Inheritance - Using extends
QuizJ8 Home « Inheritance - Using extends
Quiz
OO Concepts Quiz 4
The quiz below tests your knowledge of the material learnt in OO Concepts - Lesson 4 - Inheritance - Using extends
.
Question 1 : What is output from the following code snippet?
package info.java8;
public class A {
}
package info.java8;
public class B {
}
package info.java8.sub;
import info.java8.A;
import info.java8.B;
public class AA extends A, B {
public static void main(String[] args) {
System.out.println("In class AA");
}
}
- The code snippet doesn't compile as class <code>AA</code> cannot extend more than one class.
Quiz Progress Bar
Quiz 1
Access Modifiers
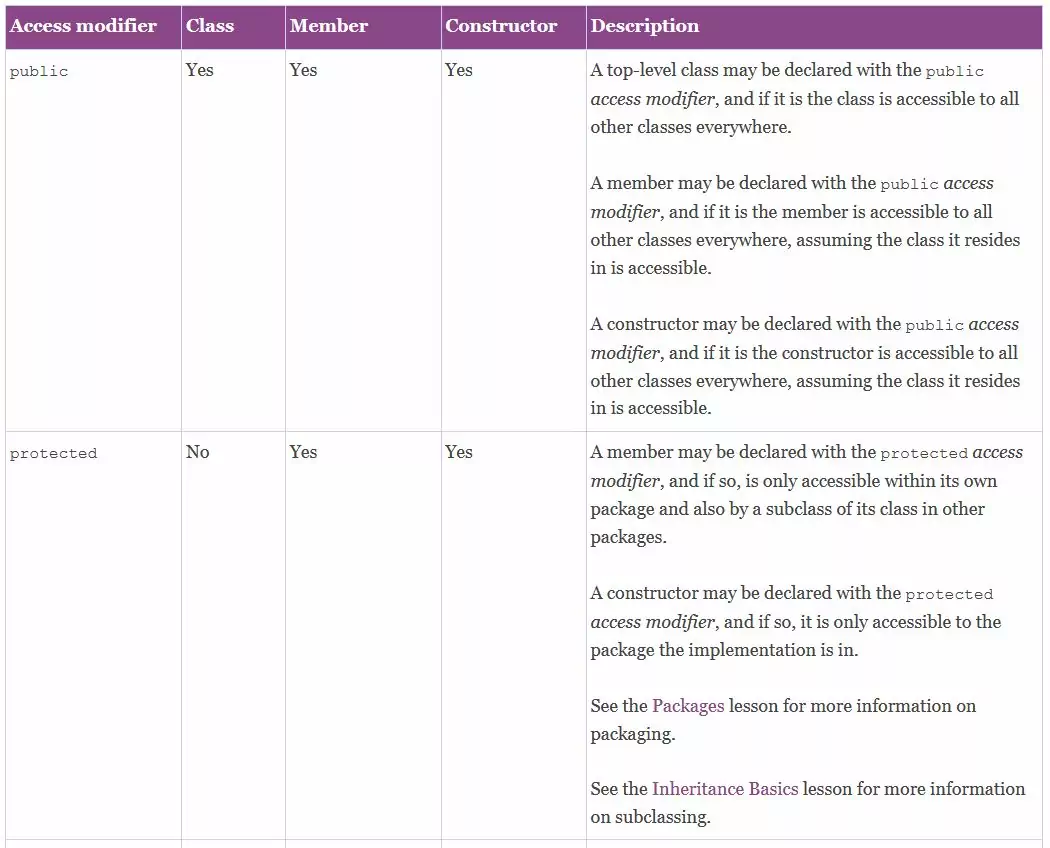
Quiz 2
Protecting Our Data
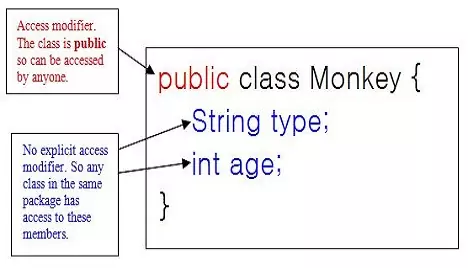
Quiz 3
Inheritance - Basics
