Exception OverviewJ8 Home « Exception Overview
In this lesson we begin our studies of Java exceptions by looking at the Java exception hierarchy and the various classes within it. We then list the methods from the Throwable
class along with the
checked and runtime exceptions available within the java.lang
package, which is implicitly imported into all our programs.
Before we look at the hierarchy lets define the terminology used in the exceptions part of the Flow Control section. An exception refers to an exceptional condition that has occurred to alter the
normal flow of our programs. When an exception occurs it will get thrown by the JVM
or we can determine conditions to throw an exception ourselves when required.
We can catch thrown exceptions and the code we write for this purpose is known as an exception handler. We can also declare exceptions in our method definitions, when we know for instance that
the method will try to read a file that may or may not be present.
At the top of the exception hierarchy is the Throwable
class, which all other error and exception classes inherit from. The Throwable
class creates a snapshot of the stack trace for exceptions
when they occur and may also contain information about the exception. We can use this information for debugging purposes. Only instances of the Throwable
class, or subclasses of it, can be
thrown by the JVM
or be thrown by us.
Two subclasses inherit from the Throwable
class and these are the Error
and Exception
classes. These classes, and subclasses
thereof, are used to create an instance of the appropriate error or exception along with information about the exception:
- The
Error
class and its subclasses handle error situations from within theJVM
itself and so are outside our control as programmers. As a general rule we can't recover from anError
situation and because of this we are not expected to handle anError
when it occurs. - The
Exception
class and its subclasses are what concern us more as programmers and these can be split into two categories:- Runtime exceptions: Are exceptions that are not checked for by the compiler and for this reason are also known as unchecked exceptions. Generally exceptions of type
RuntimeException
originate from problems in our code, such as an attempt to divide by zero which gives anArithmeticException
. These sorts of errors are within our control and as such should be handled by the code itself. - Checked exceptions: Are exceptions that are checked for by the compiler and if present and not declared, will give a compiler error. An example of this is a
FileNotFoundException
where a file we want to read may or may not be available. Whether the file is available or not is outside our control. So when we read the file, we need to handle or declare that this exception, or a superclass of it may occur.
- Runtime exceptions: Are exceptions that are not checked for by the compiler and for this reason are also known as unchecked exceptions. Generally exceptions of type
Exception Hierarchy Diagram Top
The diagram below is a representation of the exception hierarchy and by no means a complete list, but should help in visualisation:
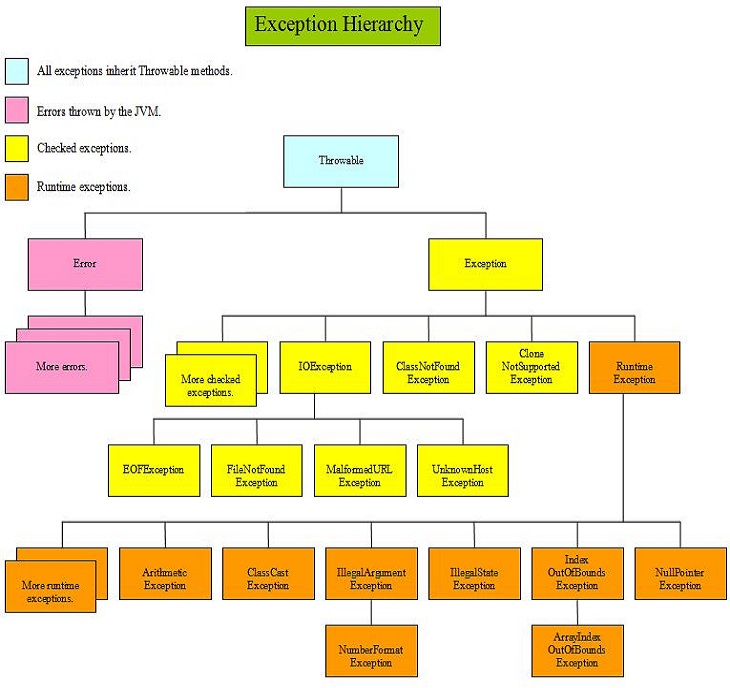
In the diagram above each box represents a class and as you can see the Exception
class, which interests us as programmers, has lots of subclasses attached to it. We are just showing some of them in
the above diagram as space permits, but I am sure you get the idea. When an exception happens an exception object of the appropriate class is created and we can use code to handle the exception. We will go into
the mechanics of how we achieve this in much greater detail over the next few lessons. For the rest of this lesson we are just going to show the methods of the Throwable
class and the
exceptions that exist in the java.lang
package. Hopefully this will give a feel for the overall architecture before we start handling exceptions ourselves.
