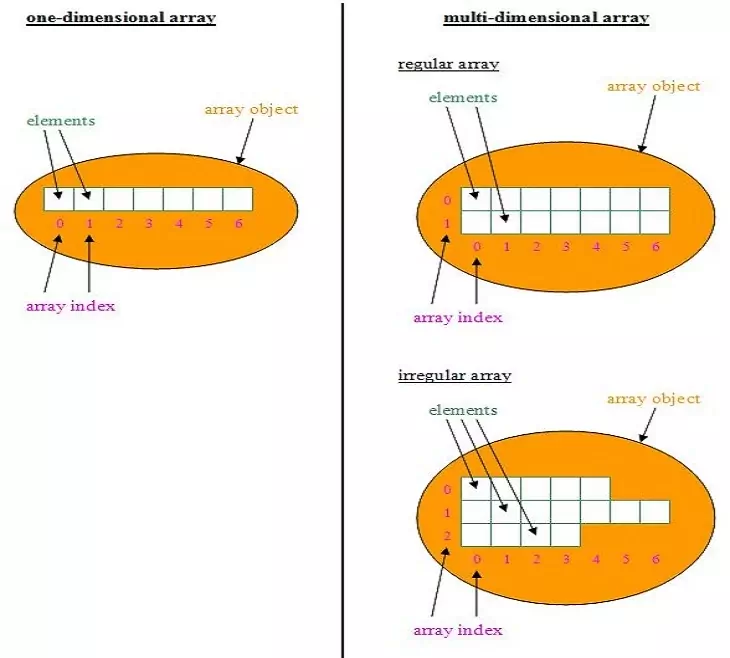
Quiz 2
Array Examples & Exceptions
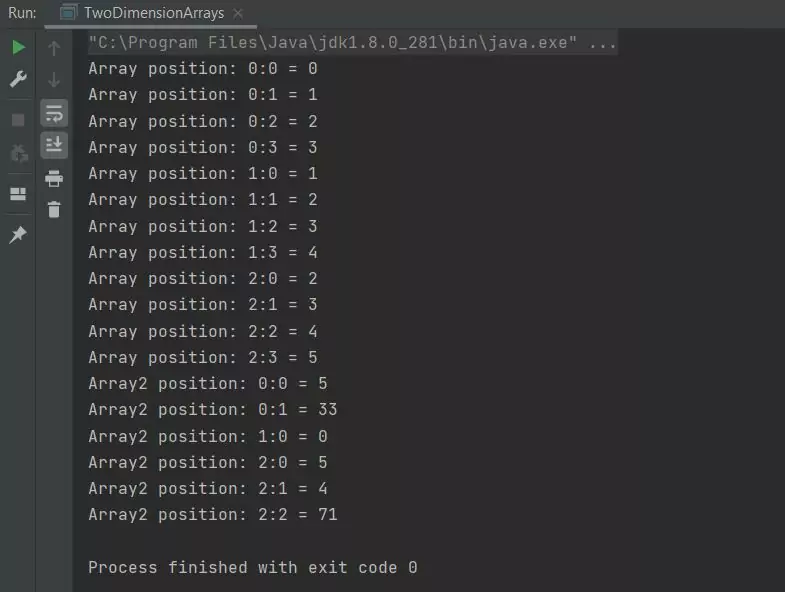
Quiz 3
Class Structure & Syntax
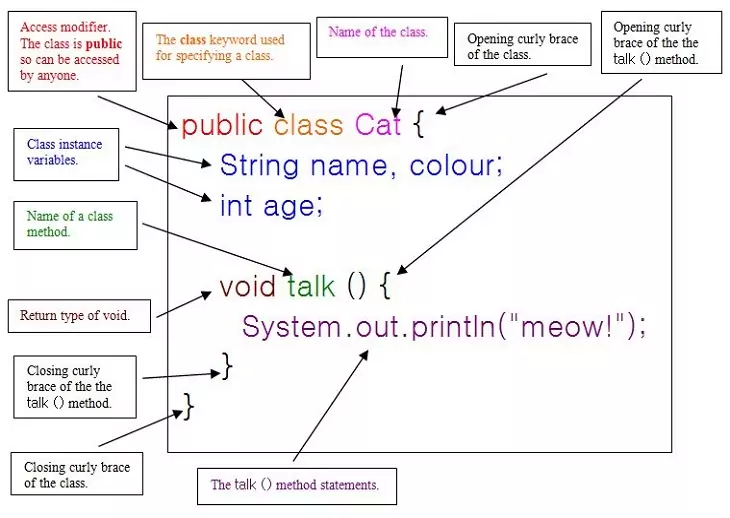
Quiz 4
Reference Variables
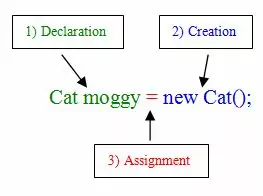
Quiz 5
Methods - Basics
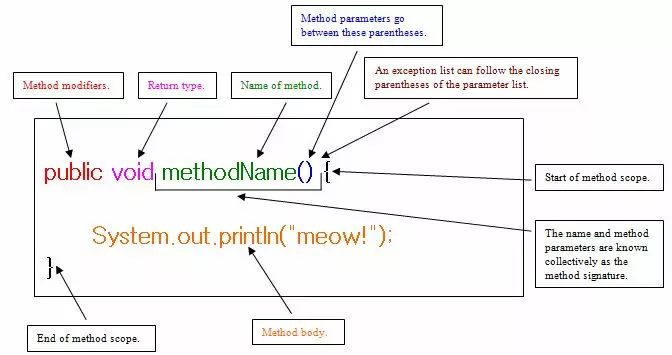
Quiz 6
Methods - Passing Values
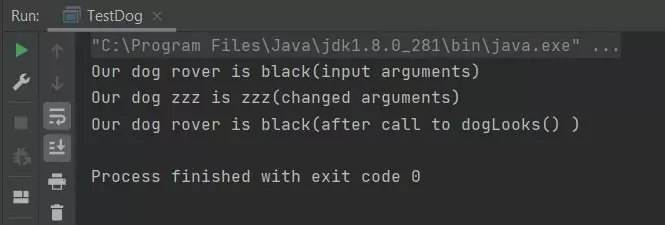
