Final Instance Variables QuizJ8 Home « Final Instance Variables Quiz
Java Objects & Classes Quiz 9
The quiz below tests your knowledge of the material learnt in Objects & Classes - Lesson 9 - Final Instance Variables.
Question 1 : What will be output from this code snippet?
public class FinalInstanceVar {
final int i;
FinalInstanceVar() {
System.out.println(this.i);
}
}
- The code snippet will not compile as final instance variables must be initialized before use.
Quiz Progress Bar
Quiz 1
Arrays
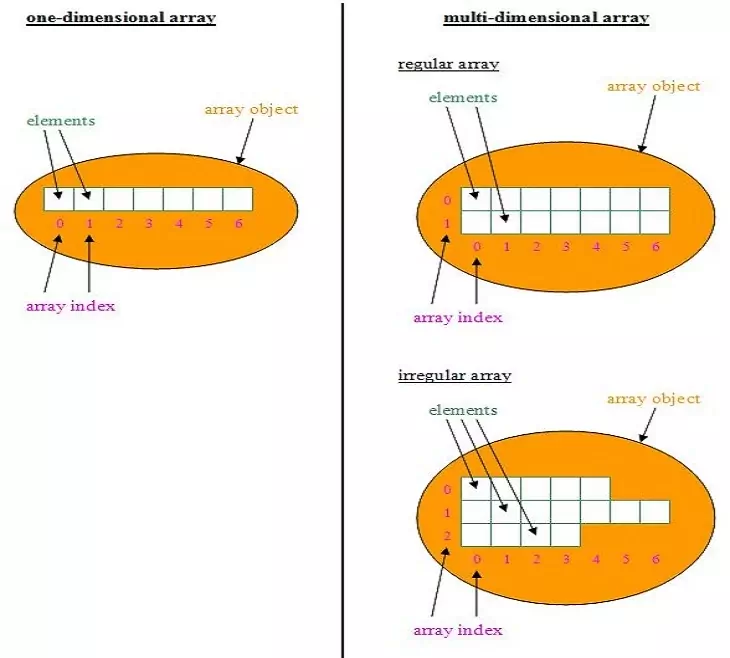
Quiz 2
