Handling ExceptionsJ8 Home « Handling Exceptions
Lets take a look at the points outlined at Oracle Java SE 8 Programmer I for this part of the certification.
- Handling Exceptions
- Differentiate among checked exceptions, unchecked exceptions, and Errors.
- Create a try-catch block and determine how exceptions alter normal program flow.
- Describe the advantages of Exception handling.
- Create and invoke a method that throws an exception.
- Recognize common exception classes (such as NullPointerException, ArithmeticException, ArrayIndexOutOfBoundsException, ClassCastException).
The table below breaks the above list down into topics with a link to the topic in question.
topic | Link |
---|---|
Differentiate among checked exceptions, unchecked exceptions, and Errors. | Exception Overview |
Create a try-catch block and determine how exceptions alter normal program flow. | try catch Construct |
Describe the advantages of Exception handling. | |
Create and invoke a method that throws an exception. | Using the throws Keyword |
Recognize common exception classes (such as NullPointerException, ArithmeticException, ArrayIndexOutOfBoundsException, ClassCastException). | Certification Exceptions |
Exception OverviewTop
An exception refers to an exceptional condition that has occurred to alter the normal flow of our programs. When an exception occurs it will get thrown by the JVM or we can determine conditions to throw an exception ourselves when required. We can catch thrown exceptions and the code we write for this purpose is known as an exception handler. We can also declare exceptions in our method definitions, when we know for instance that the method will try to read a file that may or may not be present.
At the top of the exception hierarchy is the Throwable
class, which all other error and exception classes inherit from. The Throwable
class creates a snapshot of the stack trace for exceptions
when they occur and may also contain information about the exception. We can use this information for debugging purposes. Only instances of the Throwable
class, or subclasses of it, can be
thrown by the JVM or be thrown by us.
Two subclasses inherit from the Throwable
class and these are the Error
and Exception
classes. These classes, and subclasses
thereof, are used to create an instance of the appropriate error or exception along with information about the exception:
- The
Error
class and its subclasses handle error situations from within the JVM itself and so are outside our control as programmers. As a general rule we can't recover from anError
situation and because of this we are not expected to handle anError
when it occurs. - The
Exception
class and its subclasses are what concern us more as programmers and these can be split into two categories:- Runtime exceptions: Are exceptions that are not checked for by the compiler and for this reason are also known as unchecked exceptions. Generally exceptions of type
RuntimeException
originate from problems in our code, such as an attempt to divide by zero which gives anArithmeticException
. These sorts of errors are within our control and as such should be handled by the code itself. - Checked exceptions: Are exceptions that are checked for by the compiler and if present and not declared, will give a compiler error. An example of this is a
FileNotFoundException
where a file we want to read may or may not be available. Whether the file is available or not is outside our control. So when we read the file, we need to handle or declare that this exception, or a superclass of it may occur.
- Runtime exceptions: Are exceptions that are not checked for by the compiler and for this reason are also known as unchecked exceptions. Generally exceptions of type
Exception Hierarchy Diagram
The diagram below is a representation of the exception hierarchy and by no means a complete list, but should help in visualisation:
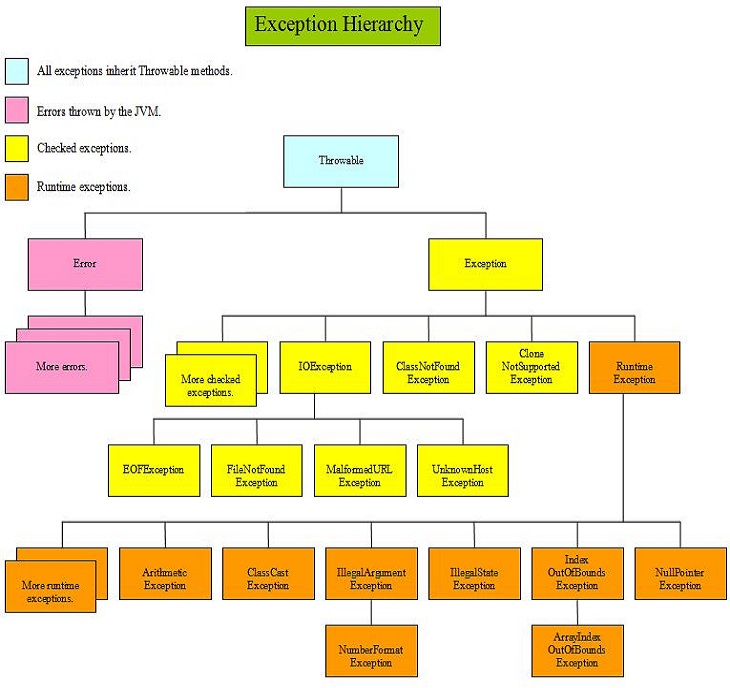
In the diagram above each box represents a class and as you can see the Exception
class, which interests us as programmers, has lots of subclasses attached to it. We are just showing some of them in
the above diagram as space permits, but I am sure you get the idea. When an exception happens an exception object of the appropriate class is created and we can use code to handle the exception.
