Generic MethodsJ8 Home « Generic Methods
Generic Methods Top
We have seen several examples of generic methods so far in this lesson and these were all contained within generic classes. It is also possible to have generic methods inside non-generic classes.
Following is a non-generic class that contains a generic method. Two things of note are generic methods can be static or non-static and notice where we declare the bounded type. When declaring a generic method in a non-generic class then we have to declare the generic type before the return type within the method:
package info.java8;
/*
Non-generic class containing a generic method
*/
public class SquareNumber {
public static void main (String[] args) {
// Call generic method with a Double
Double d = 12.12;
genMethod(d);
// Call generic method with an Integer
Integer i = 11;
genMethod(i);
// Call generic method with a String
String s = "generics";
genMethod(s);
}
/*
Method to print square of integer
*/
public static <T extends Number> void genMethod (T number) { // Generic type declared before return type
System.out.println("Input squared as integer = " + number.intValue() * number.intValue());
}
}
Building the SquareNumber
class produces the following output:
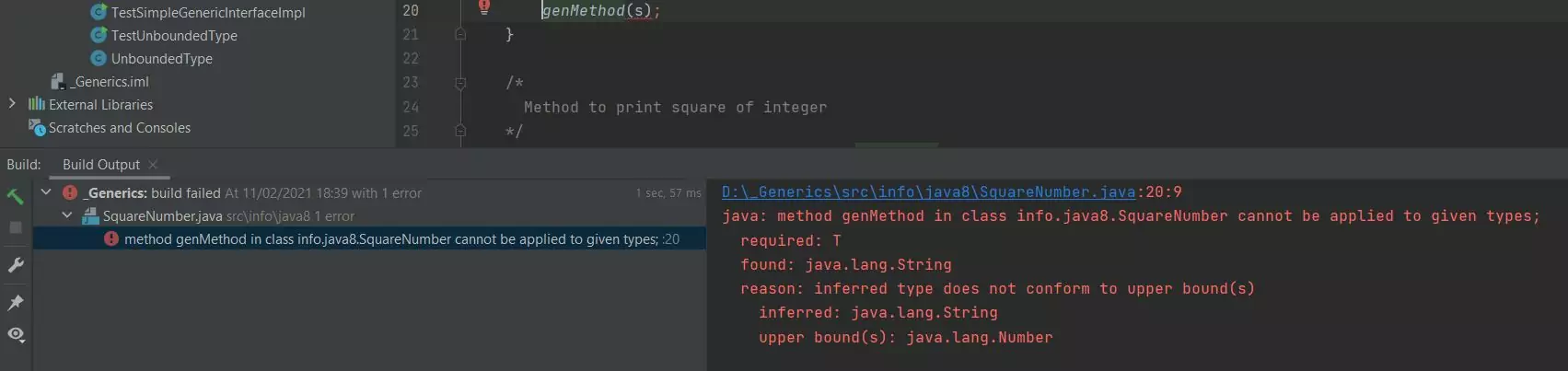
SquareNumber
class.The build failed as expected as the String
object does not extend the Number
object.
Remove the comment and last 2 lines of code from the main()
method of the SquareNumber
class as shown below.
package info.java8;
/*
Non-generic class containing a generic method
*/
public class SquareNumber {
public static void main (String[] args) {
// Call generic method with a Double
Double d = 12.12;
genMethod(d);
// Call generic method with an Integer
Integer i = 11;
genMethod(i);
}
/*
Method to print square of integer
*/
public static <T extends Number> void genMethod (T number) { // Generic type declared before return type
System.out.println("Input squared as integer = " + number.intValue() * number.intValue());
}
}
Running the amended SquareNumber
class produces the following output:
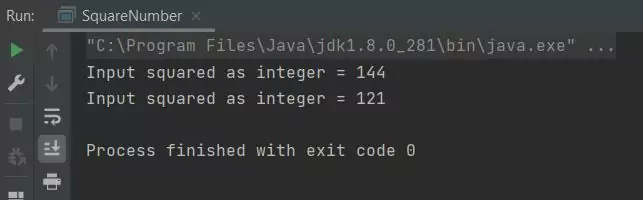
SquareNumber
class.As the screenshot shows the method now works fine and will only accept Number
objects and subclasses thereof.
Related Quiz
Generics Quiz 9 - Generic Methods Quiz
Lesson 9 Complete
In this lesson we looked at generic methods and how to use them.
What's Next?
In the next lesson we look at generic constructors.