Dates, Numbers & CurrenciesJ8 Home « Dates, Numbers & Currencies
In this lesson we look at the Date
, Calendar
, Locale
, DateFormat
and NumberFormat
classes that allow us to create and manipulate dates, times, numbers and
currencies for different regions of the world. The table below gives a brief description of each class that we will be using in this lesson:
Class | Description |
---|---|
java.util.Date | The Date class allows us to create an object that represents a specific instant in time. |
java.util.Calendar | The Calendar class allows us get an instance of a Calendar object which we can use to convert and manipulate dates and times. |
java.util.Locale | The Locale class allows us to create an object that represents a specific geographical, political, or cultural region of the world. We can then use the Locale
object in conjunction with the DateFormat or NumberFormat classes to get locale specific dates, times, numbers and currencies for that locale. |
java.text.DateFormat | The DateFormat class provides us with methods to format dates in various styles and for different locales. |
java.text.NumberFormat | The NumberFormat class provides us with methods to format numbers and currencies for different locales |
The java.util.Date
Class Top
The java.util.Date
Class represents a specific instant in time that gives us millisecond precision. The Date
class is intended to reflect an internationally standardized Computer Date and
Time which starts from 1 January 1970 00:00:00 UTC
(Universal Time Coordinated). Date
object creation using the long argument is meant to reflect dates in milliseconds strarting from this
date. Looking at the official documentation for the java.util.Date
Class we can see there is one other non-deprecated Date
constructor that creates a Date
object that represents a
date and time when the the object was allocated to the nearest millisecond.
A lot of the methods in the Date
class were deprecated from the language in the JDK 1.1
release as the Date
class didn't do a very good job of handling internationalisation
and localisation. The java.util.Calendar
was introduced into the language in the JDK 1.1
release to replace the Date
class for easier date manipulation,
internationalisation and localisation and we will look at this class in the next part of this lesson.
The Date
object still serves some purpose though:
- It's an easy way to get the current date and time if you don't want to do a lot of manipulation, localise or internationalise the date and time.
- Good for getting a universal time not affected by time zones.
- Can be useful for simple date comparisons.
- The
DateFormat
class needs aDate
object for some of its formatting methods. So aDate
object can act a bridge between date manipulation in theCalendar
class and formatting of the manipulated date in theDateFormat
class.
The table below shows the non-deprecated constructors, manipulation and comparison methods in the java.util.Date
class:
Constructor/ Method Declaration | Description |
---|---|
Constructors | |
public date() | Allocates and initialize a Date object that represents the time at which it was allocated, to millisecond precision. |
public Date(long date) | Allocates and initialize a Date object that represents the specified number of milliseconds since 1 January 1970 00:00:00 UTC . |
Date Maipulation Methods | |
public long getTime() | Returns number of milliseconds since 1 January 1970 00:00:00 UTC represented by this Date object. |
public void setTime(long time) | Set this Date object to represent a point in time since 1 January 1970 00:00:00 UTC . |
Comparison Methods | |
public boolean after(Date date) | Returns true if invoking Date object is later chronlogically that that represented by the argument Date object named date or false otherwise. |
public boolean before(Date date) | Returns true if invoking Date object is earlier chronlogically that that represented by the argument Date object named date . |
public equals(Object object) | Returns true if invoking Date object is equal to argument Date object or false otherwise. |
public compareTo(Date date) | Returns a negative integer, 0 or a positive integer if invoking Date object less than, equal to or greater than argument Date object respectively. |
Date Example Top
Time to look at an example of using the java.io.Date
class:
package info.java8;
/*
Create some dates and compare and manipulate them
*/
import java.util.Date; // Import the Date class from java.util package
class TestDate {
public static void main(String[] args) {
Date date1 = new Date();
Date date2 = new Date(99999999999L);
Date date3 = new Date(999999999999L);
System.out.println("Our first date is: " + date1);
System.out.println("Our second date is: " + date2);
System.out.println("Our third date is: " + date3);
if (date1.after(date2)) {
System.out.println("Our first date is after our second date.");
}
if (date2.before(date3)) {
System.out.println("Our second date is before our third date.");
}
date2.setTime(date2.getTime() + 999999999999L);
System.out.println("Our second date has been changed to: " + date2);
if (date2.before(date3)) {
System.out.println("Our second date is before our third date.");
}
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
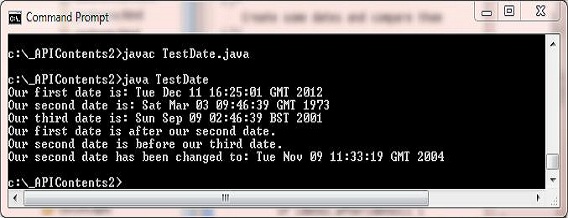
The above screenshot shows the output of compiling and running the TestDate
class in the UK locale (default for me). We create compare and manipulate some dates and print some messages to the console.
