Bitwise Shift OperatorsJ8 Home « Bitwise Shift Operators
In our final lesson on operators we look at the bitwise shift operators. Bitwise operators perform their operations on the integer types byte
, short
, int
and
long
and will not work with any other type. These operators are used to manipulate the bits within an integer value, hence the name.
- Bits with the value
0
are said to be switched off. - Bits with the value
1
are said to be switched on. - All bitwise conversions get promoted to the
int
type before conversion so you need to cast back toshort
andbyte
when using these types for the target.
Bitwise Shift Operators Overview Top
The bitwise shift operators allow us to shift the bits that make up an integer type to the left or right by a specified amount.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
<< | Left shift | int a = 1234; | 4936 | Will shift the value by the specified number of bits to the left. value << numberOfBits. |
>> | Right shift | int a = 1234; | 154 | Will shift the value by the specified number of bits to the right. value >> numberOfBits. |
>>> | Signed right shift | int a = -12345; | -772 | Will shift the value by the specified number of bits to the right, whilst retaining the signed bit. If the value is negative then the resultant moved bits will be sign-extended. value >>> numberOfBits. |
Let's look at each of the bitwise shift operators in turn to see how we got the values in the table above.
Bitwise Left Shift Top
The bitwise left shift operator allows us to shift the bits in an integer type to the left by the specified amount.
package info.java8;
/*
Bitwise Left Shift
*/
public class BitwiseLeftShift {
public static void main (String[] args) {
short a = 1234;
printBits(a);
a = (short) (a << 2);
printBits(a);
}
/*
Use a loop to print out the bits
*/
public static void printBits (short a) {
System.out.print("Input param a = " + a + ": ");
for (int i = 32768 ; i > 0; i /= 2) {
if (i == 128) {
System.out.print(" | ");
}
if ((a & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseLeftShift
class produces the following output:
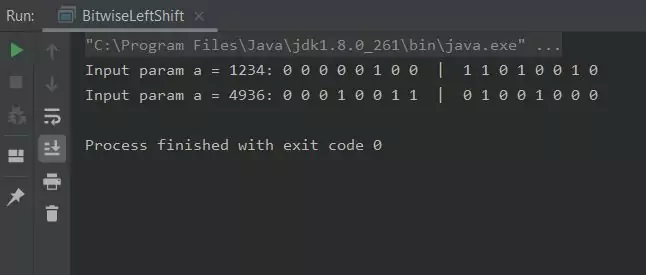
BitwiseLeftShift
class.As you can see from the output all the bits have been shifted 2 places to the left giving us a new value. We are using the |
symbol to split the result into 8-bit chunks.
Bitwise Right Shift Top
The bitwise right shift operator allows us to shift the bits in an integer type to the right by the specified amount.
package info.java8;
/*
Bitwise Right Shift
*/
public class BitwiseRightShift {
public static void main (String[] args) {
short a = 1234;
printBits(a);
a = (short) (a >> 3);
printBits(a);
}
/*
Use a loop to print out the bits
*/
public static void printBits (short a) {
System.out.print("Input param a = " + a + ": ");
for (int i = 32768 ; i > 0; i /= 2) {
if (i == 128) {
System.out.print(" | ");
}
if ((a & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseRightShift
class produces the following output:
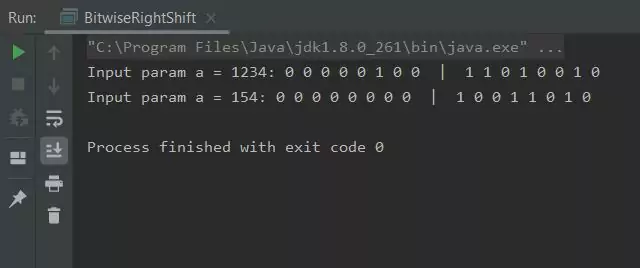
BitwiseRightShift
class.As the output shows all the bits have been shifted 3 places to the right giving us a new value. We are using the |
symbol to split the result into 8-bit chunks.
