Nested Static Member ClassesJ8 Home « Nested Static Member Classes
Nested classes are classes defined within the curly braces of other classes. A nested class is only known to the enclosing class and shares its scope. This means that non-static nested classes have access to all the members and variables of the outer class. Conversely the outer class knows nothing of the internal working of the nested class.
There are many benefits to using nested classes and those relevant to the particular nested class are listed within the appropriate lesson. The benefits that all nested classes receive are:
- When a class has a specific purpose that is only relevant to one other class it makes sense to put the helper class within the class that uses it and we can use a nested class for this purpose.
- Because nested classes have access to all the outer classes members, including members with the
private
access modifier, it gives us a way to keep outer class membersprivate
while still being able to access them, thus increasing encapsulation. - Having nested classes allows us to have inner classes close to the top-level classes that enclose them, making code easier to understand and maintain.
Nested classes can be static, which are known as static member classes, or non-static, which are known as inner classes. There are different types of inner class which we will look at in the Nested Inner Classes and Nested Anonymous Inner Classes lessons, but only one type of static member class so let's look at the static version first.
Static Member Classes Top
A static member class is associated with its outer class in the same way that static members of the outer class would be. This means that a static member class cannot refer to non-static variables and methods defined within the outer class and can only interact with them through an object reference.
Behaviourally, static member classes act like any other top-level class and essentially are top-level classes that have been nested in another top-level class to facilitate packaging, or are associated with the outer class but it makes no sense to attach them to an instance of that class.
Static Member Instantiation Top
Following is example code for instantiation of a static member class:
package info.java8;
/*
An example of a static member class
*/
public class EnclosingClass {
EnclosingClass.StaticMemberClass staticMemberObject = new EnclosingClass.StaticMemberClass();
static class StaticMemberClass {
...
}
}
We use the name of the outer class followed by the name of the inner class to instantiate the inner static class.
Static enum
Example Top
The following code uses an enum
as a static member class, we could have different static member entries for lunches and dinners for instance:
package info.java8;
/*
A Recipes Class with a static member enum
*/
public class Recipes {
/*
Static Enumeration of soup (helper class)
*/
public static enum Soup {
TOMATO("vegetable") { // Constant-specific class body
public String starRating() {
return "5 star rated";
}
},
CHICKEN("meat"),
PRAWN("seafood") { // Constant-specific class body
public String starRating() {
return "3 star rated";
}
};
String type;
/*
enum constructor
*/
Soup(String type) {
this.type = type;
}
String getType() {
return this.type;
}
/*
default star rating
*/
String starRating() {
return "not rated yet";
}
}
}
/*
Test Class for Recipes
*/
public class RecipeTest {
public static void main (String[] args) {
for (Recipes.Soup s : Recipes.Soup.values()) {
System.out.println("We have " + s + " soup in our list, which is a "
+ s.getType() + " soup and is " + s.starRating());
}
}
}
Save and compile the Recipes
and RecipeTest
classes and then run the RecipeTest
class in directory c:\_ObjectsAndClasses in the usual way.
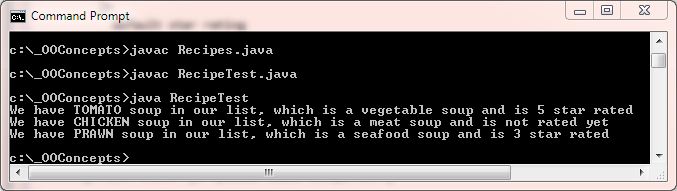
The above screenshot shows the output of running our RecipeTest
class. When an inner class is used as in the scenario above and has no relation to a particular instance of the enclosing class then favour the
use of static member classes as you save space on resources.
Related Quiz
OO Concepts Quiz 16 - Nested Static Member Classes
Lesson 16 Complete
In this lesson we learned about the various ways to code nested static member classes within our classes.
What's Next?
In the next lesson we take a first look at nested inner classes.