java.util.Calendar
Class Top
As you saw above we can manipulate Date
objects using the getTime()
and setTime()
methods, but this is a cumbersome way to manipualte dates. The java.util.Calendar
class
is an easier way to manipulate dates and offers a lot of methods to do it. On inspection of the official documentation for the Calendar
class we can see it is abstract and so cannot be instantiated
directly. To get instances of the Calendar
class we have to use one of the overloaded getInstance
static factory methods.
We will just look at a few of the methods and fields used by the Calendar
class in this lesson. The main aim of the example is to show the versatility of the class and how we can convert from Date
objects to Calendar
objects and vice versa.
The table below shows the methods used in our example but there are many more:
Method Declaration | Description |
---|---|
Get Instance Method | |
public static Calendar getInstance() | Gets a Calendar instance using default time zone and locale. |
Date Maipulation Methods | |
public abstract void add(int field, int amount) | Adds or subtracts amount of time to specified calendar field , based on the calendar's rules |
public void roll(int field, int amount) | Adds specified (signed) amount of time to specified calendar field but doesn't increment larger parts of a date than the part being modified. |
Date Conversion Method | |
public final Date getTime() | Return Date object representing this Calendar time value. |
public final void setTime(Date date) | Sets this Calendar time value from the given Date object. |
Calendar Example Top
Time to look at an example of using the java.io.Calendar
class and a few of its methods:
package info.java8;
/*
Create some dates and convert to calendar and back
*/
import java.util.Date; // Import the Date class from java.util package
import java.util.Calendar; // Import the Calendar class from java.util package
import static java.util.Calendar.*; // Static import of constants
class TestCalendar {
public static void main(String[] args) {
Date date1 = new Date(99999999999L);
System.out.println("Our date is: " + date1);
// Get Calendar instances from static factory method
Calendar cal1 = Calendar.getInstance();
Calendar cal2 = Calendar.getInstance();
cal1.setTime(date1); // Set calendar from date
System.out.println("Our cal1 month is: " + cal1.get(Calendar.MONTH));
System.out.println("Our cal1 year is: " + cal1.get(Calendar.YEAR));
System.out.println("Our cal2 month is: " + cal2.get(Calendar.MONTH));
System.out.println("Our cal2 year is: " + cal2.get(Calendar.YEAR));
cal1.add(MONTH, 21); // No need to qualify due to static imports
date1 = cal1.getTime(); // Set date from calendar
System.out.println("Our date is: " + date1);
cal2.roll(Calendar.MONTH, -21);
date1 = cal2.getTime();
System.out.println("Our date is: " + date1);
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
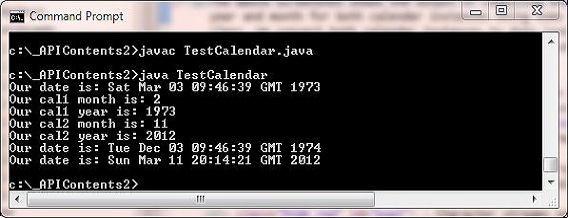
The above screenshot shows the output of compiling and running the TestCalendar
class in the UK locale. We use a static import to get all Calendar
class constants, but we still qualify most of
those used so you can see the long form. We create a date instance and convert it to a calendar instance. We also get another calendar instance and print off the year and month for both calendar instances using some
constants from the Calendar
class. We then use the add()
and roll()
date manipulation methods from the Calendar
class. We convert both calendar instances to date
isntances and printr off the results off the manipulations. The roll()
method doesn't increment larger parts of a date than the part being modified, when doing manipulation like the add()
method does. This is the reason why the year doesnt change when we roll the month back by 25 months. For full details of this and the rest of the methods in the Calendar
class look at the official documentation.
