Inheritance - Using super
QuizJ8 Home « Inheritance - Using super
Quiz
OO Concepts Quiz 7
The quiz below tests your knowledge of the material learnt in OO Concepts - Lesson 7 - Inheritance - Using super
.
Question 1 : We can explicitly code
super()
to access what? - We can explicitly code <code>super()</code> to access <code>superclass</code> constructors.
Quiz Progress Bar
Quiz 1
Access Modifiers
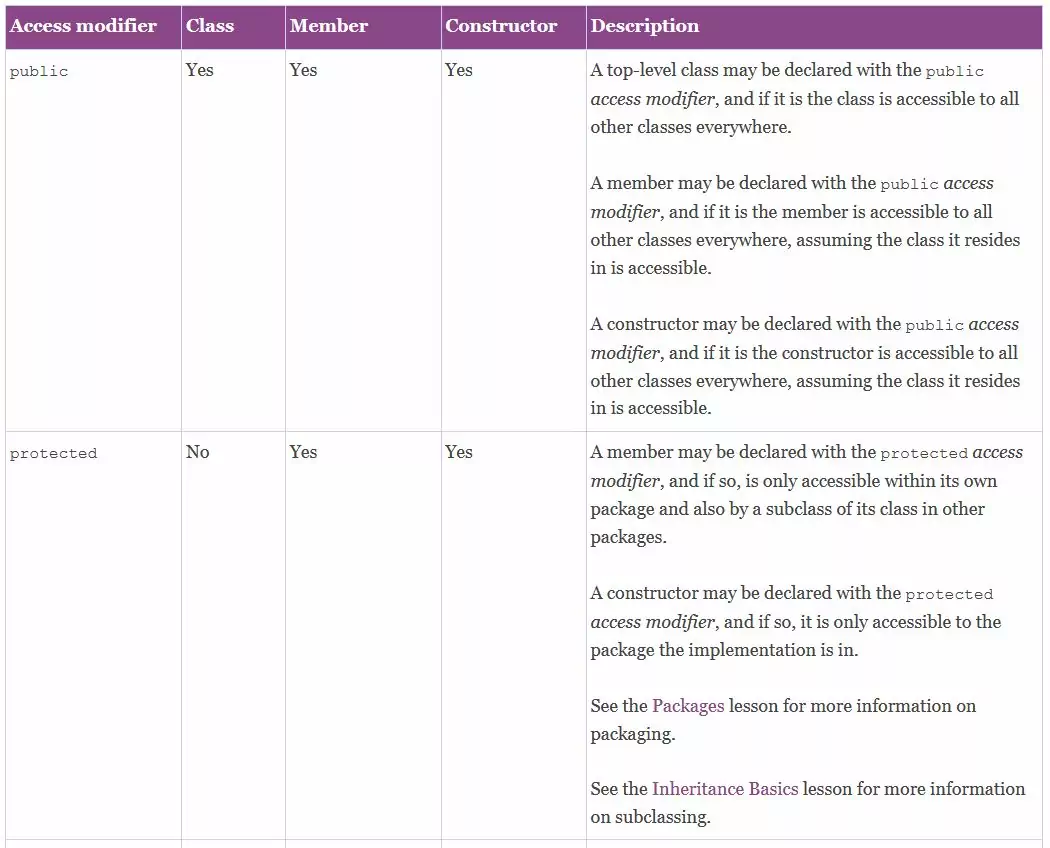
Quiz 2
Protecting Our Data
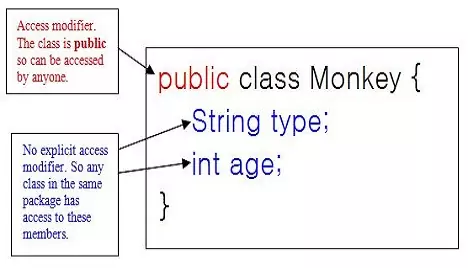
Quiz 3
Inheritance - Basics
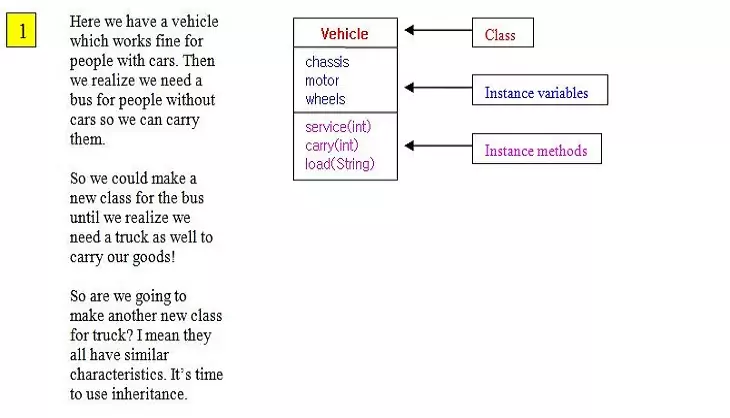
Quiz 4
Inheritance - extends
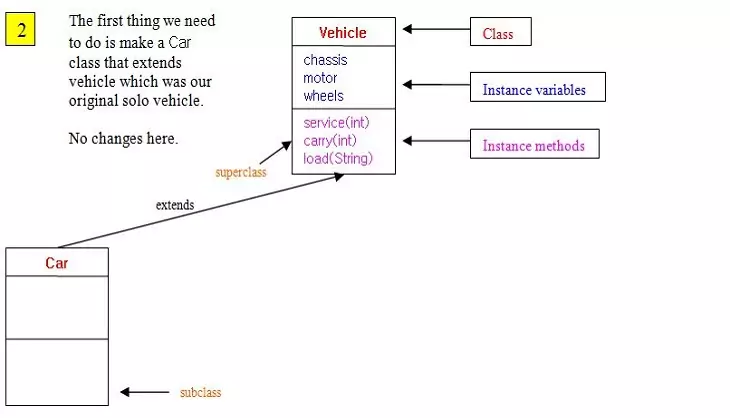
Quiz 5
Inheritance - Overriding
