Methods - Passing ValuesJ8 Home « Methods - Passing Values
In the Methods - Basics lesson we took a first look at using methods with some basic code examples and terminology to get a feel for how these members work. In this lesson we take a deeper look at passing information to a method.
Pass-By-Value Top
When we pass an argument to a method we are actually passing a copy of that argument and this is known as pass-by-value. To explore what this means lets look at some code:
package info.java8;
/*
A Dog Class
*/
public class Dog {
String name, colour;
void dogLooks(String name, String colour) {
System.out.println("Our dog " + name + " is " + colour + "(input arguments)");
name = "zzz";
colour = "zzz";
System.out.println("Our dog " + name + " is " + colour + "(changed arguments)");
}
}
Lets write a test class for our Dog
class.
package info.java8;
/*
Test Class for Dog
*/
public class TestDog {
public static void main (String[] args) {
Dog doggy = new Dog(); // Create a Dog instance
String name = "rover";
String colour = "black";
doggy.dogLooks(name, colour);
System.out.println("Our dog " + name + " is " + colour + "(after call to dogLooks() )");
}
}
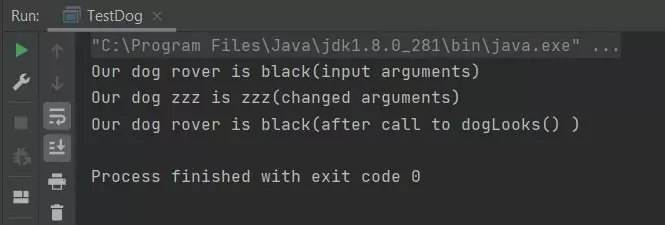
TestDog
class.The above screenshot shows the output of running our TestDog
class. As you can see even though we change the parameters in the dogLooks
method, when we return and print the passed arguments they are
the same as before the call to dogLooks
. This is because Java made a copy of the variables and passed them into our method. Therefore any changes to the variables were made to the copies and not to the originating
argument list.
Pass-By-Value Using Reference Variables Top
So what if we pass a reference variable to a method? If you recall our objects live on The Heap and we access them through their reference variable. Lets look into this:
package info.java8;
/*
Another Dog Class
*/
public class Dog2 {
String name, colour;
void dogLooks(Dog2 dog) {
System.out.println("Our dog " + dog.name + " is " + dog.colour + "(input arguments)");
dog.name = "zzz";
dog.colour = "zzz";
System.out.println("Our dog " + dog.name + " is " + dog.colour + "(changed arguments)");
}
}
package info.java8;
/*
Test Class for Dog2
*/
public class TestDog2 {
public static void main (String[] args) {
Dog2 doggy = new Dog2(); // Create a Dog instance
doggy.name = "rover";
doggy.colour = "black";
doggy.dogLooks(doggy); // Pass a reference variable to a method
System.out.println("Our dog " + doggy.name + " is " + doggy.colour
+ "(after call to dogLooks() )");
}
}
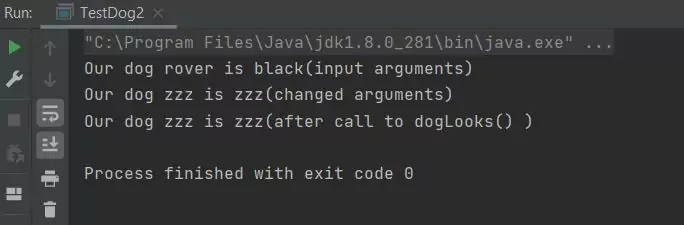
TestDog2
class.The above screenshot shows the output of running our TestDog2
class. As you can see when we change the instance variables in the method the values have changed when we return. It is still passing a copy of the
reference variable to the method, but we are updating the actual instance variables on The Heap. So the changes in the method persist when the dogLooks
method ends.
Related Quiz
Objects & Classes Quiz 6 - Methods - Passing Values
Lesson 6 Complete
In this lesson we took a second look at methods and the concept of pass-by-value.
What's Next?
In the next lesson we look at method overloading and variable arguments.