Using Loop ConstructsJ8 Home « Using Loop Constructs
In this lesson we look at the different syntax we can use to affect the flow of logic through our programs. We start by looking at code to implement if
and switch
constructs whilst identifying legal argument types for them. We then investigate the different types of loops available in
java and the iterators and their usage within a loop. We will also look at how we can break from a loop, continue from within a loop and label a loop to continue execution from. We continue our study of flow
control by looking at assertions and when, and when not, they should be used. We finish our certification lessons on flow control by looking at exceptions, handling exceptions and how to declare methods and
overriding methods that throw exceptions. We also need to understand what happens to our code when an error or any type of exception occurs in a code fragment and what happens when particular exceptions occur.
Lets take a look at the points outlined at Oracle Java SE 8 Programmer I for this part of the certification.
- Using Loop Constructs
- Create and use while loops.
- Create and use for loops including the enhanced for loop.
- Create and use do/while loops.
- Compare loop constructs.
- Use break and continue.
The table below breaks the above list down into topics with a link to the topic in question.
topic | Link |
---|---|
Create and use while loops. | The while Construct |
Create and use do/while loops. | The do while Construct |
Use break and continue. | Using while Construct with break StatementUsing while Construct with continue Statement |
Create and use for loops including the enhanced for loop. | The for ConstructThe enhanced for Construct |
Use break and continue. | Using for Construct with break StatementUsing for Construct with continue Statement |
Compare loop constructs. |
The while
ConstructTop
The while
Construct can be used to loop through a section of code while a condition remains true
. The while
Construct has two different variations known commonly as the while loop and the
do-while loop.
The following table shows the different forms of the while
construct that can be used. We are using blocks of code to wrap statements which is optional when using a single statement, but good practice and will be used here.
Construct | Description |
---|---|
while loop | |
while (condition) { | The condition can be any expression that results in a boolean and the loop will continue while the expression
remains true , processing the statement body on each pass.When the condition returns false the loop ends and control is passed to the next line following the
loop.Therefore if the condition starts as false the loop will never be entered. |
do while loop | |
do { | Unlike the normal while loop the statement body is processed before the condition
is tested. The condition can be any expression that results in a boolean and the loop will continue while the expression remains true .When the condition returns
false the loop ends and control is passed to the next line following the loop.Therefore even if the condition starts as false the loop will always execute at least once. |
while
Rules and Examples
There are a few rules to remember when coding the while
Construct:
- The expression we check against, in the parentheses, must evaluate to a
boolean
type:true
orfalse
. - When coding the
while
Construct remember that the loop will never be executed if the expression we check against, in the parentheses resolves to false on loop entry.
Following are some examples showing while
and do while
Construct usage:
/*
Some code showing while and do while statement usage
*/
boolean b = true;
int i = 2;
// while
while (b) {
System.out.println("This is executed");
break;
}
while (i == 2) {
System.out.println("This is executed");
break;
}
while (i) { // Will not compile, does not evaluate to boolean
System.out.println(" ");
}
while (i = 2) { // Assignment not evaluation, so won't compile
System.out.println(" ");
}
while (true) { // This is fine
System.out.println("This is executed");
break;
}
while (true) { // This is fine but loops endlessly
System.out.println("This is executed");
}
// do while
do {
System.out.println("This is executed once");
} while (false);
do {
System.out.println("This is executed once");
b = false;
} while (b);
The do while
ConstructTop
The while
Construct can be used to loop through a section of code while a condition remains true
. The while
Construct has two different variations known commonly as the while loop and the
do-while loop.
The following table shows the different forms of the while
construct that can be used. We are using blocks of code to wrap statements which is optional when using a single statement, but good practice and will be used here.
Construct | Description |
---|---|
while loop | |
while (condition) { | The condition can be any expression that results in a boolean and the loop will continue while the expression
remains true , processing the statement body on each pass.When the condition returns false the loop ends and control is passed to the next line following the
loop.Therefore if the condition starts as false the loop will never be entered. |
do while loop | |
do { | Unlike the normal while loop the statement body is processed before the condition
is tested. The condition can be any expression that results in a boolean and the loop will continue while the expression remains true .When the condition returns
false the loop ends and control is passed to the next line following the loop.Therefore even if the condition starts as false the loop will always execute at least once. |
do while
Rules and Examples
There are a few rules to remember when coding the while
and do while
Constructs:
- The expression we check against, in the parentheses, must evaluate to a
boolean
type:true
orfalse
. - When coding the
while
Construct remember that the loop will never be executed if the expression we check against, in the parentheses resolves to false on loop entry. - When coding the
do while
Construct remember that the loop will always be executed at least once, regardless of whether the expression we check against, in the parentheses resolves to false on loop entry.