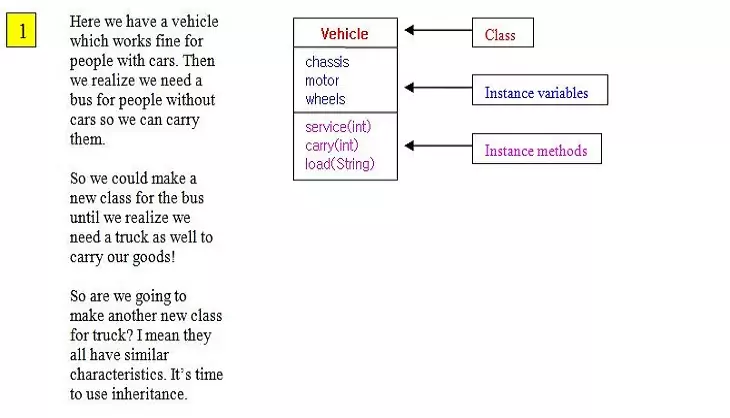
Quiz 5
Inheritance - Overriding
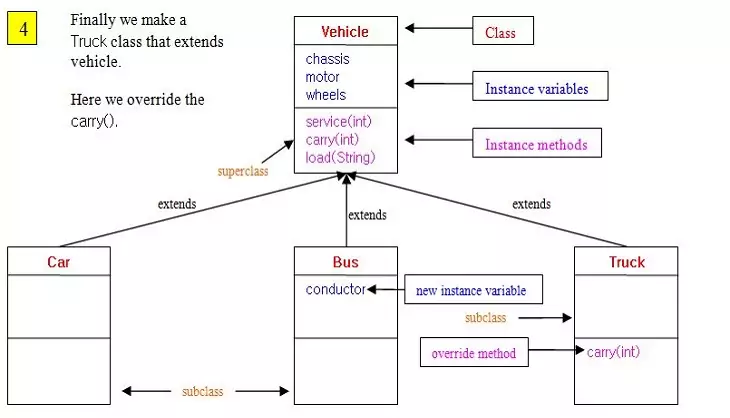
Quiz 6
Inheritance - Concepts
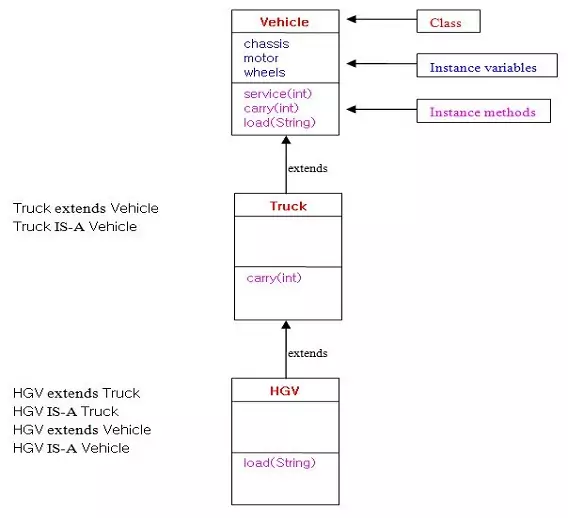
Quiz 7
Inheritance - super
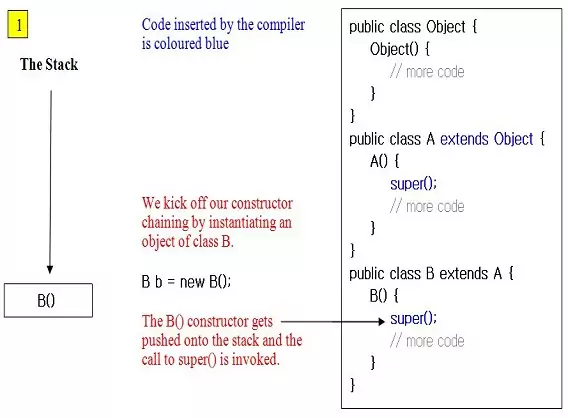
Quiz 8
Abstraction
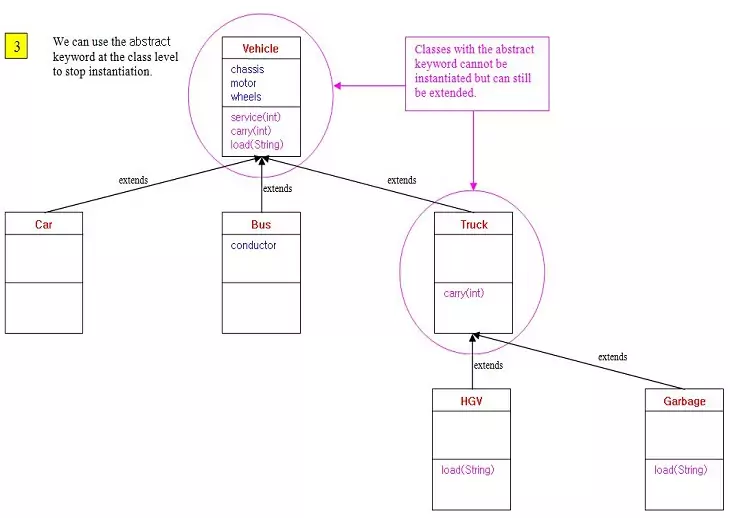
Quiz 9
Polymorphism
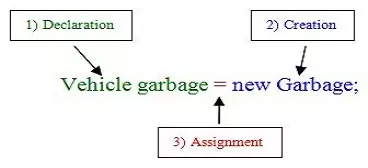
Quiz 10
Interfaces