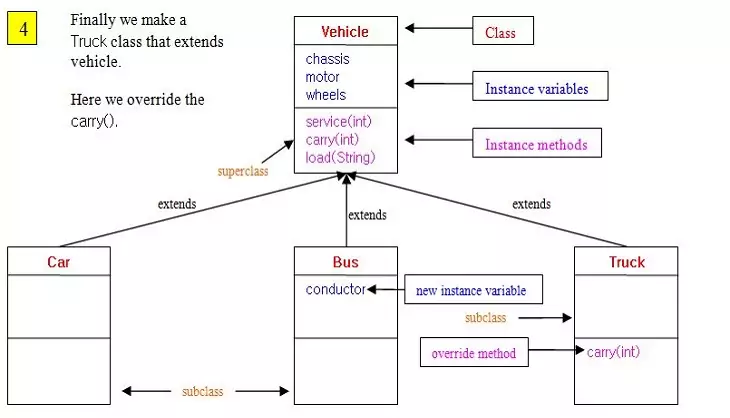
Quiz 6
Inheritance - Concepts
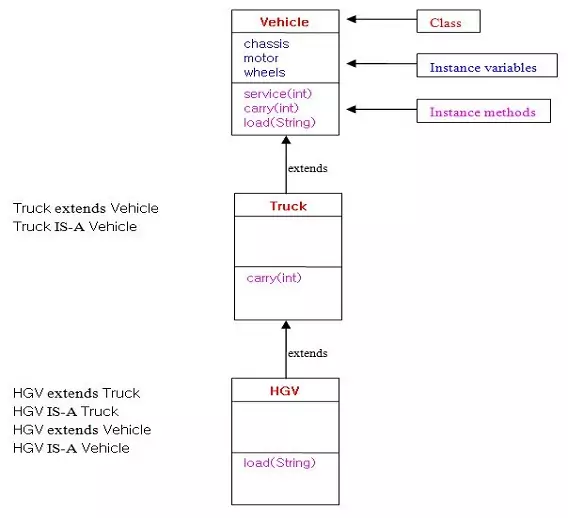
Quiz 8
Abstraction
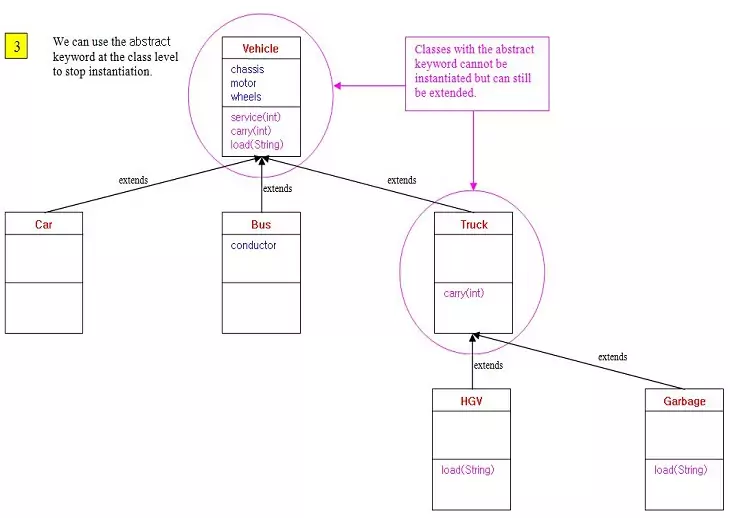
Quiz 9
Polymorphism
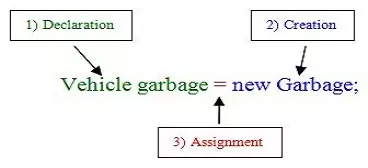
Quiz 10
Interfaces
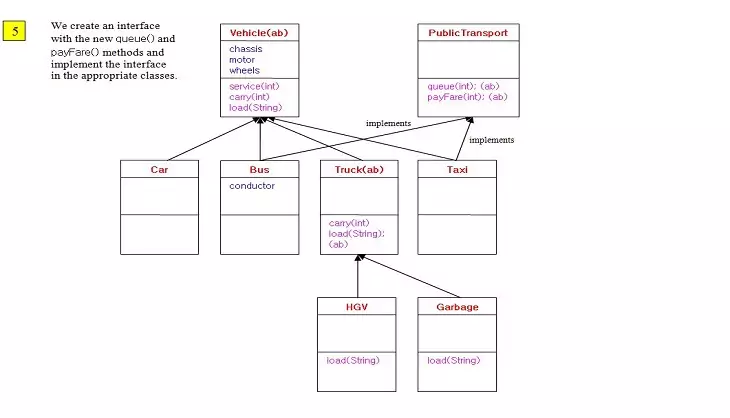
Quiz 11
Interfaces - Default Methods
