View Part 1 - StartupJ8 Home « View Part 1 - Startup
In the third lesson of the View Part 1 section of the case study we code the Manufacturer application startup class.
Creating The ManufacturerApplicationStartup
Class Top
The ManufacturerApplicationStartup
class initiates a log for the whole application, verifies the "look and feel" of the Manufacturer application and validates the run mode entered by the user.
Create the ManufacturerApplicationStartup
class in the client
package and cut and paste the following code into it.
package client;
import java.awt.Dimension;
import java.awt.Toolkit;
import javax.swing.*;
import java.io.IOException;
import java.util.logging.*;
/**
* The Manufacturer Application startup that initiates a log for the whole application,
* verifies the "look and feel" of the application and validates the run mode entered
* by the user.
*
* @author Charlie
* @version 1.0
*
*/
public class ManufacturerApplicationStartup {
/**
* The Logger instance through which all log messages from this class are
* routed. Logger namespace is J8CaseStudy
.
*/
private static Logger log = Logger.getLogger("J8CaseStudy"); // Log output
/**
* The main method to startup the Manufacturer Application.
*
* @param args The mode the user wishes to start the application in.
*
*/
public static void main(String[] args) {
new ManufacturerApplicationStartup(args);
}
/**
* Sets up a logger for the whole application, the default Swing look
* and feel and then passes control to either the ServerStartupWindow
* class or the ManufacturerWindow class dependent on command line arguments
* entered by the user.
*
* @param args The mode the user wishes to start the application in.
*
* "" Start application in networked client mode.
* "client" Start application in non-networked client mode.
* "server" Start application in server mode.
*/
public ManufacturerApplicationStartup(String[] args) {
/*
* Setup a rotating file in default user directory for machine that will
* handle logs for the whole application
*/
try {
FileHandler manufacturerFileHandler =
new FileHandler("D:\\ManufacturerApplication\\J8CaseStudy%g.log", 0, 10);
manufacturerFileHandler.setFormatter(new SimpleFormatter());
Logger log = Logger.getLogger("J8CaseStudy");
log.addHandler(manufacturerFileHandler);
log.setLevel(Level.FINEST);
} catch (IOException e) {
log.log(Level.SEVERE, e.getMessage(), e);
}
log.entering("ManufacturerApplicationStartup", "ManufacturerApplicationStartup", args);
// Try to set default Swing "look and feel" for this machine
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (UnsupportedLookAndFeelException | ClassNotFoundException |
InstantiationException | IllegalAccessException ex) {
log.log(Level.SEVERE, ex.getMessage(), ex);
}
if (args.length == 0 || "client".equalsIgnoreCase(args[0])) {
// Create an instance of the Manufacturer application window
// Uncomment instantiation and remove println in View Part 2
// new ManufacturerWindow(args);
System.out.println("Client mode:");
} else if ("server".equalsIgnoreCase(args[0])) {
// Create an instance of the Manufacturer server startup application window
// Uncomment instantiation and remove println in View Part 2
// new ManufacturerServerStartupWindow();
System.out.println("Server mode:");
} else {
/*
* Invalid run mode entered on command line, so send error message
* information to the error output (usually the screen).
*/
System.err.println("Command line run mode options are:");
System.err.println("\"\" - (no command line option): starts networked client");
System.err.println("\"client\" - starts non-networked client");
System.err.println("\"server\" - starts Manufacturer server application");
}
log.exiting("ManufacturerApplicationStartup", "ManufacturerApplicationStartup");
}
/**
* Prompts the user with an error message in a centred alert window.
*
* @param msg The message to display in the error window.
*/
public static void handleException(String msg) {
JOptionPane alert = new JOptionPane(msg, JOptionPane.ERROR_MESSAGE,
JOptionPane.DEFAULT_OPTION);
JDialog dialog = alert.createDialog(null, "Alert");
// Display alert error screen in centre of window
Dimension d = Toolkit.getDefaultToolkit().getScreenSize();
int x = (int) ((d.getWidth() - dialog.getWidth()) / 2);
int y = (int) ((d.getHeight() - dialog.getHeight()) / 2);
dialog.setLocation(x, y);
dialog.setVisible(true);
}
}
After adding the ManufacturerApplicationStartup
class your client
package should look similar to that in the screenshot below.
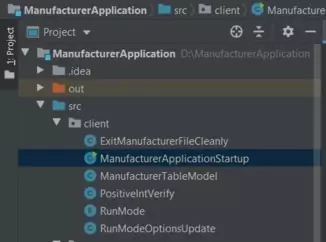
client
package after adding the ManufacturerApplicationStartup
class.Lesson 9 Complete
In this lesson we coded the View elements of the MVC pattern that are used on application startup.
Related Java Tutorials
Fundamentals - if
Construct
Exceptions - Handling Exceptions
API Contents - Inheritance - Using the package
keyword
API Contents - Inheritance - Using the import
keyword
Concurrency - Synchronization - Synchronized Blocks
Swing - Dialogs
What's Next?
In the final lesson of this section we code the View elements of the MVC pattern that are concerned with run mode options.