Quiz 7
Methods - Overloading & Varargs
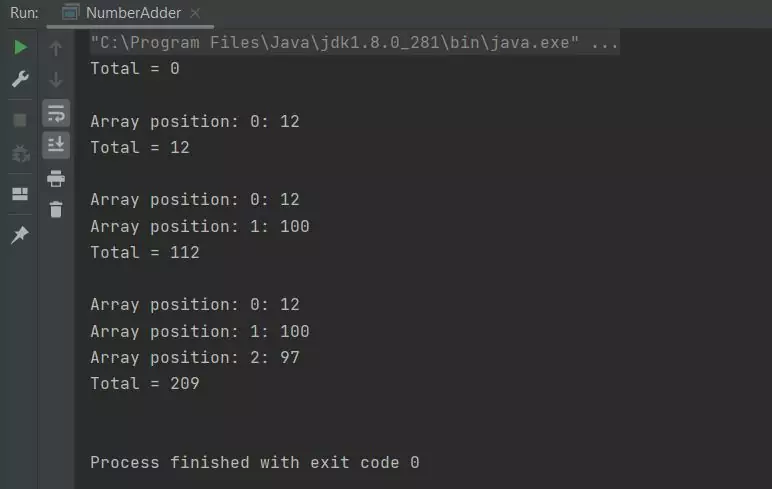
Quiz 8
Instance Variables & Scope
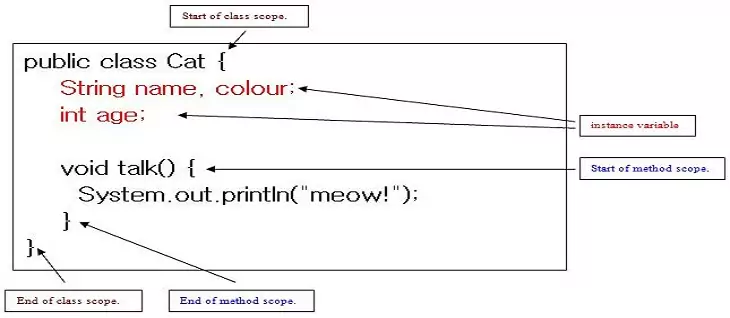
Quiz 9
Final Instance Variables
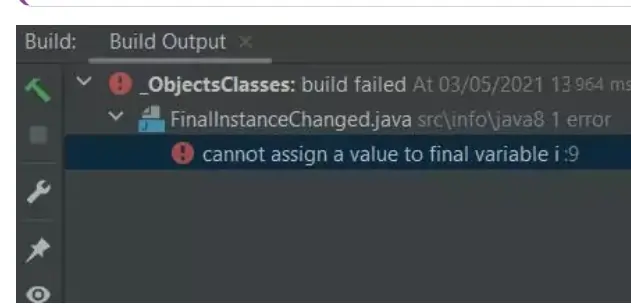
Quiz 10
Constructor Basics
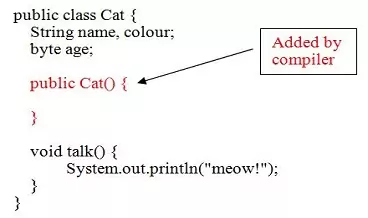
Quiz 11
Overloaded Constructors and this
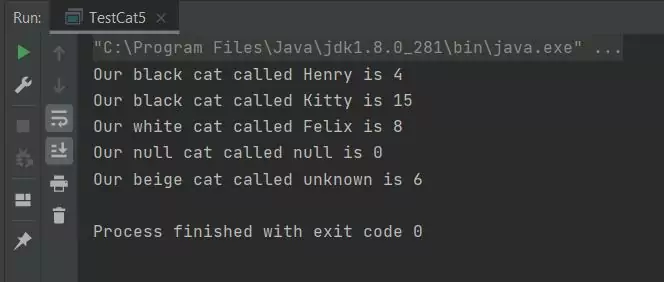