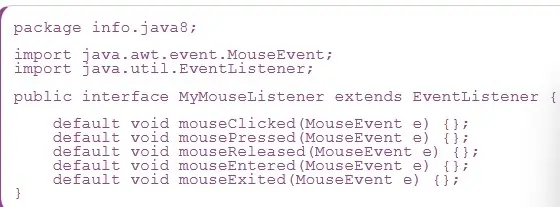
Quiz 12
Deeper Into Polymorphism
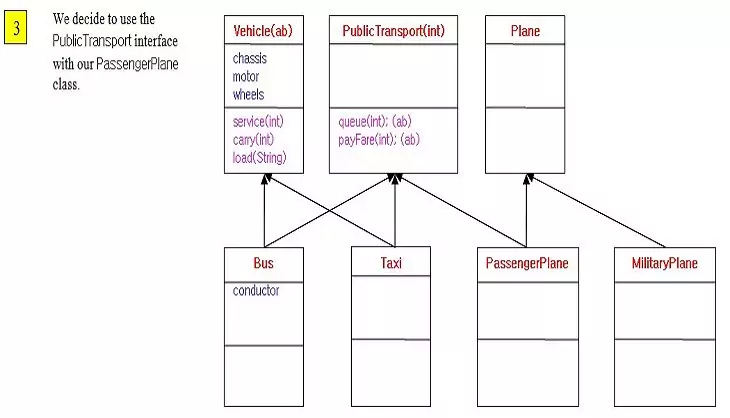
Quiz 13
Functional Interfaces
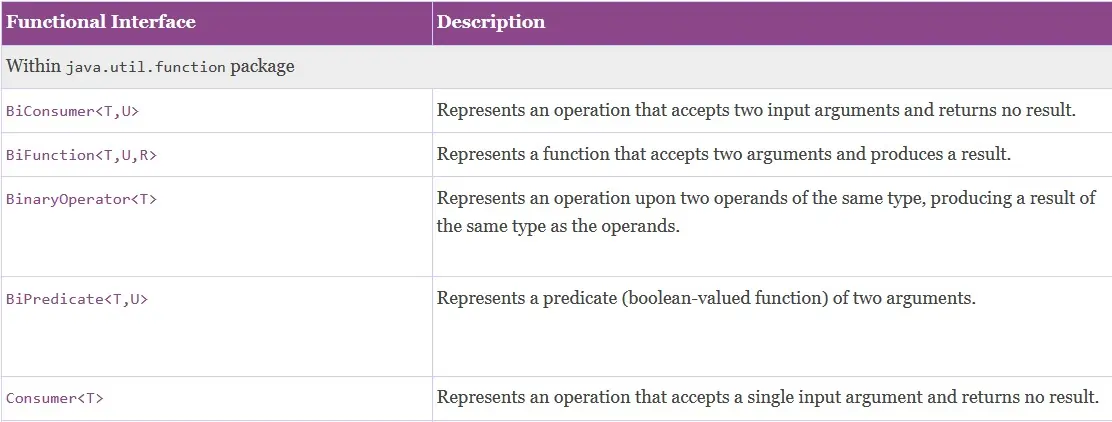
Quiz 14
Lambda Expressions
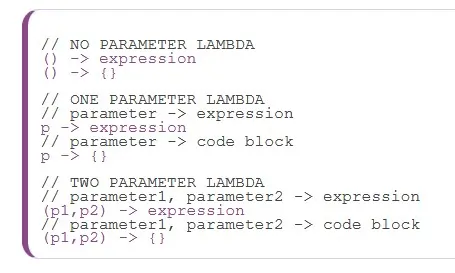
Quiz 15
Method References
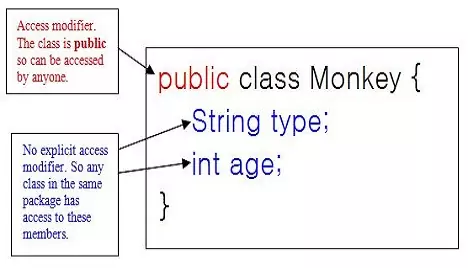
Quiz 16
Nested Static Classes