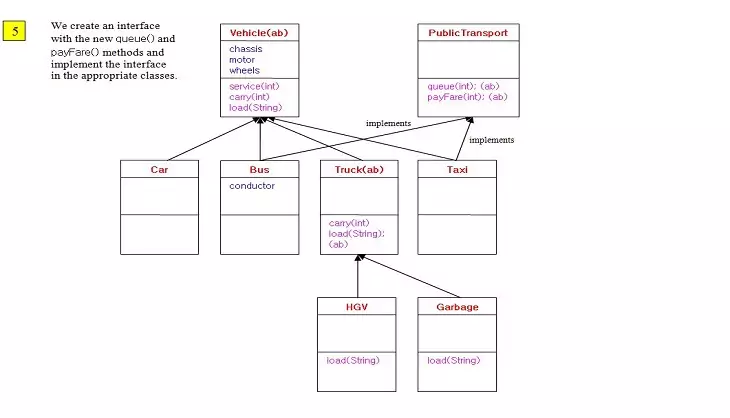
Quiz 11
Interfaces - Default Methods
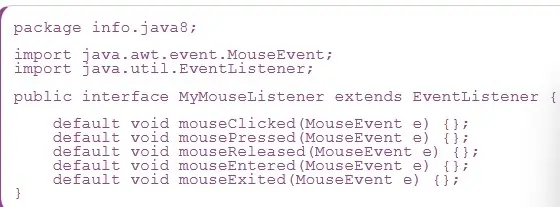
Quiz 12
Deeper Into Polymorphism
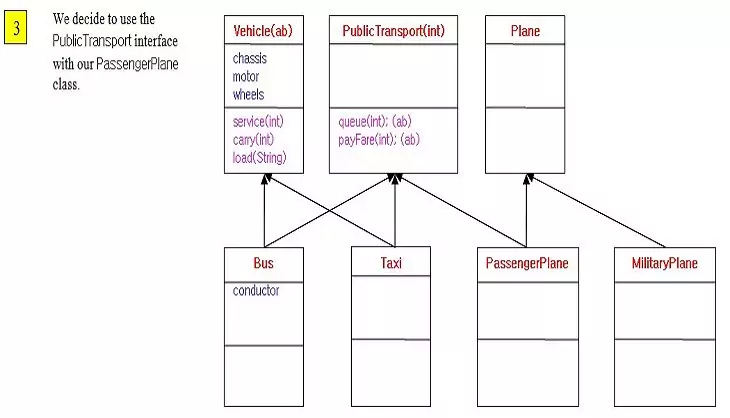
Quiz 13
Functional Interfaces
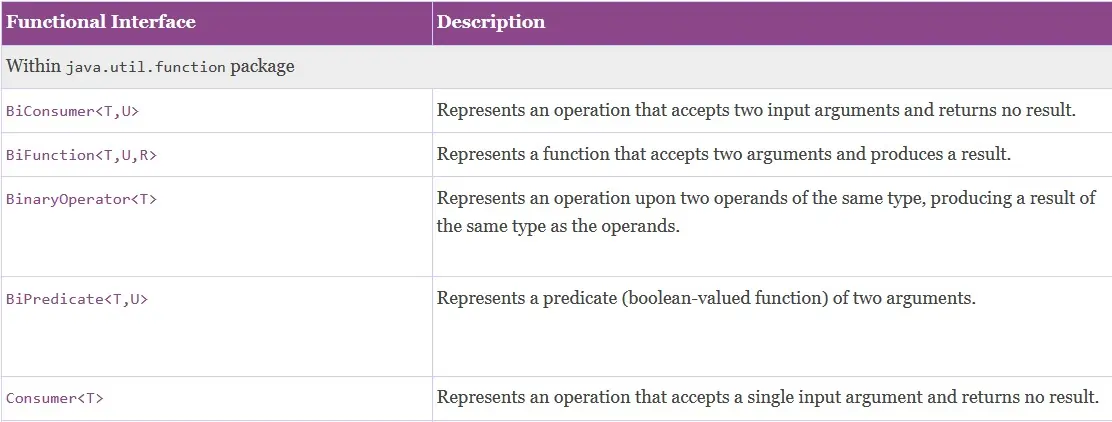
Quiz 14
Lambda Expressions
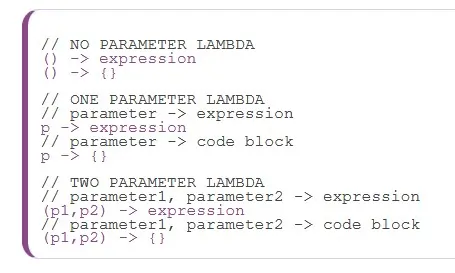
Quiz 15
Method References
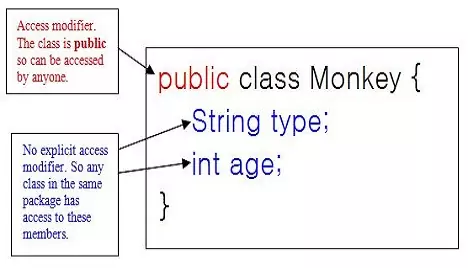
Quiz 16