try catch
ConstructTop
The following table shows the try catch
construct.
Construct | Description |
---|---|
try catch | |
try { |
Execute statements in try code block.Execute statements in catch code block. |
try catch
Rules
When using the try catch
construct there are certain rules that must be adhered to or you get a compiler error:
- When using a
try
block it must be accompanied by acatch
block. - When using a
catch
block it must immediately follow thetry
block.
Using the throws
Keyword Top
The compiler demands that we either handle or declare exceptions which are neither error or runtime exceptions, in other words checked exceptions. We looked at handling exceptions
in the last lesson, now we will look at declaring exceptions using the throws
keyword. To show this we will recode the DivideByZero
class to declare that it throws an
exception of type Exception
.
package info.java8;
/*
A DivideByZero class
*/
public class DivideByZero {
public static void main(String[] args) throws Exception { // Declare checked exception
try {
int a = 5 / 0; // JVM
will not like this
}
/*
We handle the exception in a catch block and rethrow it
*/
catch (ArithmeticException ex) {
System.out.println("We caught exception: " + ex);
throw new Exception(); // Need to handle or declare checked Exception
}
}
}
Save, compile and run the recoded DivideByZero
class in directory c:\_FlowControl in the usual way.
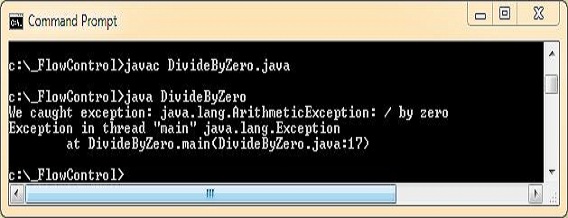
The above screenshot shows the output of compiling and running our reworked DivideByZero
class. This time the code compiles fine as we are declaring the Exception
checked exception
that we rethrow. We catch the ArithmeticException
exception and rethrow it as an Exception
and the exception propogates up the stack and our program ends.
Certification ExceptionsTop
The following table lists some exceptions that are useful to know for the certification:
Error/Exception | Description |
---|---|
ArithmeticException | Thrown when an exceptional arithmetic condition has occurred. An example of this exception is shown in the Declaring Exceptions lesson when we look at Using the throws keyword. |
ArrayIndexOutOfBoundsException | Attempt to access array with an illegal index. An example of this exception is shown in the Arrays lesson when we look at java.lang Array Exceptions. |
ClassCastException | Attempt to cast an object to a subclass of which it is not an instance. An example of this exception is shown in the Generics lesson when we look at a Raw Type/Generic Type Comparison. |
IllegalArgumentException | Method invoked with an illegal argument. |
IllegalStateException | Method invoked while an application isn't in the correct state to receive it. |
NullPointerException | Attempt to access an object with a null reference.An example of this exception is shown in the Reference Variables lesson when we look at The Heap. |
NumberFormatException | Invalid attempt to convert the contents of a string to a numeric format. An example of this exception is shown in the Exception Handling lesson when we look at Using try catch finally . |
Related Java Tutorials
Fundamentals - Conditional Statements
Fundamentals - Loop Statements
Fundamentals - Primitive Variables
Fundamentals - Operators
Flow Control - Using Assertions
Flow Control - Exception Overview
Flow Control - Exception Handling
Flow Control - Declaring Exceptions