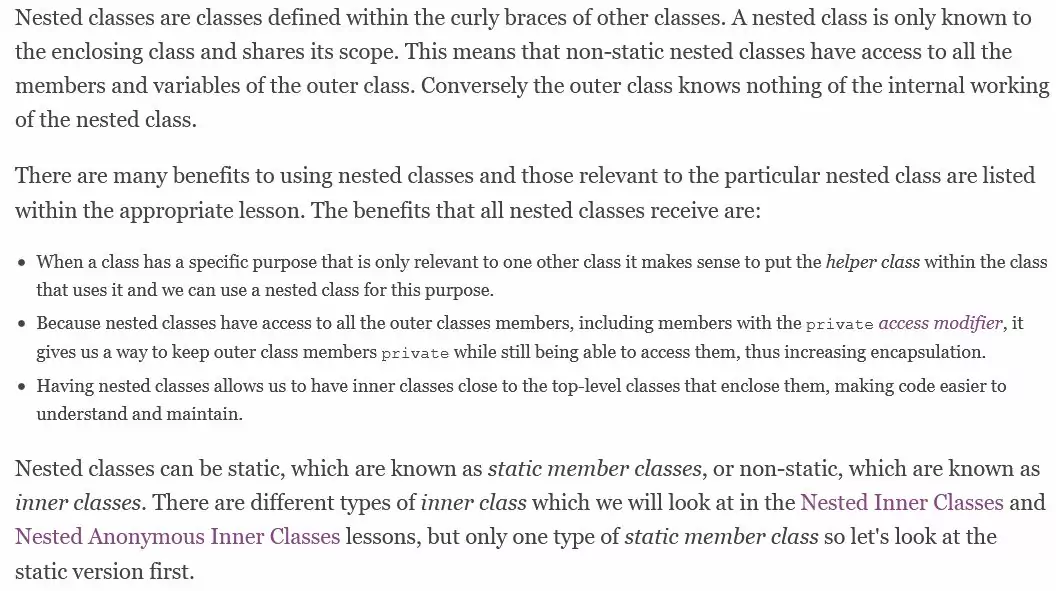
Quiz 17
Nested Inner Classes
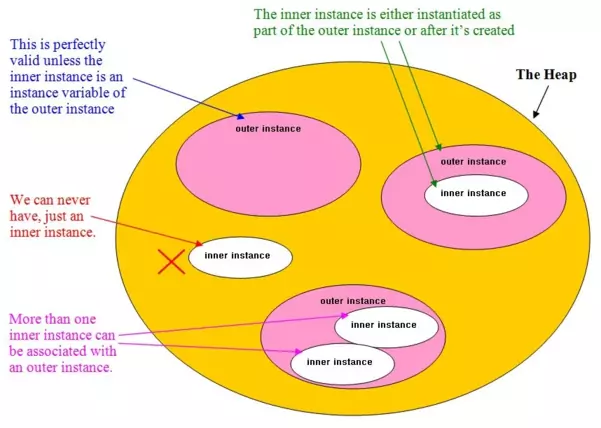
Quiz 18
Anonymous Inner Classes

Quiz 19
Object
Superclass
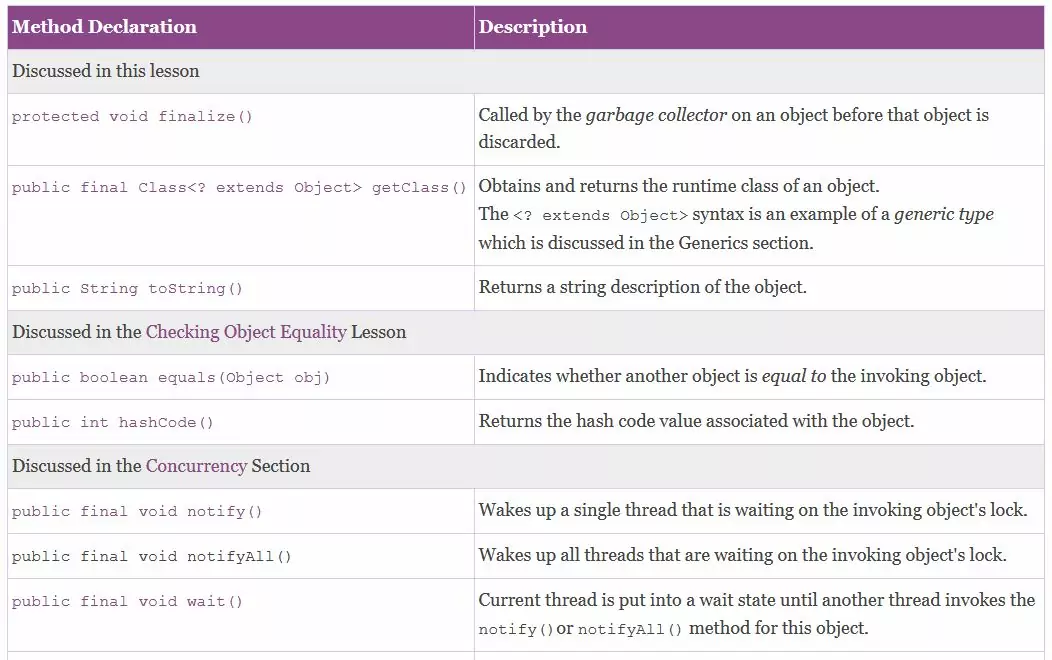
Quiz 20
Checking Object Equality
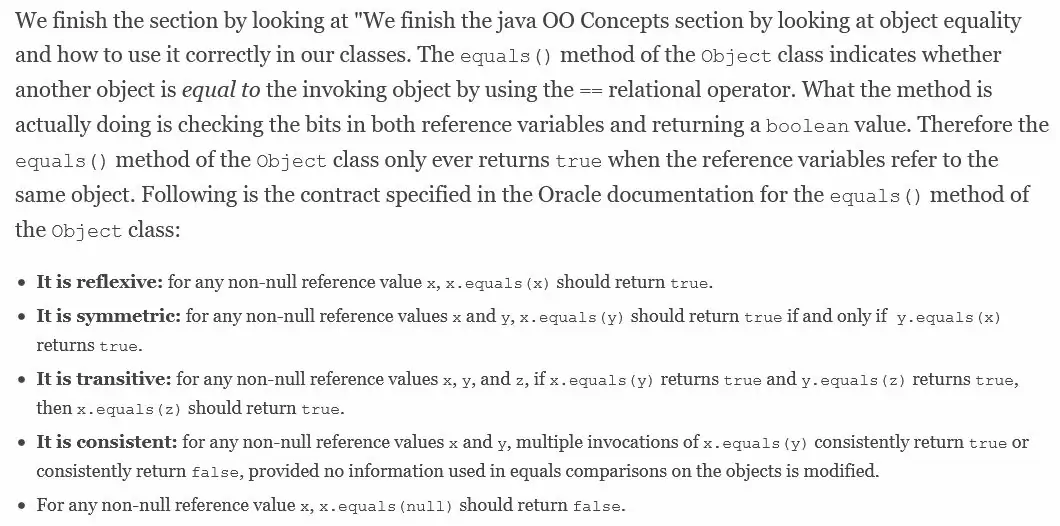
What's Next?
In the next quiz we test your knowledge of abstraction.