UtilitiesJ8 Home « Utilities
In this lesson we look at the Utilities hierarchy and examine the java.util.Arrays
and java.util.Collections
classes that contains a lot of static
utility methods we can use with our arrays and collections.
Utilities Hierarchy Diagram Top
The diagram below is a representation of the Utilities hierarchy and covers the classes we will study in this lesson.
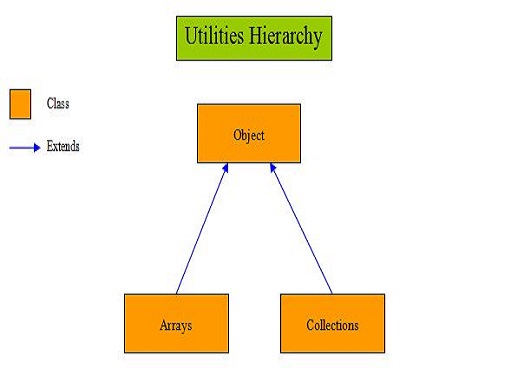
java.util.Arrays
Class Top
The java.util.Arrays
class gives us methods to search, compare, fill, get a hashcode, sort and give a readable string representation of arrays. All the methods accept Object
and most
are overloaded to accept a primitive type, appropriate to the method in question. The class also includes a static factory that allows arrays to be viewed as lists.
Arrays
Method Overview Top
The table below shows the declarations of the methods for the java.util.Arrays
class used in the example below:
Method Declaration | Description |
---|---|
Used in the example below | |
public static <T> List<T> asList(T... a) | Returns a fixed-size list backed by the specified array so that changes to the returned list are relected in the array. |
public static int binarySearch(int[] a, int key) | Searches the specified array of ints for the specified key value using the binary search algorithm. |
public static String deepToString(Object[] a) | Returns a string representation of the "deep contents" of the specified array. |
public static boolean equals(int[] a, int[] a2) | Returns true if the two specified arrays of ints are equal to one another. |
public static int hashCode(int[] a) | Returns a hash code based on the contents of the specified array. |
public static void sort(int[] a) | Sorts the specified array of ints into ascending numerical order. |
For more information on the other methods in the java.util.Arrays
class the following link will take you to the online version of documentation for the JavaTM 2 Platform Standard Edition 5.0 API Specification.
Take a look at the documentation for the java.util.Arrays
class which you can find by scrolling down the lower left pane and clicking on Arrays.
Arrays
Example Top
Lets write a simple class that creates some arrays and uses some of the methods from the java.util.Arrays
class on them:
package info.java8;
/*
Simple class to create some array and use several methods from the java.util.Arrays class
*/
import java.util.*; // Import the java.util package
public class UsingJavaUtilArrays {
public static void main (String[] args) {
Integer[][] intArray1 = { {5, 33}, {0}, {5, 4, 71} };
List<Integer[]> l = Arrays.asList(intArray1);
System.out.println(l.toString());
System.out.println("intArray1 is " + Arrays.deepToString(intArray1));
System.out.println("intArray1[0] is " + Arrays.deepToString(intArray1[0]));
System.out.println("intArray1[1] is " + Arrays.deepToString(intArray1[1]));
System.out.println("intArray1[2] is " + Arrays.deepToString(intArray1[2]));
int intArray2[] = {0, 1, 5, 8, 6, 3, 4, 9, 2, 7};
System.out.println("intArray2 hashcode = " + Arrays.hashCode(intArray2));
System.out.println("Binary search of intArray2 for a value of 4 = "
+ Arrays.binarySearch(intArray2, 4));
int intArray3[] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
System.out.println("intArray3 hashcode = " + Arrays.hashCode(intArray3));
System.out.println("intArray2 = intArray3 ? " + Arrays.equals(intArray2, intArray3));
Arrays.sort(intArray2);
System.out.println("intArray2 hashcode = " + Arrays.hashCode(intArray2));
System.out.println("intArray2 = intArray3 ? " + Arrays.equals(intArray2, intArray3));
}
}
Save, compile and run the UsingJavaUtilArrays
class in directory c:\_Collections in the usual way.