Array Examples & Exceptions
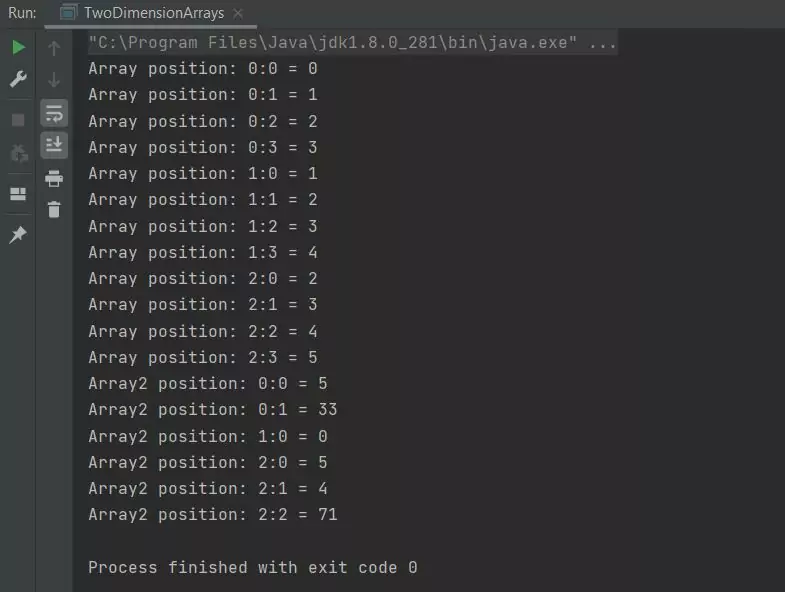
Quiz 3
Class Structure & Syntax
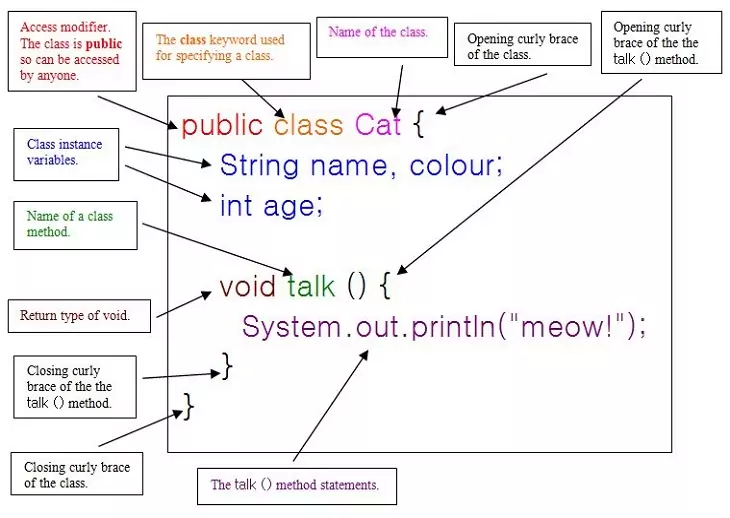
Quiz 4
Reference Variables
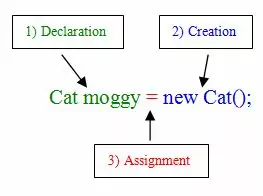
Quiz 5
Methods - Basics
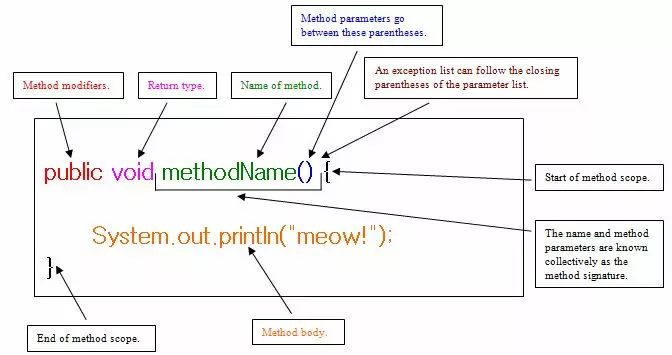
Quiz 6
Methods - Passing Values
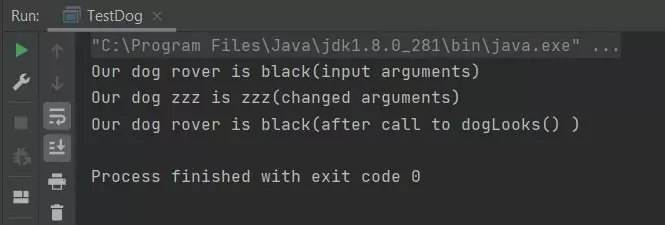
Quiz 7
Methods - Overloading & Varargs
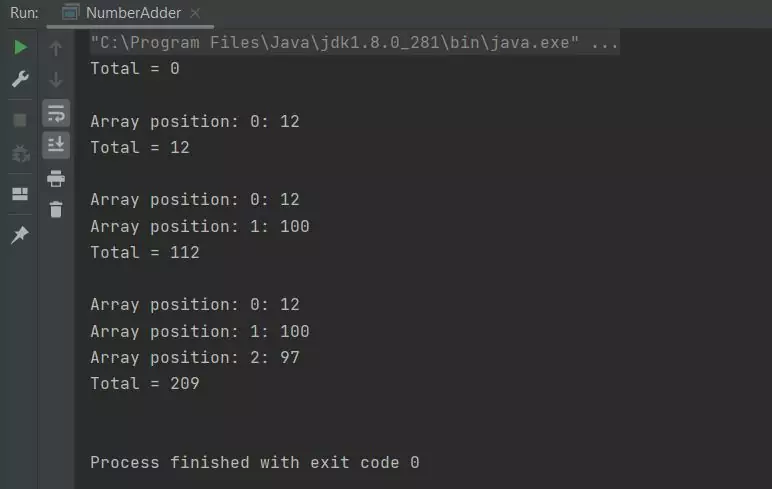
Quiz 8
Instance Variables & Scope
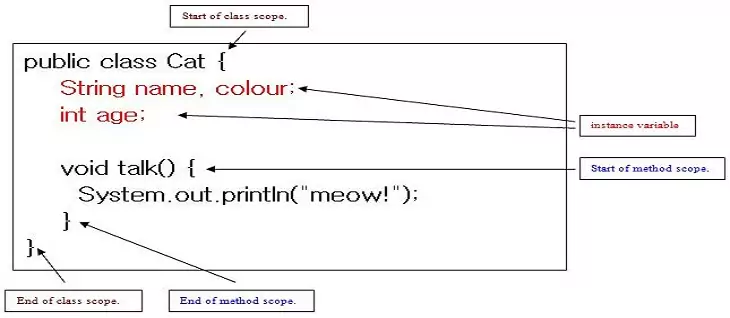
Quiz 10
Constructor Basics
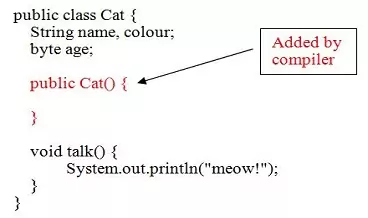
