Assignment OperatorsJ8 Home « Assignment Operators
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In the third of five lessons on operators we look at the assignment operators available in Java.
Assignment Operators Overview Top
The single equal sign =
is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A
point to note here is that the type of variable must be compatible with the type of expression.
Shorthand Assignment Operators
The shorthand assignment operators allow us to write compact code that is implemented more efficiently.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
+= | Addition | int b = 5; b += 5; | 10 | |
-= | Subtraction | int b = 5; b -= 5; | 0 | |
/= | Division | int b = 7, b /= 22; | 3 | When used with an integer type, any remainder will be truncated. |
*= | Multiplication | int b = 5; b *= 5; | 25 | |
%= | Modulus | int b = 5; b %= 2; | 1 | Holds the remainder value of a division. |
&= | AND | boolean a = false; boolean a = false; boolean a = true; boolean a = true; |
false false false true | Will check both operands for true values and assign true or false to the first operand dependant upon the outcome of the expression. |
|= | OR | boolean a = false; boolean a = false; boolean a = true; boolean a = true; |
false true true true | Will check both operands for true values and assign true or false to the first operand dependant upon the outcome of the expression. |
^= | XOR | boolean a = false; boolean a = false; boolean a = true; boolean a = true; |
false true true false | Will check both operands for different boolean values and assign true or false to the first operand dependant upon the outcome of the expression. |
Automatic Type Conversion, Assignment Rules Top
The following table shows which types can be assigned to which other types, of course we can assign to the same type so these boxes are greyed out.
When using the table use a row for the left assignment and a column for the right assignment. So in the highlighted permutations byte = int
won't convert and int = byte
will convert.
Type | boolean |
char |
byte |
short |
int |
long |
float |
double |
---|---|---|---|---|---|---|---|---|
boolean = | NO | NO | NO | NO | NO | NO | NO | |
char = | NO | NO | NO | NO | NO | NO | NO | |
byte = | NO | NO | NO | NO | NO | NO | NO | |
short = | NO | NO | YES | NO | NO | NO | NO | |
int = | NO | YES | YES | YES | NO | NO | NO | |
long = | NO | YES | YES | YES | YES | NO | NO | |
float = | NO | YES | YES | YES | YES | YES | NO | |
double = | NO | YES | YES | YES | YES | YES | YES |
Casting Incompatible Types Top
The above table isn't the end of the story though as Java allows us to cast incompatible types. A cast instructs the compiler to convert one type to another enforcing an explicit type conversion.
A cast takes the form target = (target-type) expression.
There are a couple of things to consider when casting incompatible types:
- With narrowing conversions such as an
int
to ashort
there may be a loss of precision if the range of theint
exceeds the range of ashort
as the high order bits will be removed. - When casting a floating-point type to an integer type the fractional component is lost through truncation.
- The target-type can be the same type as the target or a narrowing conversion type.
- The
boolean
type is not only incompatible but also inconvertible with other types.
Lets look at some code to see how casting works and the affect it has on values:
package info.java8;
/*
Casting Incompatible Types
*/
public class Casting {
public static void main (String[] args) {
char a = 'D';
short b = 129;
int c = 4127;
long d = 33445566L;
float e = 12.34F;
double f = 456.789;
byte g = (byte) a;
System.out.println(a + " Cast char to byte: " + g);
g = (byte) b;
System.out.println(b + " Cast short to byte: " + g);
g = (byte) c;
System.out.println(c + " Cast int to byte: " + g);
g = (byte) d;
System.out.println(d + " Cast long to byte: " + g);
g = (byte) e;
System.out.println(e + " Cast float to byte: " + g);
g = (byte) f;
System.out.println(e + " Cast double to byte: " + g);
}
}
Running the Casting
class produces the following output:
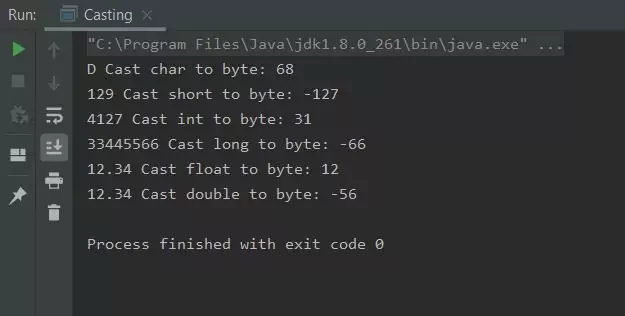
Casting
class.The first thing to note is we got a clean compile because of the casts, all the type conversions would fail otherwise. You might be suprised by some of the results shown in the screenshot above, for instance some of the
values have become negative. Because we are truncating everything to a byte we are losing not only any fractional components and bits outside the range of a byte
, but in some cases the signed bit as well. Casting
can be very useful but just be aware of the implications to values when you enforce explicit type conversion.
Related Quiz
Fundamentals Quiz 8 - Assignment Operators Quiz
Lesson 9 Complete
In this lesson we looked at the assignment operators used in Java.
What's Next?
In the next lesson we look at the bitwise logical operators used in Java.