Following are some examples showing while
and do while
Construct usage:
/*
Some code showing while and do while statement usage
*/
boolean b = true;
int i = 2;
// while
while (b) {
System.out.println("This is executed");
break;
}
while (i == 2) {
System.out.println("This is executed");
break;
}
while (i) { // Will not compile, does not evaluate to boolean
System.out.println(" ");
}
while (i = 2) { // Assignment not evaluation, so won't compile
System.out.println(" ");
}
while (true) { // This is fine
System.out.println("This is executed");
break;
}
while (true) { // This is fine but loops endlessly
System.out.println("This is executed");
}
// do while
do {
System.out.println("This is executed once");
} while (false);
do {
System.out.println("This is executed once");
b = false;
} while (b);
Example usage of the different forms of the while statement is shown in the Fundamentals - Loop Statements lesson.
Using while
Construct with break
StatementTop
For a while
construct, the break
statement will exit the current loop immediately. Lets look at an example of this:
package info.java8;
/*
while Construct
*/
public class UsingWhile {
public static void main (String[] args) {
int i = 1;
while (i < 13) {
System.out.println("The square of " + i + " = " + i*i);
i++;
}
i = 1;
while (i < 13) {
// Using a break to exit loop
if (i == 6) { break; }
System.out.println("The square of " + i + " = " + i*i);
i++;
}
}
}
Running the UsingAParameter
class produces the following output:
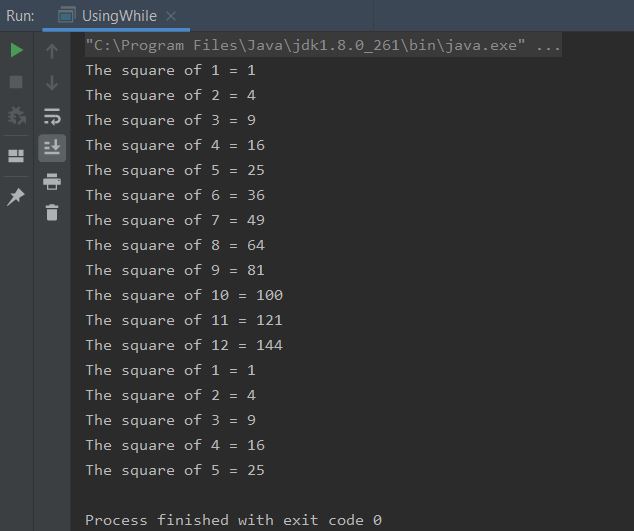
In the first loop what we are doing here is using the control variable (i) and squaring it for the output while it is less than 13. We increment the control variable by 1 just before exiting the while
loop
each time. In the second loop we break
from the loop when the control variable (i) reaches 6. The break
statement forces an exit from the loop immediately.
Using while
Construct with continue
StatementTop
For a while
construct, the continue
statement will pass control back to the start of the loop immediately. Lets look at an example of this:
package info.java8;
/*
Using continue in a while Construct
*/
public class UsingWhileContinue {
public static void main (String[] args) {
int i = 1;
while (i < 13) {
// Using a continue to force an iteration
if ((i%2) == 0) { i++; continue; }
System.out.println("The square of " + i + " = " + i*i);
i++;
}
}
}
Running the UsingAParameter
class produces the following output:
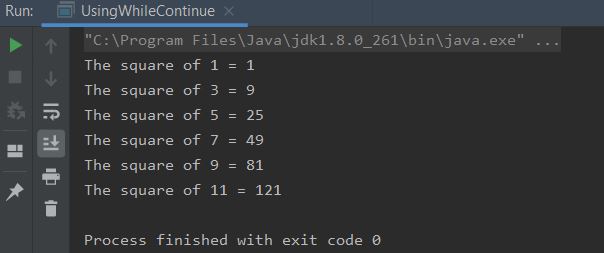
In this variation we are checking to see if the control variable (i) leaves no remainder. If this is the case we are forcing a continue
to the next iteration. For this example it means we are only printing out odd
squares within the specified range. If you notice when we hit this condition we have to increment our control variable before passing control back to the top of the loop. Unlike the for
construct, the while
has no auto increment and hence if we do not increment, we end up in an infinite loop situation.
The for
ConstructTop
The for
Construct will loop through a section of code a set number of times. The for
Construct is very versatile and has two different variations known commonly as the for loop and the
enchanced for loop.
The for loop contains three parts. In the first part we initialize a counter variable with a value, this only happens on initial entry to the for loop. The second part is a condition
which tests the variable value at the start of each loop and if the condition is no longer true
the for loop is exited. The final part of the for
Construct is an expression to be
evaluated at the end of each iteration of the loop. This normally takes the form of a counter that is decremented or incremented.
The enchanced for loop was introduced in java and implements a for-each style loop that iterates through a collection of objects in a sequential order from start to finish.