Quiz 12
Static Overview
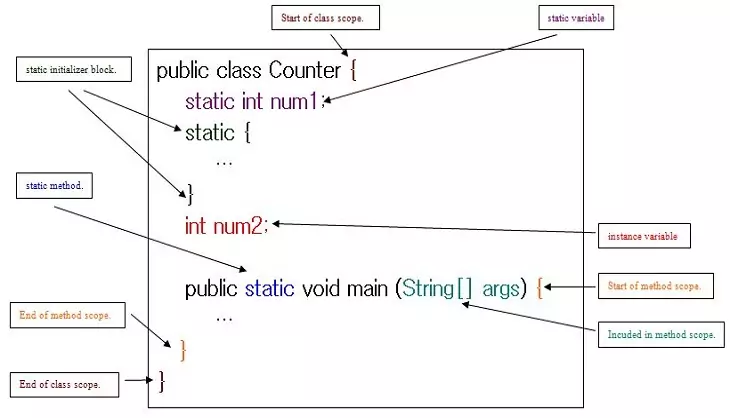
Quiz 14
Java Constants
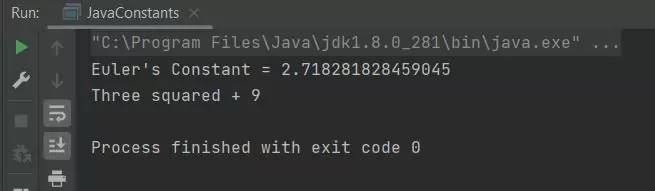
Quiz 15
Basic Enumerations
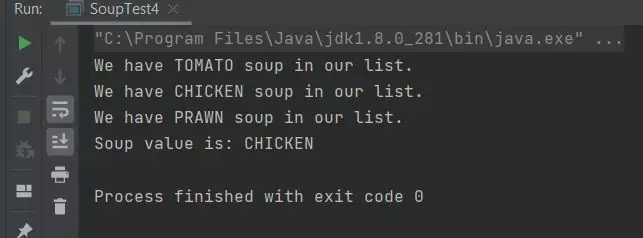
Quiz 16
Advanced Enumerations
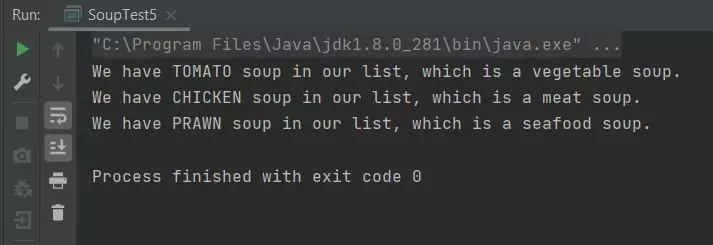
What's Next?
The next quiz we investigate Java constants and how to use static initializer blocks.