GUI ConceptsJ8 Home « GUI Concepts
In our first lesson of the section we look at GUI concepts and the MVC paradigm and how this pattern can help in removing some of the complexity from the disparate parts of a user application.
Within the preceding sections we used command line inputs to run our Java programs and for learning purposes this works just fine. In the real world any modern non-trivial user application includes a Graphical User Interface (GUI) that enables users to interact with our systems in a meaningful way. The disparate parts of a GUI are based on years of research into human computer interaction (HCI) and so the different components that make up a GUI, such as windows, scroll bars and buttons are instantly recognisable and their usability known, to even the most casual of computer users.
The user is blissfully unaware of what is happening in the background when they press a button to upload a file or update a record. As far as the user is concerned they know that pressing the 'update record' button updates the current record. Behind the scenes such an action can have multiple steps involved in persisting the changes to a database and include several tiers of the underlying architecture. There could also be a situation where several users are working on the same records concurrently and so behind the scenes we have to control access to shared data and how we choose to display it. The client and server software could even be stored on totally separate machines and need to interact remotely. Client-server programming of this sort can at first appear complicated because of all the components present in the different layers of a distributed system. From a logical viewpoint we can think of the different layers that make up the disparate parts of a distributed system as different tiers of an overall architecture. This way of conceptualising a system is often referred to as a multi-tier architecture.
Three-Tier Architecture Top
The most common form of the multi-tier architecture paradigm is known as the three-tier architecture model. The three-tier architecture model separates our client-server programming into three tiers consisting of a presentation tier, an application tier and a data tier. Each tier is developed and maintained as an independent area of work and the different tiers can, and often do, exist on different software platforms. This separation of concerns reduces the complexity of the overall architecture and means any of the tiers can be modifed or even replaced with different technologies independently and without affecting the other tiers. The following diagram is a representation of a three-tier architecture model to aid in understanding of the concept:
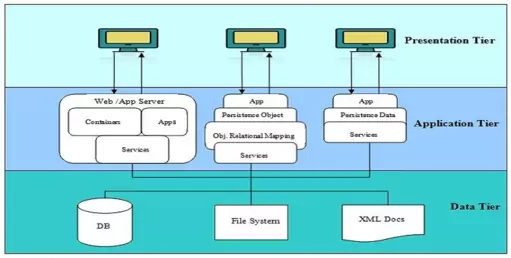
The Model-View-Controller Pattern Top
The processing involved to get even a simple multi-user GUI implemented can be quite complex and so various design patterns have emerged over the years to simplify the design of such systems. Perhaps the best known of these patterns, which also fits into the architectural model above and the one we will talk about here and use in the Case Study is the Model-View-Controller (MVC) Pattern. The aim of the MVC pattern is to separate the tasks involved in creating a GUI application into three areas, these being as the name suggests the Model, View and Controller areas. Using this pattern we can keep these regions of the application separate from each other and in the real world it's not uncommon to have different teams that specialize in and develop each region of this pattern in isolation. An added benefit of this approach is that the different parts of the system are not tightly coupled, meaning we can make changes to one part of the system without hugely impacting the rest of it. There are many variations on the MVC pattern and the diagram below is perhaps a more classic interpretation of the pattern where the view gets updated directly from the model.
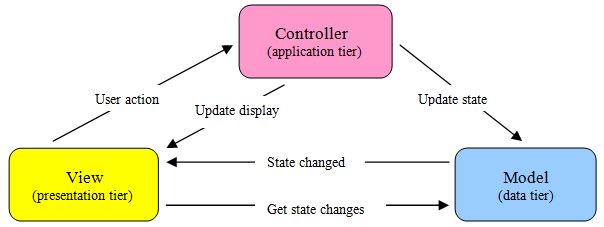
The different areas covered in the MVC pattern are discussed in the subsections below.
The Model Top
The Model acts as an interface to the underlying system state, data and application logic. The job of the Model is to provide a consistent way to retrieve and manipulate state held within an application. The Model knows nothing of the View and Controller parts of the pattern although changes to state can be notified to observers. This has the added benefit of reusability as our Model is not coupled to any specific View or Controller and so can be reused via its interface.
The View Top
The View is the physical representation of the data presented in a format suitable for the user of the data. The format in regards to these lessons is a Java GUI application, but the format could just as easily be a HTML, JSON or XTML document. The point is, it is the responsibility of the View to format the data appropriately for whatever usage is required. The View also has the responsibility of interpreting user interaction where appropriate and handling and dispatching user actions as required.
The Controller Top
The Controller allows the abstraction between the presentation and data tiers by acting as an intermediary between the View and the Model. This means that any requests by users should always be passed from the View to the Controller which will then call the Model as and when required. This separation of concerns decreases the complexity within each tier of our architecture and enhances reusability.
How Java Fits In Top
When developing our own Java GUI application we can develop the Model (data tier) and Controller (application tier) using the Core Java we have already learned in the first seven sections of the site. For our View (presentation tier) we would use the Java Swing component classes to create interactive front-ends for our users to work with. Swing, which is loosely based upon the MVC paradigm, incorporates many enhancements to the AWT toolkit used by Java for graphical content. With these two libraries we have everything required to create interactive GUIs for our users.
Java Documentation Top
Java comes with very rich documentation that you will go back to time and again. The following link will take you to the online version of documentation for the Java™ Platform, Standard Edition 8 API Specification . I suggest adding this link to your browser's favourites toolbar for fast access.
Lesson 1 Complete
In this lesson we looked at the GUI concepts and the MVC pattern.
What's Next?
In the next lesson we introduce the AWT and Swing libraries which we use to create our own GUIs within Java.