The following table shows the different forms of the for
construct that can be used. We are using blocks of code to wrap statements which is optional when using a single statement, but good practice and will be used here.
Construct | Description |
---|---|
for loop | |
for ([initialization]; [condition]; [iteration]) { | The initialization , condition , iteration and
statement body components of a for Construct are all optional.The following will create an infinite for loop :for (;;) {...} // Infinite loop.The following will create a for loop with no body:for (int i=1; i<5; i++); // No bodyThe initialization component is generally an assignment statement that
sets the initial value of a control variable used to control iteration of the loop.The condition component is a conditional expression that is always tested againt the control variable
for true before each iteration of the loop. So if this is false to begin with then any statement body component will never be executed.The iteration component
is an expression that determines the amount the control variable is changed for each loop iteration.The statement body is executed each time the condition component
returns true when tested againt the control variable. |
enhanced for loop | |
for (declaration : expression) { | The declaration component declares a variable of a type compatible with the collection to be accessed which will hold a value the same as the current element within the collection.The expression component can be the result of a method call or an expression that evalutes to a collection type.The statement body is executed each time an element of the collection is iterated over. |
Example usage of the different forms of the for statement is shown in the Fundamentals - Loop Statements lesson.
The enhanced for
ConstructTop
The enhanced for
construct will loop through each element within a collection. Lets look at some code to illustrate how this works:
package info.java8;
/*
enhanced for Construct
*/
public class EnhancedFor {
public static void main (String[] args) {
int [] anArray = {22,33,44,55};
for (int i : anArray) {
System.out.println("Array element = " + i);
}
}
}
Running the UsingAParameter
class produces the following output:
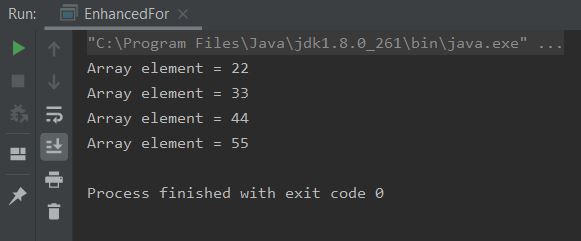
What we are doing here is creating an array collection of numbers; we will expand on arrays in the Arrays lesson. We then use the enhanced for
construct to iterate over the array collection.
Using for
Construct with break
StatementTop
We can also use the break
statement within any loop construct. For a for
construct, the break
statement will exit the loop. Lets look at an example of this:
package info.java8;
/*
Using break in a for Construct
*/
public class UsingForBreak {
public static void main (String[] args) {
for (int i=1; i<11; i++) {
System.out.println("\nThe " + i + " times table: ");
for (int j=1; j<11; j++) {
// Using a break to exit inner loop
if (j == 4) { break; }
System.out.print(j + "*" + i + "=" + j*i + ": ");
}
}
}
Running the UsingAParameter
class produces the following output:

What we are doing here is running a loop within a loop to print off times tables up to 10. So the inner for
loop runs 10 times for each outer for
loop iteration. In the second example we break from the
inner loop during the fourth iteration. This is just to show that using the break
statement forces an exit from the loop you are in not any outer loops.
Using for
Construct with continue
StatementTop
We can also use the continue
statement within any loop construct. For a for
construct, the continue
statement will stop this iteration of the loop and continue at the start of the next iteration. Lets look at an example of this:
package info.java8;
/*
Using continue in a for Construct
*/
public class UsingForContinue {
public static void main (String[] args) {
for (int i=1; i<13; i++) {
// Using a continue to force an iteration
if ((i%2) == 0) { continue; }
System.out.println("The square of " + i + " = " + i*i);
}
}
}
Running the UsingAParameter
class produces the following output:
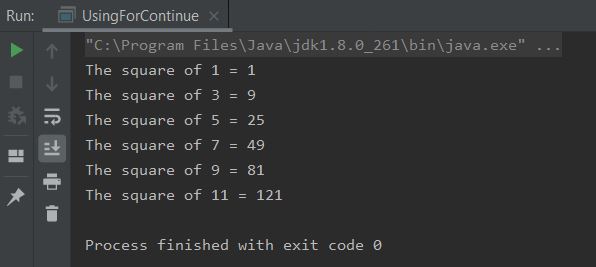
In this variation we are checking to see if the control variable (i) leaves no remainder. If this is the case we are forcing a continue
to the next iteration. For this example it means we are only printing out odd
squares within the specified range.
Related Java Tutorials
Fundamentals - Conditional Statements
Fundamentals - Loop Statements
Fundamentals - Primitive Variables
Fundamentals - Operators
Flow Control - Using Assertions
Flow Control - Exception Overview
Flow Control - Exception Handling
Flow Control - Declaring Exceptions