java.util.Locale
Class Top
The Locale
class allows us to create an object that represents a specific geographical, political, or cultural region of the world. We can then use the Locale
object in conjunction with the
DateFormat
or NumberFormat
classes to get locale specific dates, times, numbers and currencies for that locale.
Operations that require specific locale information to perform tasks are known as locale-sensitive and as such use a Locale
object to tailor user information accordingly. As examples,
this could include the way dates are displayed, the way numbers are formatted or the way currencies are viewed.
So once we set a specific locale we can use this object in conjunction with the DateFormat
or NumberFormat
classes to format locale-sensitive data for our users. In this way
we can handle internationalisation and localisation when we want it, or do nothing and automatically use a default locale otherwise.
The table below shows the methods used in our example but there are many more:
Method Declaration | Description |
---|---|
Class Method | |
public static Locale getAvailableLocales() | Returns array of all installed locales. |
Instance Methods | |
public final String getDisplayCountry() | Returns name for country locale that is appropriate for display to the user. |
public final String getDisplayLanguage() | Returns name for language locale that is appropriate for display to the user. |
Locale Example Top
Time to look at an example of using the java.io.Locale
class and a few of its methods:
package info.java8;
/*
Get some locales and display them
*/
import java.util.Locale; // Import Locale Date class from java.util package
class TestLocale {
public static void main(String[] args) {
Locale[] allLocales = Locale.getAvailableLocales();
int i = 0;
for(Locale locale : allLocales) {
i++;
System.out.println("Country: " + locale.getDisplayCountry());
System.out.println("Language: " + locale.getDisplayLanguage());
if (i > 3) break;
}
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
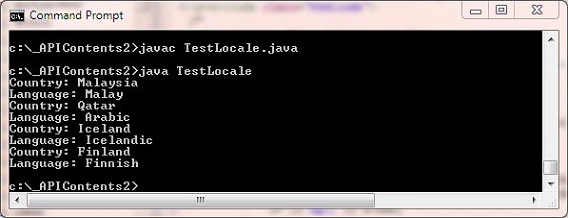
The above screenshot shows the output of compiling and running the TestLocale
class in the UK locale (default for me). We just print off some values from available locales. The real power of locales is when
you use them with dates, number and currencies which we will look at next.
java.util.DateFormat
Class Top
The DateFormat
class allows us to, not suprisingly to get formatted dates and times as well as parsing dates and times in a language-independent manner. On inspection of the official documentation for the
DateFormat
class we can see it is abstract and so cannot be instantiated directly. To get instances of the DateFormat
class we have to use one of the overloaded static factory
methods available and we can also pass options to the constructor to return dates and times with different levels of detail.
