Bitwise Logical OperatorsJ8 Home « Bitwise Logical Operators
In the fourth lesson on operators we look at the bitwise logical operators. Bitwise operators perform their operations on the integer types byte
, short
, int
and
long
and will not work with any other type. These operators are used to manipulate the bits within an integer value, hence the name.
- Bits with the value
0
are said to be switched off. - Bits with the value
1
are said to be switched on. - All bitwise conversions get promoted to the
int
type before conversion so you need to cast back toshort
andbyte
when using these types for the target.
Bitwise Logical Operators Overview Top
The bitwise logical operators perform the same operations as the logical operators discussed in the last lesson but work on a bit-by-bit basis on the integer type. The following table shows all possible combinations for a bit using 0
and 1
.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
& | AND | bit a = 0 and bit b = 0 a & b bit a = 0 and bit b = 1 a & b bit a = 1 and bit b = 0 a & b bit a = 1 and bit b = 1 a & b |
0 0 0 1 | Will turn the result bit to 0 unless both bits are 1 .Useful for switching bits off. |
| | OR | bit a = 0 and bit b = 0 a | b bit a = 0 and bit b = 1 a | b bit a = 1 and bit b = 0 a | b bit a = 1 and bit b = 1 a | b |
0 1 1 1 | Will turn the result bit to 1 if either bits are 1 .Useful for switching bits on. |
^ | XOR (exclusive OR ) | bit a = 0 and bit b = 0 a ^ b bit a = 0 and bit b = 1 a ^ b bit a = 1 and bit b = 0 a ^ b bit a = 1 and bit b = 1 a ^ b |
0 1 1 0 | Will switch the result bit to 1 if only one of the bits is 1 otherwise the result bit is switched to 0.Useful for highlighting unmatched bits. |
~ | NOT | bit a = 0 ~a bit a = 1 ~a |
1 0 | Useful for switching bits to show the compliment of the number. |
Let's look at each of the bitwise logical operators in turn to get a feel for how they work.
Bitwise AND Top
The bitwise AND operator can be used for turning non-matched bits off. Lets look at some code to illustrate how this works:
package info.java8;
/*
Bitwise AND
*/
public class BitwiseAnd {
public static void main (String[] args) {
byte a = 97;
printBits(a);
byte b = 57;
printBits(b);
byte c = (byte) (a & b);
printBits(c);
}
/*
Loop through input parameter and print out the bits
*/
public static void printBits (byte aByte) {
System.out.print("Input param aByte = " + aByte + ": ");
for (int i = 128; i > 0; i /= 2) {
if ((aByte & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseAnd
class produces the following output:
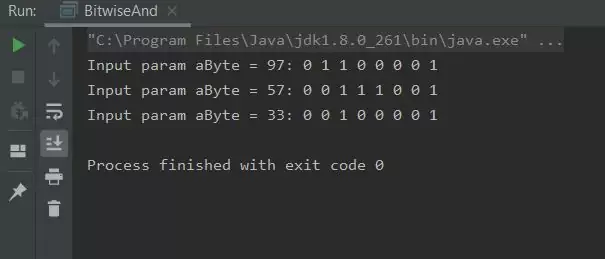
BitwiseAnd
class.Ok there are several new things going on with the above piece of code that we will go through. The first thing to notice is we are casting to a byte when using the bitwise AND (byte c = (byte) (a & b);
). All
bitwise conversions get promoted to the int
type before conversion so we need to cast back to the byte
type. Also we are enclosing the bitwise operation in parentheses so the whole expression gets
evaluated and not just the a
. We are using the printBits
method to save replication of code. We pass each variable to this method and print the value. We then use a for
loop to print out
each bit of the byte variable passed to the method. We will go through the for
loop in the Loop Statements lesson but what we are doing here is just dividing 256 by 2 to get to each bit. The 8th bit is used for the sign
(0 = positive, 1 negative), hence the actual value range of a byte is 127
to -128
as stated in the Primitive Variables lesson. As you can see from the output the
bitwise AND operator switches non-matched bits off.