Throwable
Methods Top
The table below shows the declarations of the methods from the Throwable
class which being the superclass of all exceptions we can use when handling exceptions. For full details of the Throwable
class the following link will take you to the online version of the Oracle documentation JavaTM 2 Platform Standard Edition 5.0 API Specification, where you
can look at the Throwable
class in more detail. You can find all the documentation by scrolling down the lower left pane and clicking on Throwable.
Method Declaration | Description |
---|---|
public Throwable fillInStackTrace() | Returns a Throwable object, that can be rethrown, which contains a completed stack trace. |
public Throwable getCause() | Returns a Throwable object, or null if the cause of the exception is nonexistent or unknown. |
public String getLocalizedMessage() | Returns a localized description of the exception. |
public String getMessage() | Returns a description of the exception. |
public StackTraceElement[] getStackTrace() | Returns an array of stack trace elements, where each element represents one stack frame. |
public Throwable initCause(Throwable cause) | Set the cause of the throwable. |
public void printStackTrace() | Displays the stack trace for the exception. |
public void printStackTrace(PrintStream s) | Sends stack trace for the exception to specified stream. |
public void printStackTrace(PrintWriter s) | Sends stack trace for the exception to specified print writer. |
public void setStackTrace(StackTraceElement[] stackTrace) | Sets stack trace returned from getStackTrace() and printed by printStackTrace() and related methods. |
public String toString() | Returns a short description of this throwable exception. |
We will use the above methods as we work through this section..
java.lang
Checked Exceptions Top
The table below lists all the checked exceptions that are defined in the java.lang
package.
Checked Exception | Description |
---|---|
ClassNotFoundException | Class requested not found. |
CloneNotSupportedException | Occurs when an attempt is made to clone an object of a class that doesn't implement the Cloneable interface. |
Exception | Exception and subclasses thereof that an application may want to catch. |
IllegalAccessException | Attempted access of class refused. |
InstantiationException | Occurs when an attempt is made to instantiate an object from an abstract class or interface. An example of this exception is shown in the Abstraction lesson when we look at Abstract Classes. |
InterruptedException | Occurs when a thread has been interrupted by another thread. |
NoSuchFieldException | Field requested doesn't exist. |
NoSuchMethodException | Method requested doesn't exist. |
java.lang
Runtime Exceptions Top
The table below lists all the runtime exceptions that are defined in the java.lang
package.
Runtime Exception | Description |
---|---|
ArithmeticException | Arithmetic exception occurred. An example of this exception is shown in the Exception Handling lesson when we look at Using try catch . |
ArrayIndexOutOfBoundsException | Attempt to access array with an illegal index. An example of this exception is shown in the Arrays lesson when we look at java.lang Array Exceptions. |
ArrayStoreException | Attempt to store incompatible type into object array element. |
ClassCastException | Attempt to cast an object to a subclass of which it is not an instance. |
EnumConstantNotPresentException | Attempt to access enum constant by name that is not contained within the enum type. |
IllegalArgumentException | Method invoked with an illegal argument. |
IllegalMonitorStateException | Illegal operation performed on an object monitor. |
IllegalStateException | Method invoked while an application isn't in the correct state to receive it. |
IllegalThreadStateException | Attempt to perform an operation on a monitor whilst not owning it. |
IndexOutOfBoundsException | An index is out of bounds. |
NegativeArraySizeException | Attempt to create an array with a negative index. An example of this exception is shown in the Arrays lesson when we look at java.lang Array Exceptions. |
NullPointerException | Attempt to access an object with a null reference.An example of this exception is shown in the Reference Variables lesson when we look at The Heap . |
NumberFormatException | Invalid attempt to convert the contents of a string to a numeric format. An example of this exception is shown in the Exception Handling lesson when we look at Using try catch finally . |
RuntimeException | Runtimes Exception and subclasses thereof thrown by the JVM . |
SecurityException | Thrown when a security violation has occurred. |
StringIndexOutOfBoundsException | Attempt to access string with an illegal index from a String method.An example of this exception is shown in the String Class lesson when we look at The charAt() Method. |
TypeNotPresentException | Type not found. |
UnsupportedOperationException | Unsupported operation encountered. |
Lesson 1 Complete
In this lesson we began our studies of Java exceptions by looking at the Java exception hierarchy and the various classes within it.
What's Next?
Now we know what the Java exception hierarchy looks like and the classes involved its time to start handling exceptions which may occur within our code.