Bitwise Signed Right Shift Top
The bitwise signed right shift operator allows us to shift the bits in an integer type to the right by the specified amount, whilst retaining the signed bit.
package info.java8;
/*
Bitwise Signed Right Shift
*/
public class BitwiseSignedRightShift {
public static void main (String[] args) {
short a = -12345;
printBits(a);
a = (short) (a >>> 4);
printBits(a);
}
/*
Use a loop to print out the bits
*/
public static void printBits (short a) {
System.out.print("Input param a = " + a + ": ");
for (int i = 32768 ; i > 0; i /= 2) {
if (i == 128) {
System.out.print(" | ");
}
if ((a & i) != 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println(" ");
}
}
Running the BitwiseSignedRightShift
class produces the following output:
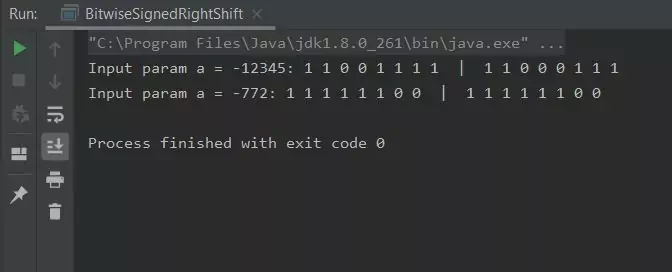
BitwiseSignedRightShift
class.As the output shows all the bits have been shifted 3 places to the right giving us a new value. We are using the |
symbol to split the result into 8-bit chunks.
Did you spot anything else? When you right shift a negative number, when the interim expression is promoted to an int
from a short
or byte
all the extra bits are sign-extended.
Thus when we cast back to a short
in our example all bits shifted in after our signed bit are 1
and we get an unexpected result. Be aware of this when using this particular bitwise operator.
Bitwise Shorthand Assignment Operators Top
The bitwise shorthand assignment operators allow us to write compact code that is implemented more efficiently. When using the bitwise shorthand assignment operators there is no need to cast either.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
<<= | Left shift | short a = 1234; | 4936 | Left shift 1234 by 2 bits. |
>>= | Right shift | short a = 1234; | 154 | Right shift 1234 by 3 bits. |
>>>= | Signed right shift | short a = -12345; | -772 | Signed right shift -12345 by 4 bits. |
Operator Precedence Top
We will finish our discussion on operators with a table showing the order of precedence that is invoked when Java interprets operator symbols. The list includes the special operators which will be discussed in later lessons. When using multiple operators in an expression it is always best practice to use parentheses to explicitly state the order of precedence for clarity and readability. The table lists order of precedence from highest to lowest.
Precedence | Operators | Operation | Associativity |
---|---|---|---|
1 | () | method call | left |
[ ] | array index | ||
. | method access | ||
2 | + | unary plus | right |
- | unary minus | ||
++ | prefix/postfix increment | ||
-- | prefix/postfix decrement | ||
! | boolean logical NOT | ||
~ | bitwise NOT | ||
(type) | type cast | ||
new | object creation | ||
3 | / | division | left |
* | mutiplication | ||
% | modulus | ||
4 | + | addition or string concatenation | left |
- | subtraction | ||
5 | << | bitwise left shift | left |
>> | bitwise right shift | ||
>>> | bitwise signed right shift | ||
6 | / | less than | left |
<= | less than or equal to | ||
> | greater than | ||
>= | greater than or equal to | ||
instanceof | reference test | ||
7 | == | equal to | left |
!= | not equal to | ||
8 | & | boolean logical AND or bitwise AND | left |
9 | ^ | boolean logical XOR or bitwise XOR | left |
10 | | | boolean logical OR or bitwise OR | left |
11 | && | short-circuit boolean logical AND | left |
12 | || | short-circuit boolean logical OR | left |
13 | ? : | conditional | right |
14 | = | assignment | right |
+= | shorthand addition | ||
-= | shorthand subtraction | ||
/= | shorthand division | ||
*= | shorthand mutiplication | ||
%= | shorthand modulus | ||
&= | shorthand boolean logical AND or bitwise AND | ||
|= | shorthand boolean logical OR or bitwise OR | ||
^= | shorthand boolean logical XOR or bitwise XOR | ||
<<= | shorthand bitwise left shift | ||
>>= | shorthand bitwise right shift | ||
>>>= | shorthand bitwise signed right shift |
Related Quiz
Fundamentals Quiz 10 - Bitwise Shift Operators Quiz
Lesson 11 Complete
In this lesson we took a final look at the symbols used in Java for mathematical and logical manipulation by looking at bitwise shift operators.
What's Next?
In the next lesson we take our first look at conditional statements when we look at the if
construct.