The table below just shows the formats we can pass to the static factory methods of the DateFormat
class and the methods from the class used in our example:
Method Declaration | Description |
---|---|
Date Format Style | |
SHORT | Completely numeric representation for a date or time. |
MEDIUM | A longer representation than SHORT . |
LONG | A longer representation than MEDIUM . |
FULL | Longest representation for a date or time. |
Get Instance Method | |
public static final DateFormat getDateInstance(int style) | Get date format with specified format style, default locale. |
public static final DateFormat getTimeInstance(int style) | Get time format with specified format style, default locale. |
DateFormat Example Top
Time to look at an example of using the java.io.DateFormat
class and a few of its methods:
package info.java8;
/*
Create a date and use formatter to display it
*/
import java.util.Date; // Import the Date class from java.util package
import java.text.DateFormat; // Import the DateFormat class from java.text package
import static java.text.DateFormat.*; // Static import of DateFormat constants
class TestDateFormat {
public static void main(String[] args) {
Date now = new Date();
System.out.println("Date is: " + now);
DateFormat dfm = null;
int[] styles = {SHORT, MEDIUM, LONG, FULL};
String[] stylesDesc = {"SHORT", "MEDIUM", "LONG", "FULL"};
// Output current date in various forms for default locale
for(int i=0; i<styles.length; i++) {
dfm = DateFormat.getDateInstance(styles[i]);
System.out.println("style Desc: " + stylesDesc[i] + " is " + dfm.format(now));
dfm = DateFormat.getTimeInstance(styles[i]);
System.out.println("style Desc: " + stylesDesc[i] + " is " + dfm.format(now));
}
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
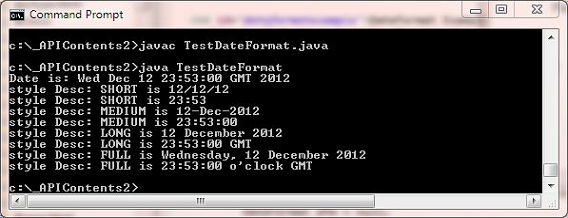
The above screenshot shows the output of compiling and running the TestDateFormat
class in the UK locale (default for me). We just print off different styles for date and time for the current instant in time.
java.util.NumberFormat
Class Top
The NumberFormat
class allows us to get formatted number as well as parsing numbers for any locale. On inspection of the official documentation for the NumberFormat
class we can see it is
abstract and so cannot be instantiated directly. To get instances of the NumberFormat
class we have to use one of the overloaded static factory methods available and we can also pass
options to the constructor to return formatted numbers or currencies.
The table below just shows the formats we can pass to the static factory methods of the NumberFormat
class and the methods from the class used in our example:
Method Declaration | Description |
---|---|
Get Instance Method | |
public static final NumberFormat getInstance() | Get general purpose number format, default locale. |
public static final NumberFormat getCurrencyInstance() | Get currency format, default locale. |
Other Methods | |
public void setMaximumIntegerDigits(int newValue) | Sets maximum number of digits allowed in the integer portion of a number. |
public void setMaximumFractionDigits(int newValue) | Sets maximum number of digits allowed in the fraction portion of a number. |
NumberFormat Example Top
Time to look at an example of using the java.io.NumberFormat
class and a few of its methods:
package info.java8;
/*
Create a number and use formatter to display it
*/
import java.text.NumberFormat; // Import the NumberFormat class from java.text package
import static java.text.NumberFormat.*; // Static import of NumberFormat constants
class TestNumberFormat {
public static void main(String[] args) {
double d = 5678.5678;
System.out.println("double is: " + d);
NumberFormat nfm = null;
// Output number in various forms for default locale
nfm = NumberFormat.getInstance();
System.out.println("Formatted as number: " + nfm.format(d));
// Set integer and fraction digits and reprint
nfm.setMaximumIntegerDigits(2);
nfm.setMaximumFractionDigits(2);
System.out.println("Formatted as number with max 2 digits for int/frac: " + nfm.format(d));
nfm = NumberFormat.getCurrencyInstance();
System.out.println("Formatted as currency: " + nfm.format(d));
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
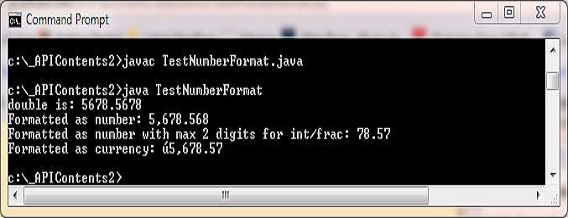
The above screenshot shows the output of compiling and running the TestNumberFormat
class in the UK locale (default for me). We just print off number and then again with 2 digits for integer and fraction and finally currency.