Bitwise OR Top
The bitwise OR operator can be used for turning non-matched bits on. Lets look at some code to illustrate how this works:
package info.java8;
/*
Bitwise OR
*/
public class BitwiseOr {
public static void main (String[] args) {
byte a = 97;
printBits(a);
byte b = 57;
printBits(b);
byte c = (byte) (a | b);
printBits(c);
}
/*
Loop through input parameter and print out the bits
*/
public static void printBits (byte aByte) {
System.out.print("Input param aByte = " + aByte + ": ");
for (int i = 128; i > 0; i /= 2) {
if ((aByte & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseOr
class produces the following output:
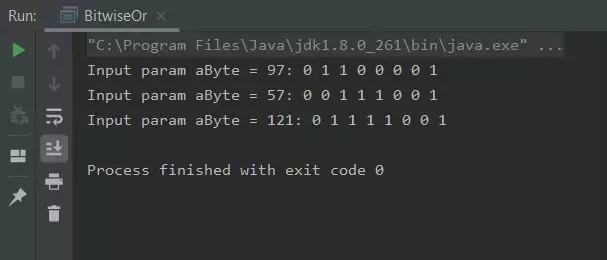
BitwiseOr
class.As you can see from the output the bitwise OR operator switches non-matched bits on.
Bitwise XOR Top
The bitwise XOR operator can be used for highlighting unmatched bits as any matching bits will be switched to 0
, whilst unmatched bits are switched to 1
. Lets look at some code to illustrate how this works:
package info.java8;
/*
Bitwise XOR
*/
public class BitwiseXor {
public static void main (String[] args) {
byte a = 97;
printBits(a);
byte b = 57;
printBits(b);
byte c = (byte) (a ^ b);
printBits(c);
}
/*
Loop through input parameter and print out the bits
*/
public static void printBits (byte aByte) {
System.out.print("Input param aByte = " + aByte + ": ");
for (int i = 128; i > 0; i /= 2) {
if ((aByte & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseXor
class produces the following output:
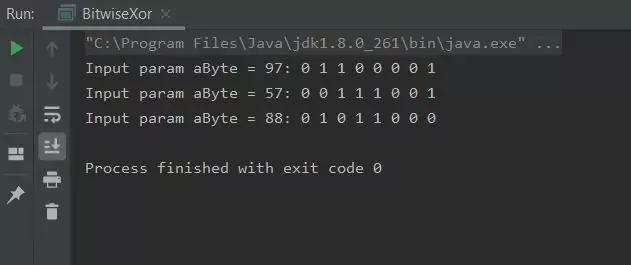
BitwiseXor
class.As you can see from the output the bitwise XOR operator switches non-matched bits on and matched bits off.
Bitwise NOT Top
The bitwise NOT operator can be used for switching bits to show the compliment of the number. Lets look at some code to illustrate how this works:
package info.java8;
/*
Bitwise NOT
*/
public class BitwiseNot {
public static void main (String[] args) {
byte a = 57;
printBits(a);
a = (byte)~a;
printBits(a);
}
/*
Loop through input parameter and print out the bits
*/
public static void printBits (byte aByte) {
System.out.print("Input param aByte = " + aByte + ": ");
for (int i = 128; i > 0; i /= 2) {
if ((aByte & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseNot
class produces the following output:
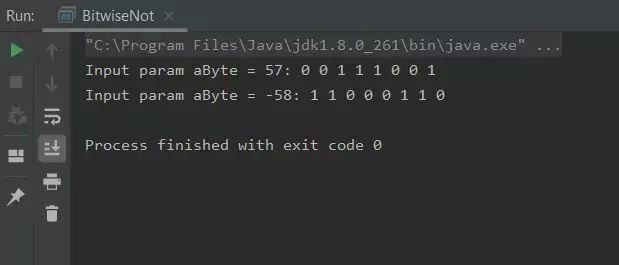
BitwiseNot
class.As you can see from the output the bitwise NOT operator switches bits to show the compliment of the number.
Bitwise Shorthand Assignment Operators Top
The bitwise shorthand assignment operators allow us to write compact code that is implemented more efficiently. When using the bitwise shorthand assignment operators there is no need to cast either.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
&= | Bitwise AND | byte a = 74; | 72 | Will give the Bitwise AND result of 74 and 124. |
|= | Bitwise OR | byte a = 74; | 126 | Will give the Bitwise OR result of 74 and 124. |
^= | Bitwise XOR | byte a = 74; | 54 | Will give the Bitwise XOR result of 74 and 124. |
Related Quiz
Fundamentals Quiz 9 - Bitwise Logical Operators Quiz
Lesson 10 Complete
In this lesson we took a fourth look at the symbols used in Java for mathematical and logical manipulation by looking at bitwise logical operators.
What's Next?
In the next lesson we take our final look at operators when we investigate bitwise shift operators.