Interfaces - Default MethodsJ8 Home « Interfaces - Default Methods
Up until Java8 all interface methods were implicitly declared as public and abstract and had no body, thus providing a contract which an implementing class would have to honour by creating implementations for each method within the implemented interface. This design also meant that the designers of an interface couldn't update it with new methods without breaking the contract of every implementation class that would now have to be refactored to include the new implementations.
The Java designers got around this problem by introducing interface default methods which have a body but do not need to be implemented thus avoiding breaking any existing contracts and refactoring billions of lines of production code.
In this lesson we will discuss interface default methods along with static methods which are also allowed in interfaces from Java8 onwards.
You may be wondering if interfaces are not just the same as abstract classes now as both can contain abstract methods and methods with bodies? Well no for two reasons:
- A class can only extend from one abstract class whereas a class can implement multiple interfaces.
- Abstract classes can create state through instance variables which are not allowed in interfaces.
Using default
With Interfaces Top
Interface default methods are easily recognizable in an interface as they start with the default
keyword and have a body. An example of the benefits of using default methods is the MouseListener
interface which notifies the user when a mouse event occurs. The code below shows the interface in its Java7 incarnation:
public interface MouseListener extends EventListener {
void mouseClicked(MouseEvent e);
void mousePressed(MouseEvent e);
void mouseReleased(MouseEvent e);
void mouseEntered(MouseEvent e);
void mouseExited(MouseEvent e);
}
An implementer of the interface might only be interested in a click event but would have to implement all the other methods in pre Java8 verions of Java.
Following is a Java8 version of the MouseListener
interface using default methods:
package info.java8;
import java.awt.event.MouseEvent;
import java.util.EventListener;
public interface MyMouseListener extends EventListener {
default void mouseClicked(MouseEvent e) {};
default void mousePressed(MouseEvent e) {};
default void mouseReleased(MouseEvent e) {};
default void mouseEntered(MouseEvent e) {};
default void mouseExited(MouseEvent e) {};
}
Any implementer of the interface now only has to implement the click event if that is all they are interested in:
package info.java8;
import java.awt.event.MouseEvent;
public class MyMouseListenerImpl implements MyMouseListener {
public void mouseClicked (MouseEvent e) {
System.out.println("Mouse has been clicked!");
}
}
The following MyMouseListenerImplTest
class tests our implementation of the MyMouseListener
interface:
package info.java8;
public class MyMouseListenerImplTest {
private static Object MouseEvent;
public static void main (String[] args) {
MyMouseListener ml = new MyMouseListenerImpl();
ml.mouseClicked((java.awt.event.MouseEvent) MouseEvent);
}
}
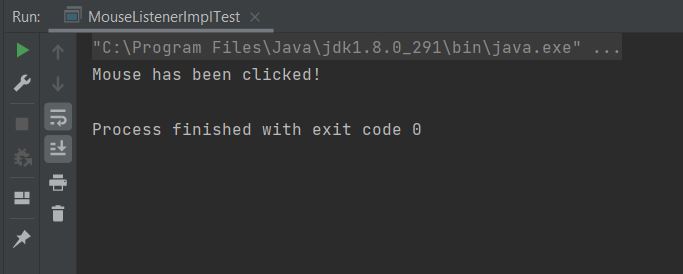
MyMouseListenerImplTest
class implementation.The above screenshot shows the output of running our MyMouseListenerImplTest
test class. This is a lot easier than having to code methods to implement all the methods and reduces code bloat.
Using static
With Interfaces Top
From Java8 it is acceptable to use static methods with implementation code within interfaces. Although this was always technically possibly it was considered an anti-pattern and so pre Java8 interfaces were often accompanied by a companion utility class containing static methods.
A prime example of this is the Collection
interface which is accompanined by the Collections
static utility class.
From Java8 there is no need for these utility classes as the static methods along with their implementation code can be directly written in the interface.
Due to backwards compatibility is is unliknely that classes like Collections
will ever be refactored into the interface they support but when writing your own interfaces there is no longer a reason to provide a
companion static utility class.
Related Quiz
OO Concepts Quiz 11 - Interfaces - Default Methods
Lesson 11 Complete
In this lesson we looked at default and static interface methods which were introduced in Java8.
What's Next?
In the next lesson we delve deeper into polymorphism.