Java BasicsJ8 Home « Java Basics
Lets take a look at the points outlined at Oracle Java SE 8 Programmer I for this part of the certification.
- Java Basics
- Define the scope of variables.
- Define the structure of a Java class.
- Create executable Java applications with a main method; run a Java program from the command line; produce console output.
- Import other Java packages to make them accessible in your code.
- Compare and contrast the features and components of Java such as: platform independence, object orientation, encapsulation, etc.
The table below breaks the above list down into topics with a link to the topic in question.
topic | Link |
---|---|
Define the scope of variables. | Variable Scope |
Define the structure of a Java class. | A Look At Java Code Structure |
Create executable Java applications with a main method; run a Java program from the command line; produce console output. | The Java main() Method |
Import other Java packages to make them accessible in your code. | Import Java packages |
Compare and contrast the features and components of Java such as: platform independence, object orientation, encapsulation, etc. | Java Features |
Variable ScopeTop
In Java we can declare variables anywhere within a block of code. A block starts with its opening curly brace and ends with its closing curly brace. A block and where it resides defines the type of the variable and the scope of the enclosed data. Therefore each time you start a new block of code you start a new scope. Scope determines the visibility and lifetime of the variables within it. Java comes with three kinds of scope and we name variables according to the scope they reside in as detailed in the table below.
Variable | Scope | Lifetime |
---|---|---|
static | Static variables apply to the class as a whole and are declared within the class but outside a method. | Exists for as long as the class it belongs to is loaded in the JVM. See the Static Members lesson for more information. |
instance | Instance variables apply to an instance of the class and are declared within the class but outside a method. | Exists for as long as the instance of the class it belongs to. See the Instance Variables & Scope lesson for more information. |
local | Local variables apply to the method they appear in. | Exists until the method it is declared in finishes executing. See the Method Scope lesson for more information. |
A Look At Java Code Structure Top
A Java source file is made up of a class with the code for the class going inside the class definition. The name of the file that we store the source in, which is also known as a compilation unit, should be the same as the class it contains including any capitalisation when the class is public. The reason for this is the Java compiler will use the name of the public class within our program to create the bytecode version of our source file. Before we go into more details of this lets take a look at the structure of a Java source file using a diagram.
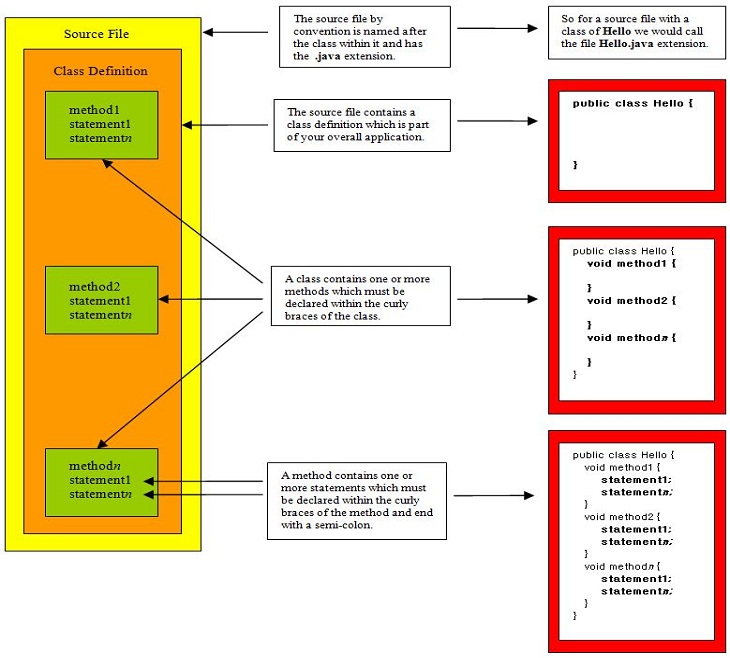
So using the diagram above we can note down a few points about a Java source file:
- If we want to create a class called Hello then by convention and best practice we should save our source file as Hello.java . Always capitalize the first letter of each word used for the class name.
- The
public
keyword preceding the name of the class is an access modifier and in this case means the class can be accessed by anyone.
Only one public class per source file is allowed and if present is what we use as the identifer when we come to compile our source file into bytecode.
If there is no public class present we can call a source file any valid identifier we choose.
An empty source file with nothing in it will also compile without error. - The source file will then contain the
class
keyword followed by the name of the class and a set of curly braces. - We can specify one or more methods within the curly braces of the class, with each method also having a set of curly braces.
- The
void
keyword preceding the name of each method specifies that the method doesn't return any value. This is known as a return type and is mandatory. - We can specify one or more statements within the curly braces of each specified method and each must end with a semi-colon.
There is more to source files than the points raised above but for now this is more than enough to think about.
As a side note Java has a set of coding standards for how to set out your code. Of course with the restrictions of screen sizes the site can't always adhere to these standards. Also in some tests we put more than one statement on a line which is what you will get if you sit the certification. Use the following link Code Conventions for the Java Programming Language to get a copy of coding standards to get into the habit of using them.
The Java main()
Method Top
A Java application is a class or collection of classes that interact with each other to produce the desired results. So how does the application know which class to start off with? Well within our suite of classes one of the classes needs to have a special method called main()
which is used to run the application from the command line. This is achieved by typing the java
command on the command line followed by the name of the class containing the main()
method. Lets take a look at the syntax of the main()
method and see how it fits into a class.
