Generic ConstructorsJ8 Home « Generic Constructors
We have seen several examples of generic constructors so far in this section and these were all contained within generic classes. It is also possible to have generic constructors inside non-generic classes.
Following is a non-generic class that contains a generic constructor. Notice where we declare the bounded type, when declaring a generic constructor in a non-generic class then we have to declare the generic type before the constructor name:
package info.java8;
/*
Non-generic class containing a generic constructor
*/
public class CubeNumber {
private final int cubedNumber;
// Pass bounded type to our constructor
public <T extends Number> CubeNumber(T number) {
cubedNumber = number.intValue() * number.intValue() * number.intValue();
}
// getter for cubed number
public int getNumber() {
return cubedNumber;
}
}
Building the CubeNumber
class produces the following output:
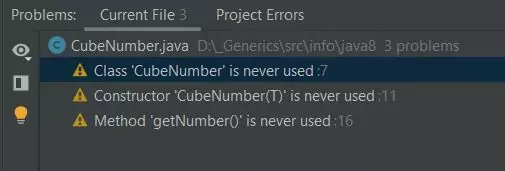
CubeNumber
class.Now we need to write a test class to show how we can pass various Number
objects to our CubeNumber
class:
package info.java8;
/*
Test our CubeNumber class that accepts Number and subclasses for its constructor
*/
public class TestCubeNumber {
public static void main(String[] args) {
// Test the CubeNumber using an Integer object
CubeNumber integerObj = new CubeNumber(12);
System.out.println("Input cubed = " + integerObj.getNumber());
// Test the CubeNumber using a Double object
CubeNumber doubleObj = new CubeNumber(13.45);
System.out.println("Input cubed = " + doubleObj.getNumber());
// Test the CubeNumber using a String object
CubeNumber StringObj = new CubeNumber("foobar");
System.out.println("Input cubed = " + StringObj.getNumber());
}
}
Building the TestCubeNumber
class produces the following output:
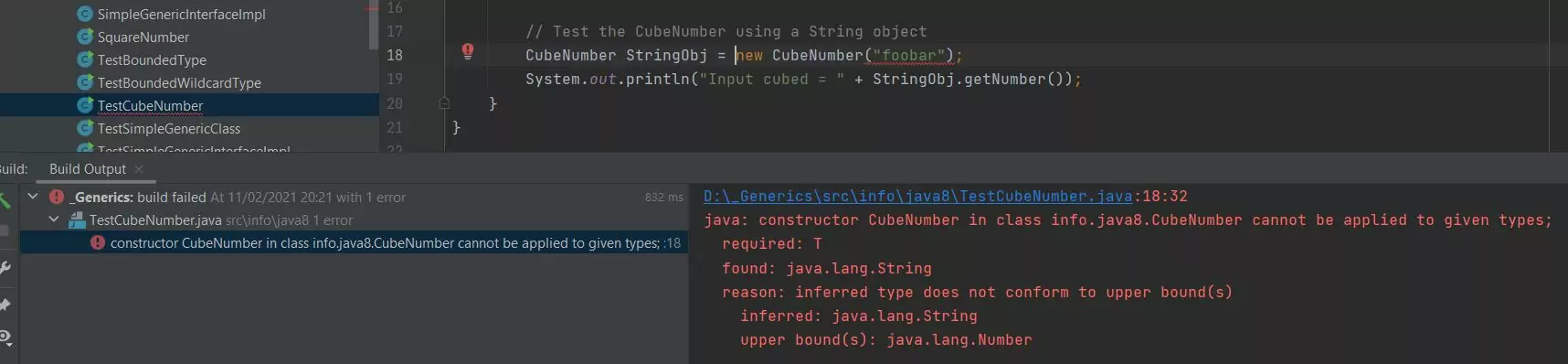
TestCubeNumber
class.The build failed as expected as the String
object does not extend the Number
object.
Remove the comment and last 2 lines of code from the main()
method of the TestCubeNumber
class as shown below.
package info.java8;
/*
Test our CubeNumber class that accepts Number and subclasses for its constructor
*/
public class TestCubeNumber {
public static void main(String[] args) {
// Test the CubeNumber using an Integer object
CubeNumber integerObj = new CubeNumber(12);
System.out.println("Input cubed = " + integerObj.getNumber());
// Test the CubeNumber using a Double object
CubeNumber doubleObj = new CubeNumber(13.45);
System.out.println("Input cubed = " + doubleObj.getNumber());
}
}
Running the amended TestCubeNumber
class produces the following output:
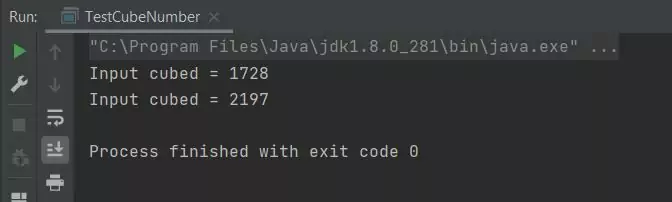
TestCubeNumber
class.As the screenshot shows the method now works fine and will only accept Number
objects and subclasses thereof.
Related Quiz
Generics Quiz 10 - Generic Constructors Quiz
Lesson 10 Complete
In this lesson we looked at generic constructors and how to use them.
What's Next?
That's it for the generics section, the next section is all about Java streams.