Locale-sensitive Dates & Numbers Top
So far in this lesson we have looked at the Date
and Calendar
classes we can use to get and manipulate and dates and times. We then took a brief look at how we can use different locales and how
the Locale
class holds language and country information for internationalisation. After this we investigated formatting of dates using the DateFormal class
and how we can pass different styles
to the static factory methods to egt different sorts of descriptions about dates and times. We finally looked at the NumberFormat
class to see how we can format numbers and currencies.
In this final part of the lesson we look at using all these different classes together and see how we can use different locales with our dates and formatting classes to truly internationalise our output data.
Locale-sensitive Example Top
Time to look at an example of using different locales on dates and numbers:
package info.java8;
/*
Create locale specific dates and numbers
*/
import java.util.Date; // Import the Date class from java.util package
import java.util.Locale; // Import the Locale class from java.util package
import static java.util.Locale.*; // Static import of Locale constants
import java.text.DateFormat; // Import the DateFormat class from java.text package
import static java.text.DateFormat.*; // Static import of DateFormat constants
import java.text.NumberFormat; // Import the NumberFormat class from java.text package
import static java.text.NumberFormat.*; // Static import of NumberFormat constants
class TestDifferentLocales {
public static void main(String[] args) {
Date now = new Date();
double d = 5678.5678;
System.out.println("Date is: " + now);
DateFormat dfm = null;
NumberFormat nfm = null;
Locale[] locales = {UK, FRANCE, GERMANY, ITALY, US};
int[] styles = {SHORT, MEDIUM, LONG, FULL};
String[] stylesDesc = {"SHORT", "MEDIUM", "LONG", "FULL"};
// Output current date and a number in various forms for different locale
for(Locale locale : locales) {
System.out.println("Formatted displays for country: " + locale.getDisplayCountry());
nfm = NumberFormat.getInstance(locale);
System.out.print("Formatted number: " + nfm.format(d));
nfm = NumberFormat.getCurrencyInstance(locale);
System.out.println(". Formatted currency: " + nfm.format(d));
// Output different styles for this locale
for(int i=0; i<styles.length; i++) {
dfm = DateFormat.getDateInstance(styles[i], locale);
System.out.print("Style Desc: " + stylesDesc[i] + ": Date is: " + dfm.format(now));
dfm = DateFormat.getTimeInstance(styles[i], locale);
System.out.println(". Time is: " + dfm.format(now));
}
}
}
}
Save, compile and run the file in directory c:\_APIContents2 in the usual way.
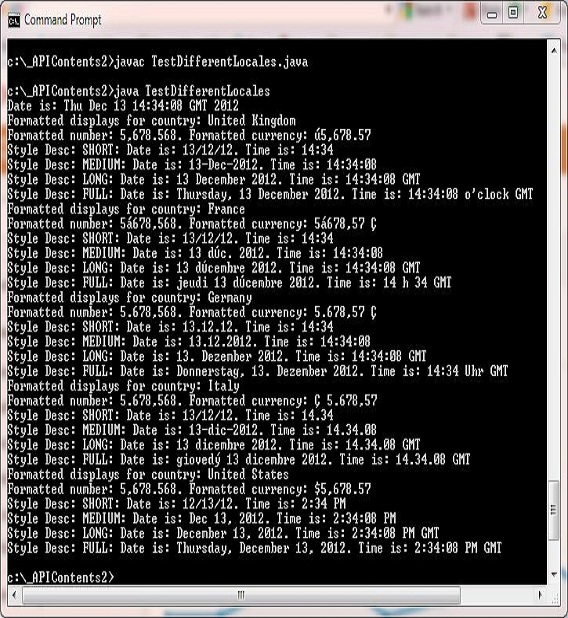
The above screenshot shows the output of compiling and running the TestDifferentLocales
class in the UK locale (default for me). We print off formatted dates and numbers for different locales and styles.
Lesson 4 Complete
In this lesson we looked at the Date
, Calendar
, Locale
, DateFormat
and NumberFormat
classes that allow us to create and manipulate dates,
times, numbers and currencies for different regions of the world.
What's Next?
In the next lesson we look at regular expressions and how we can use regular expression patterns for matching data.