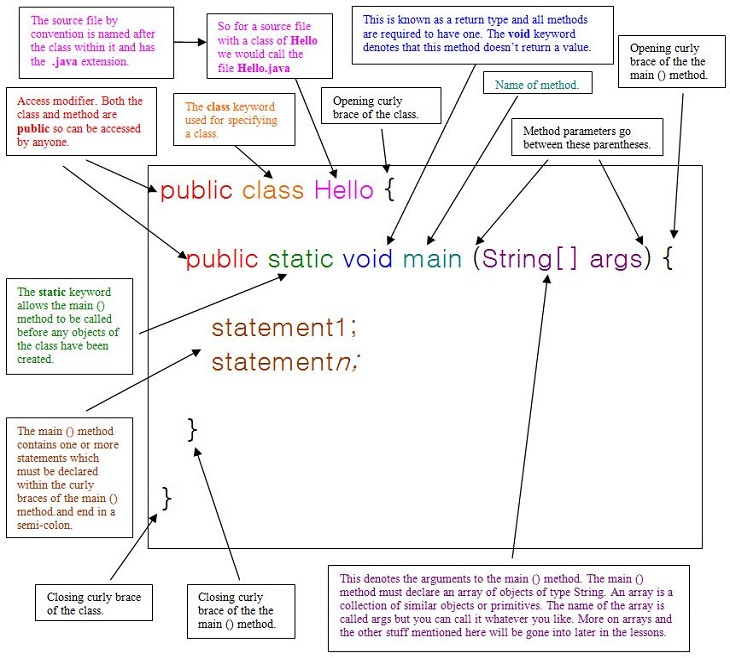
There's a lot of information contained within the diagram above so take your time looking at it and getting a feel for the syntax and how it all hangs together. We will cover things like access modifier, objects and such in later lessons so we don't overload too much at the moment.
Import Java packagesTop
In Java we can keep libraries of related classes together using a package and this is in essence what the Java language is, a collection of packaged libraries.
- If a class is part of a package then the
package
statement must be the first line of code in a source file.
There are two major benefits to the package approach adopted by Java.
- A package provides the apparatus for us to group related classes together under the same umbrella.
- We need a way to uniquely identify classes and Java doesn't allow us to have two top-level classes with the same name, within the same namespace. By using packaging we can partition the namespace to alleviate naming conflicts.
- Within any reasonable sized system we will have classes that relate to different aspects of said system, such as the Model View Controller paradigm. Packaging allows us to separate concerns into areas such as a Model subpackage, a View subpackage and so on. This makes the whole development process easier and more manageable.
- A package is part of the Java mechanism we use to enforce Encapsulation.
- By not marking members within a package with an access modifier, we are saying that these members are package-private and can only be accessed from within this package.
- By marking members with the
protected
access modifier, we are saying that these members can only be accessed from within this package or from a subclass outside the package.
The following table shows usage of a single package
statement and an example of a multiple hierarchy package
statement.
Package Form | Example | Description |
---|---|---|
Single package | ||
package pkg; | package A; | This file is part of package A. |
Multiple package hierarchy | ||
package pkg.subPkg1.subPkg2...subPkgN; | package A.B.C; | This file is part of Package C, which is a subpackage of B, which is a subpackage of A. |
Imports
Java allows us to import parts of a package, or even the whole package if required for use in our code and we do this using the import
keyword.
- If a class uses the
import
keyword to import parts or all of a package, then theimport
keyword must follow thepackage
statement if there is one, or must be the first line of code in a source file if there is nopackage
statement.
The following table shows how to import a single class
from a package and all classes from a package using the import
statement.
Import Form | Example | Description |
---|---|---|
Single class import | ||
import pkg.class; | import java.rmi.Remote; | Import the Remote class from java.rmi |
Multiple class import | ||
import pkg.subPkg1.subPkg2...subPkgN; | import java.rmi.*; | Import all java.rmi classes |
Static Imports
With the introduction of Java 5 a new feature became available when using the import
statement which is commonly known as static imports. When using the import
keyword followed by
static
we can import all the static members of a class or interface.
The following table shows how to import a single static member from a package and all static members from a package using the import static
statement.
Import Static Form | Example | Description |
---|---|---|
Single static member import | ||
import static pkg.staticMember; | import static java.lang.Math.acos; | Import the acos static member from java.lang.Math |
Multiple static member import | ||
import static pkg.allStaticMembers; | import static java.lang.Math.*; | Import all java.lang.Math static members |
Java FeaturesTop
Related Java Tutorials
Fundamentals - Primitive Variables
Fundamentals - Method Scope
Objects & Classes - Arrays
Objects & Classes - Class Structure and Syntax
Objects & Classes - Reference Variables
Objects & Classes - Reference Variables - The new Operator
Objects & Classes - Methods - Overloaded Methods
Objects & Classes - Methods - Overloaded varargs Ambiguities
Objects & Classes - Instance Variables & Scope
Objects & Classes - Constructors
Objects & Classes - Enumerations
OO Concepts - Abstraction - Abstract Classes
OO Concepts - Inheritance - Overriding Methods
OO Concepts - Inheritance Concepts - Accessing Superclass Overrides
Inheritance Concepts -Superclass Constructors
OO Concepts - Interfaces
OO Concepts - Nested Classes
Flow Control - Methods - Overridden Methods & Exceptions
API Contents - Packages
API Contents - Packages - Imports
API Contents - Packages - Static Imports