Nested Static Classes
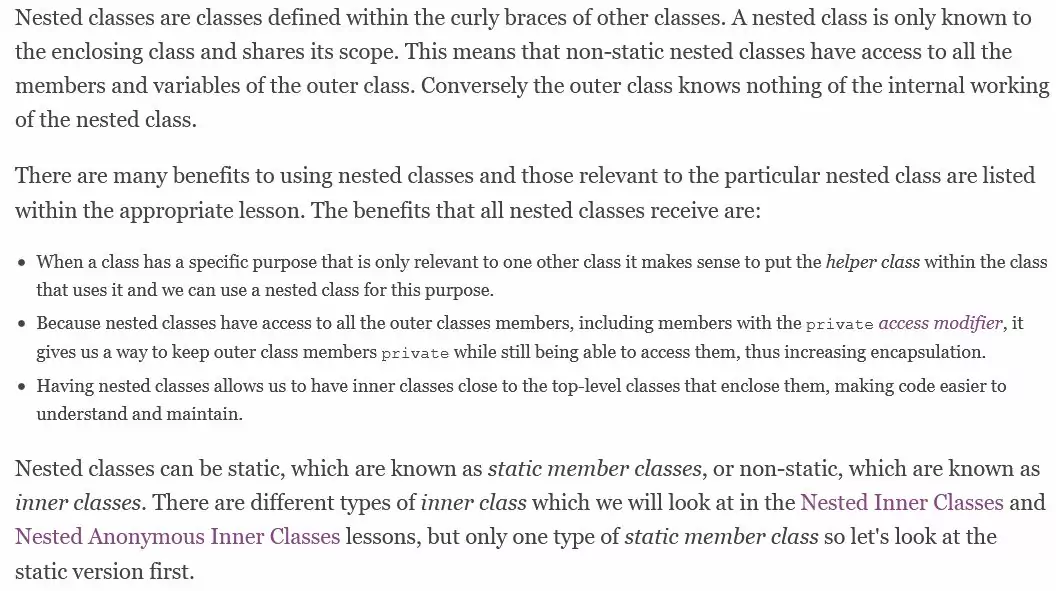
Quiz 17
Nested Inner Classes
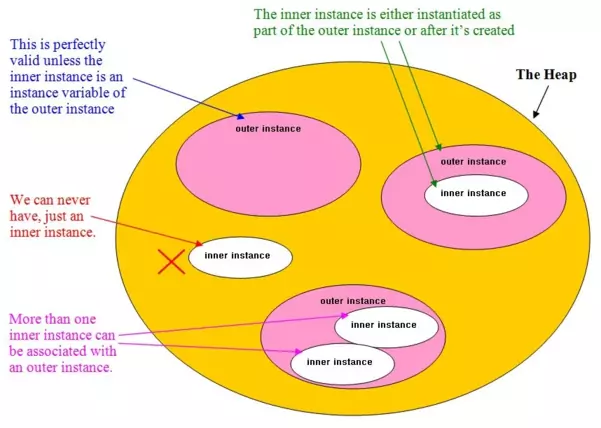
Quiz 18
Anonymous Inner Classes

Quiz 19
Object
Superclass
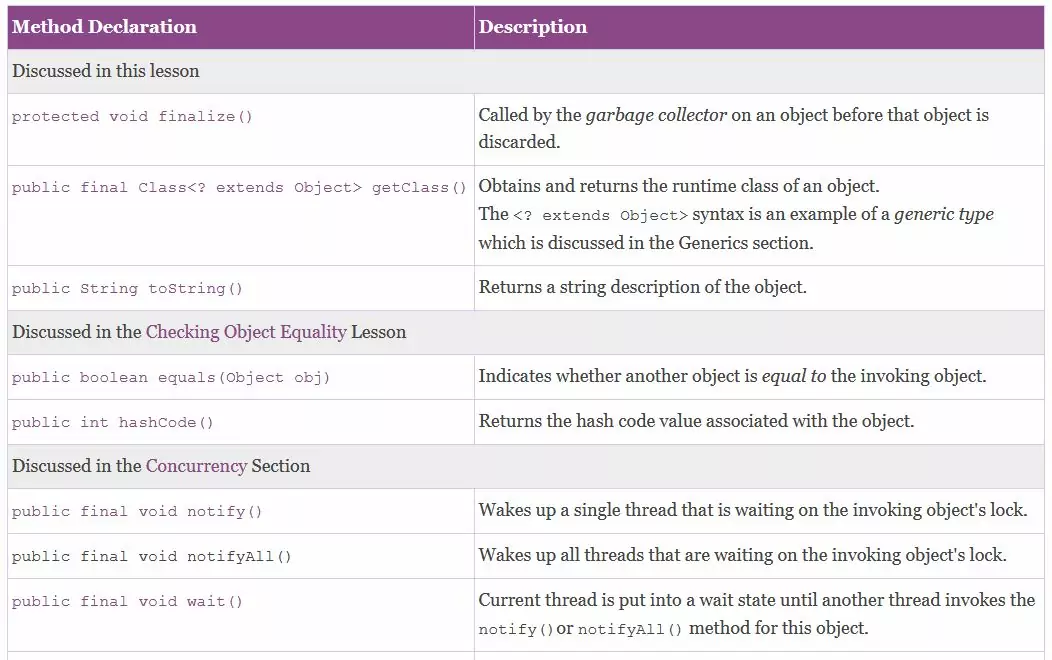
Quiz 20
Checking Object Equality
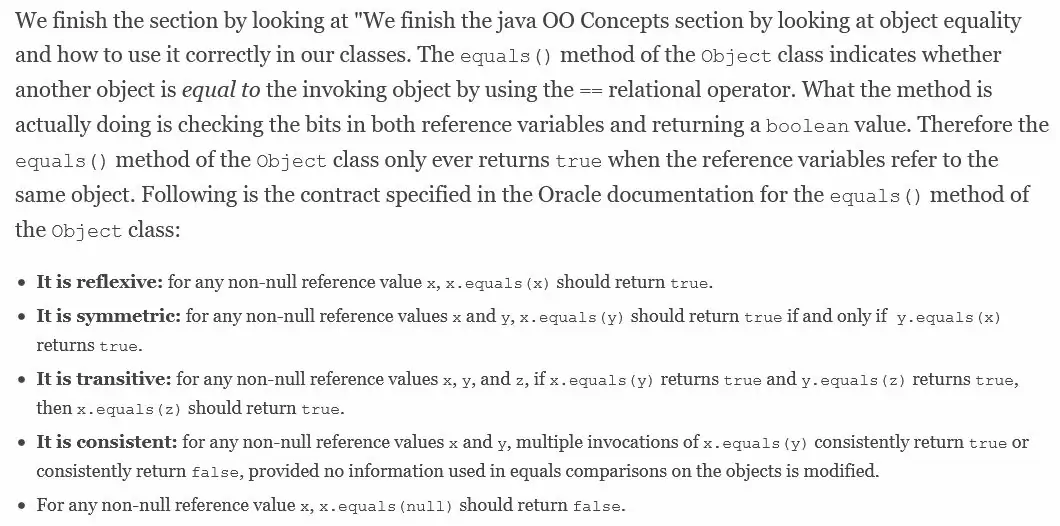
What's Next?
In the next quiz we test your knowledge of overriding methods when using inheritance.