View Part 1 - DesignJ8 Home « View Part 1 - Design
In the View Part 1 section of the case study, consisting of four lessons, we look at the view part of the MVC paradigm and code as much of the view as we can from the information provided within the Project Proposal. Before we start coding the view we need to spend some time thinking about the overall design of the Graphical User Interface (GUI). This includes the common panel we are going to display to the user on startup for the entry of the Manufacturer file location and possibly a port, and how we are going to display the Manufacturer data in a readable form for our users whilst allowing options to search, stock and unstock.
Designing The GUI Top
Before we start coding the view we need to spend some time thinking about the overall design of the GUI. This includes the common panel we are going to display to the user on startup for the entry of the Manufacturer file location and possibly a port, and how we are going display the Manufacturer data in a readable form for our users whilst allowing options to search, stock and unstock.
In our first lesson of the section we draw up some rough sketches for our panels, make some design decisions with regards to user interaction with the panels and name some classes needed to implement our design choices.
Before we get started on designing and coding the GUI let's remind ourselves of the things requested for the client side of the application by the stakeholder Stocking Goods Limited:
The project will be written using Java technology only and must implement the following features:
- A client application that uses a GUI written in Swing which connects to the Manufacturer data file and can be run in networked or non-networked mode. This will be
achieved using the following command line arguments appended to our startup application:
- "client" - Run the application in non-networked mode so that access to the Manufacturer data file from the GUI runs in the same
JVM
and doesn't include any networking or serialization of objects. - "" (no argument) - Run the application in networked mode so that access to the Manufacturer data file from the GUI runs in a different
JVM
and includes networking and serialization of objects.
- "client" - Run the application in non-networked mode so that access to the Manufacturer data file from the GUI runs in the same
- Network server functionality that allows access to the Manufacturer data file remotely using RMI-JRMP. This will be achieved using the following command line
argument appended to our startup application:
- "server" - Run the application in server mode with functionality that provides the logic for displaying configurable objects and starting and exiting the server in a safe manner.
- Access to the Manufacturer data file must include search, stocking and unstocking functionality and provide record locking for the Manufacturer data file as specified in the Stock interface.
- Search functionality should include the capability to search by name, by location, by name and location or search all records.
- Stocking orders should only occur once. If the amount entered is wrong the goods will have to be unstocked and the correct stock order reentered.
Initial Design Decisions Top
We will place all our view code in the client
package as first mentioned in the Proposal Conclusions section.
We need a way to start up the application from the command line with or without arguments and so what we need is an application start up program that does some validation, sets the 'look and feel'
and then invokes another program dependant upon the any command line argument entered. This will be the first program we code for the GUI and as this is an application to work with the Manufacturer
file we will call this program ManufacturerApplicationStartup
which is an informative name for what the class does. Assuming run mode checks pass control will be passed to a class to
start up the server (ManufacturerServerStartupWindow
), or to display Manufacturer information (ManufacturerWindow
) both of which we will defer coding until the
View Part 2 section of the case study. In the case of the ManufacturerWindow
which will always be run for a client we need a way to validate the run
mode options entered for non-network clients / network clients and for this purpose we will write a class called RunModeDialog
.
The run modes entered are constants and do not vary from run to run and so we can use an Enumerated class to hold the run mode values, which we will call RunMode
.
Computer users are familiar with data being displayed in a tabular format in rows and columns and the data held within the Manufacturer file certainly fits nicely into this paradigm. In Swing we use
the JTable component to display data in a tabular format. The constructors of the JTable
component have some disadvantages such as making every field in a table editable. Luckily we can create our own table models which is exactly what we will do and we will call this class
ManufacturerTableModel
.
We have a method to shut down the Manufacturer file within the Model.StockImpl
class called lockLockingMap()
that locks the locking map manufacturerRecordNumbersLock
so that no other client can modify it. We will create a class called ExitManufacturerFileCleanly
that accesses this method to ensure a clean shutdown of the file.
When a user starts up the application for the first time we would like to have a default value for the port number. It would also be user friendly if the last set of run mode options entered by a
user were persisted for the next time the application is used and we will do this in the SavedRunModeOptions
class.
Common Run Mode Options Panel Top
Regardless of the run mode entered most of the options available for entry by the user are common and so we can save a lot of effort by designing a single panel for user entry of the Manufacture file / port number and modify this dependant upon the run mode entered.
The following rough sketches show how the common panel will look dependant upon the run mode entered. We can create a class for this called RunModeOptions
where we can create a common
panel used by both the client and server applications to specify the run mode options. The panel changes slightly dependent on the run mode entered on application startup but has a "common feel" for
all three run modes.
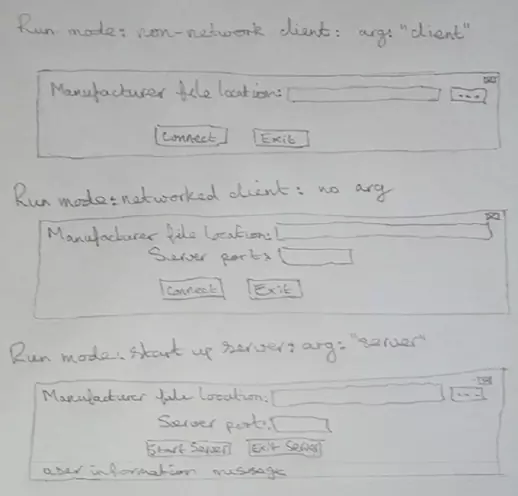
We also need an automated way to transfer information about changes to our RunModeOptions
panel to any interested observers and for this purpose we will code a RunModeOptionsUpdate
class to do this.
For the box with the ellipsis (...
) we will need to code a utility class that provides the user with the ability to browse for the Manufacturer file rather than forcing them to remember (and type in) a
fully qualified Manufacturer file location.
We can validate the port number entered within this panel in a user friendly way and to do this we will write a class to verify user entry called PositiveIntVerify
.
Manufacturer Panel Top
The other screen we have to design is a bit more involved and needs to encompass the requests from the stakeholder for search functionality, stocking and unstocking and make use of our custom made
table model class ManufacturerTableModel
.
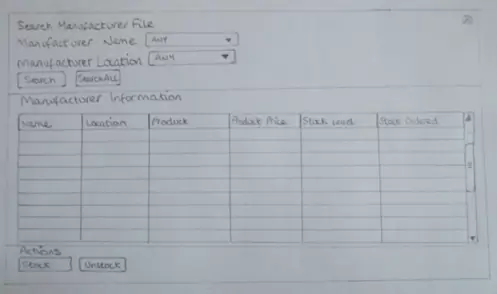
We will create a class called ManufacturerWindow
to render our Manufacturer data and functionality and will defer coding this to the View Part 2 section of the case study.
Lesson 7 Complete
In this lesson we nailed down most of the design for the View elements of the MVC pattern that can be derived from the project proposal.
Related Java Tutorials
Objects & Classes - Class Structure and Syntax
Swing - Swing Containers
What's Next?
In the next lesson we begin coding the View elements of the MVC starting with the classes that can be coded independently.