Java ConstantsJ8 Home « Java Constants
In the last two lessons we looked at static variables and their scope and static methods and their usage. In our final lesson on statics we investigate Java constants and how to use static initializer blocks.
Java Constants Top
We assign a java constant (a value that never changes) through the use of the static
and final
keywords. You must always assign a value to a static variable marked as final
before you use it or the compiler complains. A static variable that is marked as final
can never change once it has been assigned a value. So once assigned a value, trying to change a variable marked as final
will cause a compiler error.
You can also use the final
keyword to create final local variables, final instance variables,
within parameter lists and for preventing inheritance/overriding.
So lets use some code to look at creating some Java constants.
package info.java8;
/*
Test class for Java Constants
*/
public class JavaConstants {
public static final double EULERS_CONSTANT = 2.718281828459045; // Base of natural logarithms
public static final int SQUARE_OF_THREE; // 3 * 3
public static void main (String[] args) {
System.out.println("Euler's Constant = " + EULERS_CONSTANT);
System.out.println("Three squared + " + SQUARE_OF_THREE);
}
}

JavaConstants
class.The above screenshot shows the output of running the JavaConstants
class. We got a compiler error as we forgot to initialize SQUARE_OF_THREE
. Ok lets change the JavaConstants
class.
package info.java8;
/*
Test class for Java Constants
*/
public class JavaConstants {
public static final double EULERS_CONSTANT = 2.718281828459045; // Base of natural logarithms
public static final int SQUARE_OF_THREE = 9; // 3 * 3
public static void main (String[] args) {
System.out.println("Euler's Constant = " + EULERS_CONSTANT);
SQUARE_OF_THREE = 9; // Here we set the value of SQUARE_OF_THREE again
System.out.println("Three squared + " + SQUARE_OF_THREE);
}
}

JavaConstants
class.The above screenshot shows the output of rerunning the JavaConstants
class. We got a compiler error as we initialized SQUARE_OF_THREE
, and then tried to assign a value to if after this which we can't do. Lets
remove the second assignment:
package info.java8;
/*
Test class for Java Constants
*/
public class JavaConstants {
public static final double EULERS_CONSTANT = 2.718281828459045; // Base of natural logarithms
public static final int SQUARE_OF_THREE = 9; // 3 * 3
public static void main (String[] args) {
System.out.println("Euler's Constant = " + EULERS_CONSTANT);
System.out.println("Three squared + " + SQUARE_OF_THREE);
}
}
Save, compile and run the file in directory c:\_ObjectsAndClasses in the usual way.
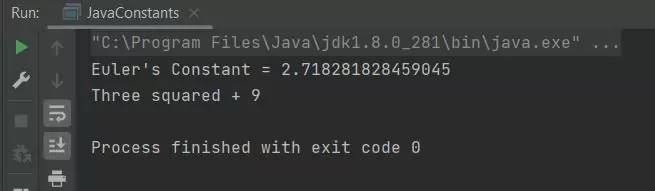
JavaConstants
class again.The above screenshot shows the output of running the JavaConstants
class, it now works fine.
Static Initializer Blocks Top
You can only initialize static variables in two ways: when you declare them or in a static initializer block. Static initializer blocks run as soon as a class is loaded, before static variables and static methods and are used to action things we want done before anything else. Lets see how static initializer blocks work with our Java constants:
package info.java8;
/*
Test class for Java Constants
*/
public class JavaConstants2 {
public static final double EULERS_CONSTANT;
public static final int SQUARE_OF_THREE;
/*
Static Initializer Block
*/
static {
EULERS_CONSTANT = 2.718281828459045;
SQUARE_OF_THREE = 9;
}
public static void main (String[] args) {
System.out.println("Euler's Constant = " + EULERS_CONSTANT);
System.out.println("Three squared + " + SQUARE_OF_THREE);
}
}
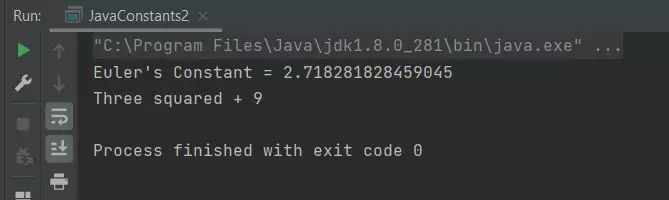
JavaConstants2
class.The above screenshot shows the output of running the JavaConstants2
class.
Java Constants Checklist Top
- There is no way to designate a constant in Java; so the convention is to use all uppercase letters and separate words using the underscore (
_
) symbol when defining a constant. - Although the
static
andfinal
keywords can go either way round the offical documentation offers advice on the order of field modifiers as follows:
Annotationpublic
protected
private
static
final
transient
volatile
. - You must initialize any static variable marked
final
before use, or the compiler throws an error. This can be done when declaring the static variable or in a static initailizer block. - Once initialized any static variable marked
final
cannot have its value changed elsewhere or the compiler throws an error.
Related Quiz
Objects & Classes Quiz 14 - Java Constants
Lesson 14 Complete
In this lesson we investigated Java constants and how to use static initializer blocks.
What's Next?
In our final lesson of this section we go through enumerations which were introduced in Java5.