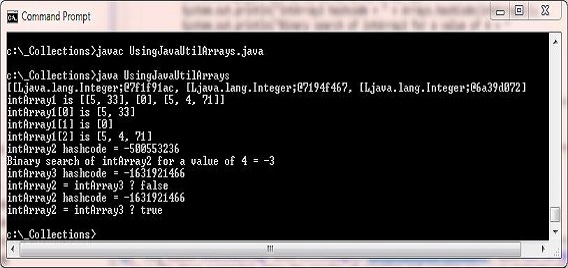
The above screenshot shows the results of creating and running our UsingJavaUtilArrays
class. A few points of interest are the asList()
method which converts an array to a list. This
method acts as a bridge between the array-based and collection-based APIs, when used in combination with java.util.Collection.toArray()
. The returned list is serializable and implements
RandomAccess
much as an ArrayList would. The other point to notice is how the hashcode()
and equals
methods are performed. Even though
the contents of intArray2
and intArray3
are the same (before the sort) they are in a different order and so have different hashcodes and therefore cannot be equal. After the sort the
arrays exactly match in contents and ordering and so are equal.
java.util.Collections
Class Top
The java.util.Collections
class static methods give us ways to search, typesafe, empty, synchronize, immute, sort and several other ways to work with our collections. As a reminder collections
only work with reference variables, so if you are using primitive variables then these need to be boxed before adding to a collection, or use an array instead.
Collections
Method Overview Top
The table below shows the declarations of the methods for the java.util.Collections
class used in the example below:
Method Declaration | Description |
---|---|
Used in the example below | |
public static <T> int binarySearch( | Searches the specified list for the specified object key using the binary search algorithm. |
public static <T> void copy( | Copies all of the elements from the src list to the dest list. |
public static static void reverse(List<?> list) | Reverses the order of the elements in the specified list . |
public static static void shuffle(List<?> list) | Randomly permutes the specified list using a default source of randomness. |
public static <T extends Comparable<? super T>> | Sorts the specified list into ascending order, according to the natural ordering of its elements. |
For more information on the other methods in the java.util.Collections
class the following link will take you to the online version of documentation for the JavaTM 2 Platform Standard Edition 5.0 API Specification.
Take a look at the documentation for the java.util.Collections
class which you can find by scrolling down the lower left pane and clicking on Collections.
Collections
Example Top
Lets write a simple class that creates some collections and uses some of the methods from the java.util.Collections
class on them:
package info.java8;
/*
Simple class to create some collections and use several methods from the java.util.Collections class
*/
import java.util.*; // Import the java.util package
public class UsingJavaUtilCollections {
public static void main (String[] args) {
List<String> al = new ArrayList<String>();
List<String> al2 = new ArrayList<String>();
String s = "hello";
al.add(s);
al.add(s);
al.add("goodbye");
al.add(s+s);
al.add("goodbye2");
al2.add(s);
al2.add(s);
al2.add("goodbye");
al2.add(s+s);
al2.add("goodbye2");
al2.add(s);
System.out.println("Binary search of al for a value of goodbye = " +
Collections.binarySearch(al, "goodbye"));
System.out.println("The content of al, before sort: " + al);
Collections.reverse(al);
System.out.println("The content of al, after sort: " + al);
Collections.shuffle(al);
System.out.println("The content of al, after shuffle: " + al);
System.out.println("The content of al2, before copy: " + al2);
Collections.copy(al2, al);
System.out.println("The content of al2, after copy: " + al2);
Collections.sort(al2);
System.out.println("The content of al2, after sort: " + al2);
}
}
Save, compile and run the UsingJavaUtilCollections
class in directory c:\_Collections in the usual way.
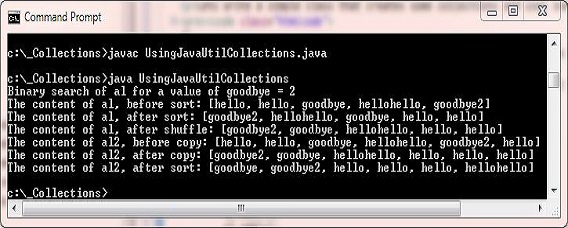
The above screenshot shows the results of creating and running our UsingJavaUtilCollections
class. The names of the methods used are fairly self explanatory so no need to go into details about the above code.
Lesson 7 Complete
In this lesson we looked at the Utilities hierarchy and examine the java.util.Arrays
and java.util.Collections
classes that contains a lot of static
utility methods we can use with our collections.
What's Next?
In our final lesson in the Collections section we look at sorting our collections using the Comparable
and Comparator
interfaces.