Quiz 11
Overloaded Constructors and this
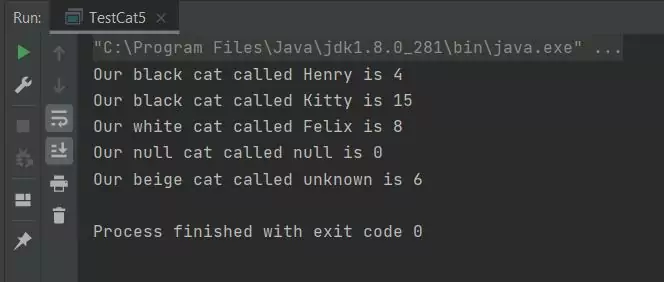
Quiz 12
Static Overview
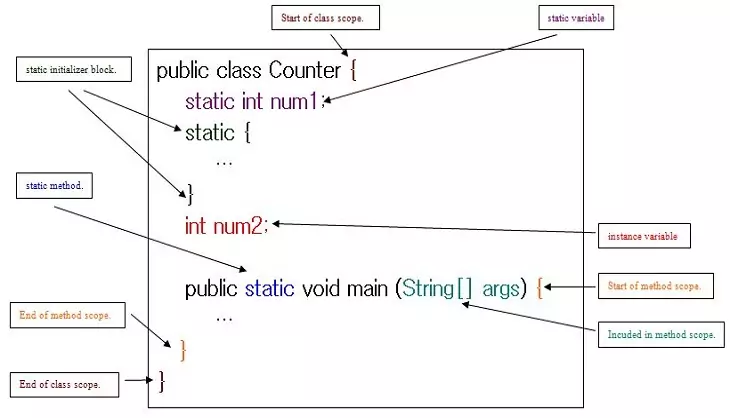
Quiz 13
Static Methods
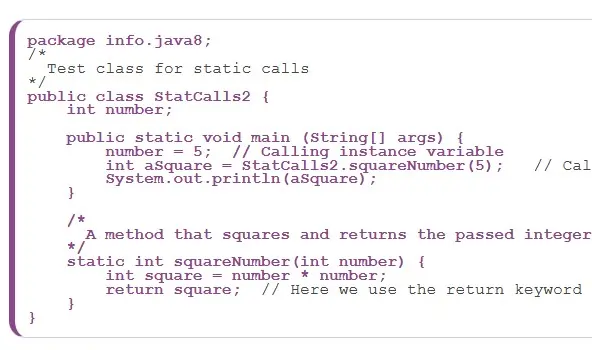
Quiz 14
Java Constants
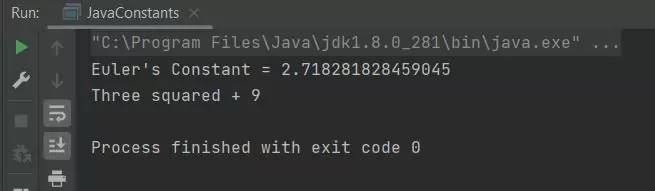
Quiz 15
Basic Enumerations
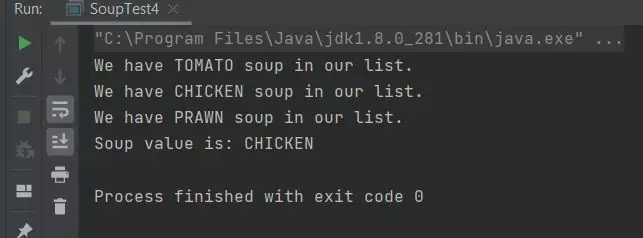
Quiz 16
Advanced Enumerations
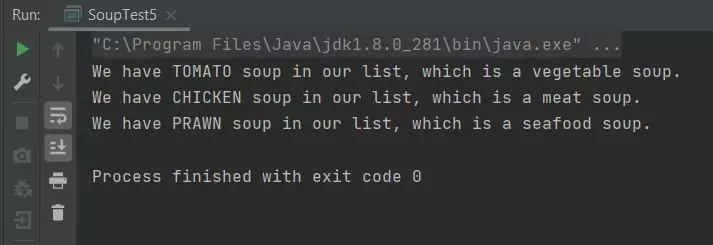
What's Next?
In the next quiz we test your knowledge of constructors basics and how we use them to instantiate objects of our classes.